Question
This question should be quick but please go in-depth when explaining about bit operators and how to unpack when given an unsigned int as well.
This question should be quick but please go in-depth when explaining about bit operators and how to unpack when given an unsigned int as well.
Part 5: Packing Up The Bytes!
We learned that a character is represented by 8 bits, also known as a byte. We also learned that integers have potentially different sizes on different machines. We are programming in a 32-bit Linux/x86 environment, so the integers on this machine are represented in 32 bits or 4 bytes. Consider the start of the following C program:
void a_function_name() { unsigned char b3 = 202; unsigned char b2 = 254; unsigned char b1 = 186; unsigned char b0 = 190; }
Note that we are using an unsigned representation of a character. An unsigned character can represent the values 0-255. Your job is to use bitwise operators to copy the bytes b3-b0 into a corresponding unsigned integer called u. Your unsigned integer should place the bytes in the following spot in the integer u:
[ b3 ][ b2 ][ b1 ][ b0 ] 31 24 16 8 0
The decimal values 31, 24, 16, 8, and 0 indicate the starting bit of each byte in the integer. Note that we begin on the right and count bits toward the left. This is known as little-endian representation and corresponds to the bit positions used by the powers of 2 to translate a number represented in bits to its decimal equivalent. In particular, little-endian means that the low-order byte of a value is stored at the lowest byte in memory. In this case, the memory is an integer represented as 4 bytes starting with the first byte (b0), starting at bit 0, and ending with the last byte (b3), starting a bit 24.
Extend the above C program in the function packing_bytes (read: adding the code from that function above). This new program must assign the bits in each of the unsigned characters b3-b0 into their corresponding place in the unsigned integer u. You should use only the bitwise operators for left shift (<<) and or (|). Print the resulting value stored in u as a hexidecimal value using printf (you can use the %X formatting option - see the man page for details). If you do this correctly your program should output the following after it is compiled and executed
$ ./bits_and_bytes_app # Output from previous parts ????????
Where each of the ? characters corresponds to a hexadecimal digit. Your output must be exactly 8 hexadecimal digits and must be exact. Note that the code to do this is rather short.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
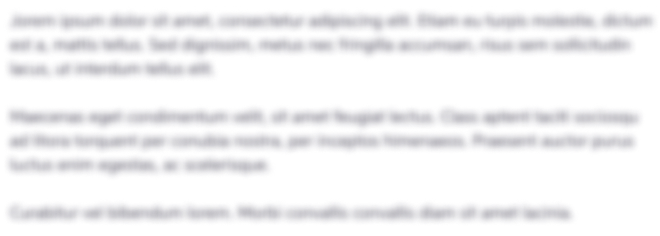
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started