Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This task requires you to implement a C + + class that has some of the functions of a dynamic array or vector. to complete
This task requires you to implement a C class that has some of the functions of a dynamic array or
vector.
to complete each of the levels of this task.
The class you will develop is called CPWordVector. We have provided the header file
CPWordVector.h as well as a client program main.cpp that makes use of the class. For level
you will complete CPWordVectors implementation in a separate file CPWordVector.cpp
and for level and you will add functions to main.cpp that make use of the class.
In this task, we will make use of the CPWordVector to store information about words and the
number of times that they occur in the input to our main program. The main.cpp program reads
words from standard input, and stores all the words into a CPWordVector one by one as it reads
them. When input is finished, main.cpp iterates over the vector and prints out all the stored words
and the number of words that have been stored the size of the vector The code to print out the
vector contents has already been provided; you can view this inside the main function of
main.cpp You will probably find it easier to develop your program if you redirect input so it comes from a text
file as we have done for other tasks this semester In CLion, you do this by RunEdit
ConfigurationsRedirect Input From. On the command line, you can use the symbol to redirect
from your text file.
The main.cpp program checks the command line arguments in order to choose which of the three
levels to execute. Depending on the command line argument that has been passed in it will call
either the level level or level function. Only level has been implemented
for you already. The argument is a number: or
For level you implement CPWordVector.cpp and then test it by running with argument
which will automatically call the level function. For levels and you leave
CPWordVector.cpp as it is and implement each of level and level in main.cpp
In order to develop and test your program, you simply pass in the argument or to your
program. In CLion, you do this by RunEdit ConfigurationsProgram Arguments. On the command
line, if you have compiled your program for example to an executable called vector.exe, you can
run the program using for example
vector test.txt
to test level where test.txt is the file containing the input. Level : Simple Insertion weight:
All you need to do to complete Level of the Task is to complete the implementation of the
CPWordVector class. You must do this by creating a new file CPWordVector.cpp and
submitting this file in your submission. You do not need to implement the level function in
main.cpp as this has been implemented for you already. Do not modify level as this may
cause the automated test to fail.
Once you have implemented the four methods of CPWordVector, you can run the main
program. If you pass in a command line argument of the main program will call level and
you will see the output. The level function simply adds a new WordInfo for every word it
sees, with a counter value of Even if a word is seen multiple times, this will not be reflected in
the vector the repeated occurrences will simply have a separate entry each time. For example, if
the input contained
mouse apple mouse dog
Then the output would be
mouse:
apple:
mouse:
dog:
Total entries: main.cpp code below: #include
#include CPWordVector.h
using namespace std;
simple
void levelCPWordVector& vector
string word;
while cin word
WordInfo info;
info.word word; info.counter ;
vector.insertvectorend info;
void levelCPWordVector& vector store frequency
add your code for level here
void levelCPWordVector& vector sorted alphabetically
add your code for level here
int mainint argc, char argv
char end;
long which ;
whichstrtolargv &end, ;
CPWordVector vector;
switchwhich
case : levelvector; break;
case : levelvector; break;
case : levelvector; break;
for auto it vector.begin; it vector.end; it
cout itword : itcounter endl;
cout "Total entries: vector.size
;
return ;
CPWordVector.h code below: #ifndef PRACSUPPCPWORDVECTORH
#define PRACSUPPCPWORDVECTORH
#include
class WordInfo
public:
std::string word;
int counter;
;
class CPWordVector
public:
WordInfo begin;
WordInfo end;
int size;
WordInfo insert WordInfo position, const WordInfo& item;
private:
WordInfo items;
int numberitems ;
;
#endif PRACSUPPCPWORDVECTORH
Step by Step Solution
There are 3 Steps involved in it
Step: 1
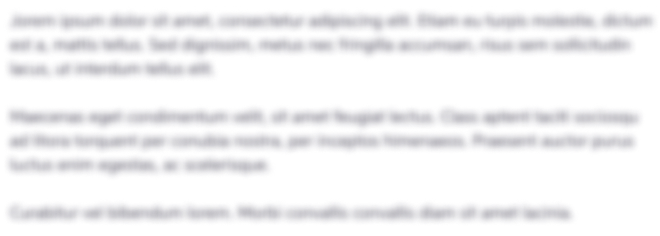
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started