Question
This question was posted before but not answered, this is for C++ .. Lab App 1.1 Bisection Algorithm Exercise 1 Read the Bisection Algorithm handout
This question was posted before but not answered, this is for C++ .. Lab App 1.1 Bisection Algorithm Exercise 1 Read the Bisection Algorithm handout (bisection.pdf) and write a function for the algorithm that returns the answer to the calculation. The main function is responsible for reading the input parameters and writing the output. Make sure to check input for errors before calling the Bisection algorithm. Use the algorithm with the given function in the example, which you will have to code into the program as a C++ function. Follow the example given. Lab App 1.2 (Extra Credit) Gaussian Elimination with Backward Substitution Exercise 1 Use the provided Gaussian Elimination Algorithm (gaussian.cpp) and write a program to compute the inverse of a matrix. If A is a matrix of size N N (a 2-D array) and Id is the identity matrix (one where all the entries are zero except for the diagonals, which are one), the inverse M of a matrix A is defined by A M = Id M can be though of as a vector of vectors X. That is M = {X1, X2, , XN}, where Xk is a vector of size N, etc. and similarly, Id = {Id1, Id2, , IdN}, where Idk are vectors in which all elements are zero except for the kth element, which is 1. For example, Id2 = {0,1,0,0,,0}. Then, given A, use the gaussian algorithm to solve A Xk = Idk for each Xk , which are the columns of the inverse matrix M. This algorithm should be a function that takes A and N as input and returns M as output. The main function should ask the user for N and the entries of the matrix A and writes the elements of M to the screen.
1. Gaussian Elimination with Backward Substitution To solve the n x n linear system E1j: a11 x1 + a12 x2 + + a1n xn = b1 E2: a21 x1 + a11 x2 + + a2n xn = b2 .. En: an1 x1 + an2 x2 + + ann xn = bn INPUT number of unknowns and equations n; augmented matrix A = (aij), that is, where ai,n+1 = bi and where 1 <= i <= n and 1 j n + 1 OUTPUT solution x1, x2, , xn or message that linear system has no unique solution Step 1 For i = 1, , n-1 do Step 2-4. (Elimination Process.) Step 2 Search for the first integer p, starting at i and up to n so that ap,i 0 If no integer p is be found then OUTPUT (no unique solution exists) STOP. Step 3 If p > i then swap the equations for p and i (Ep) <-> (Ei ). Do this by creating and calling a function that takes two arrays, and swap each of the elements of the arrays. Step 4 For j = i + 1 , , n do Steps 5 and 6 Step 5 Set m = aji/aii Step 6 Perform (Ej ) = (Ej - mji Ei ) (that is, ajk = ajk - m aik for k = i up to n+1) Step 7 If ann = 0 then OUTPUT (no unique solution exists) RETURN failure flag Step 8 Set xn = an,n+1 / ann. (Start backward substitution.) Step 9 For i = n-1, , 1 set xi = [ai,n+1 - ( from j=i+1 to n) aij xj ] / aii Step 10 RETURN success flag Step 11 If gaussian function successful, OUTPUT (xi , , xn ) values from main function (see sample run) SAMPLE RUN FOR: 3 x2 - 6 x3 = -12 2 x1 - 7 x2 + 4 x3 = 0 -4 x1 + x2 + 3 x3 = 7 Enter number of linear equations (up to 100): 3 Input values of A and B for the equation AX=B Enter the coefficients for Eq.[1] A[1][1]: 0 A[1][2]: 3 A[1][3]: -6 B[1]: -12 Enter the coefficients for Eq.[2] A[2][1]: 2 A[2][2]: -7 A[2][3]: 4 B[2]: 0 Enter the coefficients for Eq.[3] A[3][1]: -4 A[3][2]: 1 A[3][3]: 3 B[3]: 7 Solution: X[1] = 1 X[2] = 2 X[3] = 3
1. The Bisection Algorithm (write this as a function, with f(x) as a separate function) To find a solution to f(x) = 0 given the continuous function f on the interval [a,b] where f(a) and f(b) have opposite signs: INPUT endpoints a,b; tolerance tol; maximum number of iterations N0. OUTPUT approximate solution p or message of failure. Step 1Set i = 1 Step 2 While i < N0 do Steps 36. Step 3 Set p = (a + b) /2 . // (ie, compute pi ) Step 3. OUTPUT: i a b p f(p) Step 4 If | f(p) | < 1.E-19 or (b - a ) /2 < tol then (note that | f(p) | is the absolute value of f(p) ) RETURN p; // (Procedure completed successfully.) Step 5 If f(a)f(p) > 0 then set a = p (compute ai, bi ) else set b = p Step 6 Set i = i + 1 Step 7 OUTPUT: (Method failed after N0 interactions, N0 = , N0 ); (procedure completed unsuccessfully.) STOP PROGRAM EXAMPLE RUN for f(x) = 20*x^2 - 7*x - 40 (exact solutions: 1.6 and -1.25) Enter endpoints a and b surrounding solution: 1 2 Enter tolerance: 1E-5 Enter maximum number of iterations: 100 i a b p fp 1 1.00000 2.00000 1.50000 -5.50000 2 1.50000 2.00000 1.75000 9.00000 3 1.50000 1.75000 1.62500 1.43750 4 1.50000 1.62500 1.56250 -2.10938 5 1.56250 1.62500 1.59375 -0.35547 6 1.59375 1.62500 1.60938 0.53613 7 1.59375 1.60938 1.60156 0.08911 8 1.59375 1.60156 1.59766 -0.13348 9 1.59766 1.60156 1.59961 -0.02226 10 1.59961 1.60156 1.60059 0.03341 11 1.59961 1.60059 1.60010 0.00557 12 1.59961 1.60010 1.59985 -0.00835 13 1.59985 1.60010 1.59998 -0.00139 14 1.59998 1.60010 1.60004 0.00209 15 1.59998 1.60004 1.60001 0.00035 16 1.59998 1.60001 1.59999 -0.00052 17 1.59999 1.60001 1.60000 -0.00009 A zero was found at p = 1.60000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
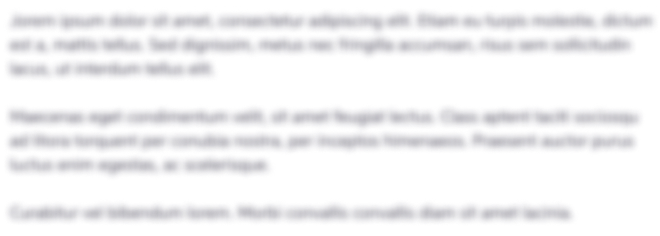
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started