Question
This question should be answered with JavaFX. Here is the Question: To sum it up, I need to know how to code the simplest form
This question should be answered with JavaFX. Here is the Question:
To sum it up, I need to know how to code the simplest form of the game Breakout using javafx. It should be a very basic program (no engine class, no background music, nothing fancy). I have created a game board with a paddle and a ball that moves properly. I just need help adding the bricks and making them disappear when they are hit. Here is what I have so far:
// Using a Timeline for animation, we move a bouncing ball around the Pane
import javafx.application.Application;
import javafx.stage.*;
import javafx.event.*;
import javafx.animation.*;
import javafx.event.*;
import javafx.scene.*;
import javafx.scene.shape.*;
import javafx.scene.layout.*;
import javafx.scene.paint.*;
import java.util.*; // for Random
import javafx.util.*;
import javafx.scene.input.*; // added from last exercise to include KeyCodes
public class Breakout extends Application
{
private double bx, by, bdx, bdy; // x,y coordinate of ball and its velocity
private double px, pdx; // paddle's x coordinate and x velocity
private Circle c; // the ball
private Rectangle r;
private Rectangle b; //the bricks
private Pane pane; // the pane to draw on
public static void main(String[] args)
{
launch();
}
@Override
public void start(Stage primaryStage)
{
Random g = new Random();
bx = Math.abs(g.nextInt())%450 + 25; // start off at random x,y coordinate
by = Math.abs(g.nextInt())%450 + 25;
do { // start off with random velocity between [-3..3,-3..3]
bdx = Math.abs(g.nextInt())%7 - 3;
bdy = Math.abs(g.nextInt())%7 - 3;
}while(bdx==0||bdy==0); // but neither should be 0
px = 220; // start the paddle in the middle with no velocity
pdx = 0;
c = new Circle(bx,by,20); // create the ball
r = new Rectangle(px,450,60,8);
pane = new Pane();
pane.getChildren().add(c); // add initial ball to pane
pane.getChildren().add(r); // add paddle to pane
Scene scene = new Scene(pane, 500,500);
Timeline timer = new Timeline(new KeyFrame(Duration.millis(20),e -> {
pane.getChildren().remove(c); // set up our Timeline, for
pane.getChildren().remove(r); // every action, remove current ball and paddle
if(bx>450) bdx*=-1;
else if(bx
if(by>450) bdy*=-1;
else if(by
bx+=bdx; // move position of ball
by+=bdy;
c = new Circle(bx,by,20); // redraw the ball
pane.getChildren().add(c);
if(px
else if(px>390) {px = 390; pdx = 0;} // note: reset px in case its 390
px+=pdx; // using 390 because px is the left end of the paddle, not the middle
r = new Rectangle(px,450,60,8);
pane.getChildren().add(r);
}));
timer.setCycleCount(Timeline.INDEFINITE);
timer.play(); // Start animation
scene.setOnKeyPressed(e -> { // register the event handler to the scene
if(e.getCode()==KeyCode.LEFT&&pdx>-3) pdx--; // change paddle's velocity based on
else if(e.getCode()==KeyCode.RIGHT&&pdx
else if(e.getCode()==KeyCode.DOWN) pdx=0; // unless already at max velocity
});
primaryStage.setTitle("Breakout");
primaryStage.setScene(scene);
primaryStage.show();
}
public void brick() {
int bNum = 0;
for(int rows = 0; rows
if(bNum
b = new Rectangle(px, 0, 50, 20);
pane.getChildren().add(b);
bNum++;
}
}
}
}
And this is the output that my code gives:
I have posted this question so many times and nobody has helped me. Hopefully somebody can this time. It is a very simple program that I need. Thanks!
For this assignment, you will create the game Breakout. If you do not know Breakout, it is a game with a bouncing ball, paddle and a series of bricks. The object is to hit the ball with the paddle so that it bounces to the other end of the game field and hits one or more bricks. As each brick is hit, it disappears and the user scores points. If the user can clear the entire field of breaks, then the game resets with a new field of bricks. You may have already implemented a portion of this if you have done chapter 15 exercises 5 & 6. If you haven't look at my solution to see my code. You should start with your own code though as you will want to implement this in your own way. How the game works: 1. Have bx, by, bdx and bdy variables to represent the ball's location and velocity. The ball s a Circle 2. Have px and pdx to represent the paddle's upper-left corner and its motion in the x direction. The paddle does not move in the y direction. The paddle is a Rectangle inner class or a class in a separate file, your choice) event handlers 3. Have any array of Bricks. Bricks are defined below as a separate class (either a nested 4. Declare the following as instance data as they will be shared among various methods and a. Circle ball -the ball (needed to add to and remove from the Pane as it moves) b. Rectangle paddle the paddle (add and remove from Pane as it moves) c. int score, lives -player's current score and lives remaining d. Text description - output of the current score and number of lives remaining e. bx, by, bdx, bdy, px, pdx as noted above (used in several locations) f. the array of Bricks (this is a 2-D array) g. the Pane and the Timeline object h. a Random generator S. Iour main method Will call launch 6. Your start method will instantiate or initialize all of your instance data a. for the ball, set bx to the middle horizontally and by to a value beneath the Bricks but well above the paddle, give px a value in the middle horizontally, pdx should start at 0, bdx and bdy should be given random values as long as bdy is positive (so that initially, the ball is moving downward), limit bdx, bdy, pdx to a reasonable range (say -3 to +3) b. create the Text, Circle and Rectangle objects and add them to the Pane; also draw four Lines to have borders around the playing field instantiate all of the Brick objects and for each Brick object, pass it the Pane object; Brick will contact a draw method that will draw a Brick onto the Pane (described below) c. d. create a Timeline object with its own event handler (described in 7) and attach to the Scene a Key EventHandler (described in 8), use a reasonable duration (mine was 15, if you have a larger number, you will want to allow larger ranges for bdx/bdy and pdx) For this assignment, you will create the game Breakout. If you do not know Breakout, it is a game with a bouncing ball, paddle and a series of bricks. The object is to hit the ball with the paddle so that it bounces to the other end of the game field and hits one or more bricks. As each brick is hit, it disappears and the user scores points. If the user can clear the entire field of breaks, then the game resets with a new field of bricks. You may have already implemented a portion of this if you have done chapter 15 exercises 5 & 6. If you haven't look at my solution to see my code. You should start with your own code though as you will want to implement this in your own way. How the game works: 1. Have bx, by, bdx and bdy variables to represent the ball's location and velocity. The ball s a Circle 2. Have px and pdx to represent the paddle's upper-left corner and its motion in the x direction. The paddle does not move in the y direction. The paddle is a Rectangle inner class or a class in a separate file, your choice) event handlers 3. Have any array of Bricks. Bricks are defined below as a separate class (either a nested 4. Declare the following as instance data as they will be shared among various methods and a. Circle ball -the ball (needed to add to and remove from the Pane as it moves) b. Rectangle paddle the paddle (add and remove from Pane as it moves) c. int score, lives -player's current score and lives remaining d. Text description - output of the current score and number of lives remaining e. bx, by, bdx, bdy, px, pdx as noted above (used in several locations) f. the array of Bricks (this is a 2-D array) g. the Pane and the Timeline object h. a Random generator S. Iour main method Will call launch 6. Your start method will instantiate or initialize all of your instance data a. for the ball, set bx to the middle horizontally and by to a value beneath the Bricks but well above the paddle, give px a value in the middle horizontally, pdx should start at 0, bdx and bdy should be given random values as long as bdy is positive (so that initially, the ball is moving downward), limit bdx, bdy, pdx to a reasonable range (say -3 to +3) b. create the Text, Circle and Rectangle objects and add them to the Pane; also draw four Lines to have borders around the playing field instantiate all of the Brick objects and for each Brick object, pass it the Pane object; Brick will contact a draw method that will draw a Brick onto the Pane (described below) c. d. create a Timeline object with its own event handler (described in 7) and attach to the Scene a Key EventHandler (described in 8), use a reasonable duration (mine was 15, if you have a larger number, you will want to allow larger ranges for bdx/bdy and pdx)Step by Step Solution
There are 3 Steps involved in it
Step: 1
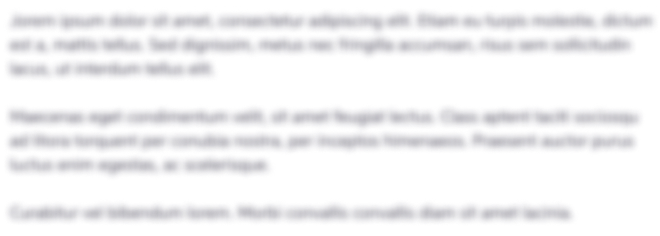
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started