Question
Three files to be submitted: ? Student Design ( A UML diagram) ( no credit if this is missing) ? Java Source code ( Student.java
Three files to be submitted:
? Student Design ( A UML diagram) ( no credit if this is missing)
? Java Source code ( Student.java - no credit if this has syntax errors)
? A driver ( StudentTest.java - no credit if this has syntax errors)
Write a class to model a college student. A student should have a name and two test scores. The following shows the design. Dont add additional instance variables and additional instance methods to make coding more convenient. 5 points will be deducted if this happens.
The following shows the design. You need to complete the design using Violet UML editor.
Student.java | |||
Instance Variables: No additional instance variables are allowed in this class. | |||
name | The name of this student | ||
testOne | The test one score of this student | ||
testTwo | The test two score of this student | ||
Instance methods: No additional instance methods are allowed in this class. | |||
Constructor(s) | Initializes all of the instance variables. It is used to create an instance of this class. A student should be created with a name, 0 for test one, and 0 for test wo. | ||
Getters/setters | |||
getName | Returns this student's name as a string public String getName(){...} | ||
setName | Changes this students name to be a new name provided when this method is invoked. public void setName(String name) {...} | ||
getTestOne | Returns this student's first test score as an int value; public int getTestOne(){...} | ||
setTestOne | Changes this student's first test score to be a new scored provided when this method is invoked. | ||
getTestTwo | Returns this student's second test score as an int value. | ||
setTestTwo | Changes this student's second test score to be a new score provided when this method is invoked. | ||
Others | |||
calculateAverage | Computes this student's average score of testOne and testTwo, and returns it as a double value. | ||
toString and equals | |||
toString | Returns a string representation of this students name, testOne, testTwo. public String toString() | ||
equals | Indicates of this student is equal to some other object. public boolean equals(Object obj) | ||
StudentTest.java This is a driver program where all methods of Student.java must be tested. There are no sample runs. | |||
main | All methods must be used/tested properly. | ||
The following shows how to translate the above design into Java code.
Create a program Student.java to model a college student and test each method in StudentTest.java.
Open TextPad; create two files such as Student.java and StudentTest.java. MyOwnClass.java andMyDriver.java, the two templates, can be used to start.
In StudentTest.java, add an empty main method.
public class StudentTest {
public static void main(String[] args) {
} }
Javadoc comments must be included for instance variables and instance methods. In Student.java, 1. Write instance variables. Instance variables represent values a student is created with. Each student is
created with three values: name, testOne and testTwo. Add three instance variables.
public class Student {
}
/** * The name of this student */
private String name;
/** * The test one score of this student */
private int testOne;
/** * The test two score of this student */
private int testTwo;
Compile Student.java first, and then compile StudentTest.java. Fix the compiler errors if any. 2. Write the constructor.
A student should be created with a real name (a string) as shown. Default values 0 should be used for both test one and test two. This is how a constructor is used in a driver program. The name John Doecould also be entered via keyboard.
Student student1 = new Student(John Doe);
In order to use the student constructor above, it must be created in Student.java. A
constructor must initialize each instance variable with a provided value or a default value.
/** * Constructs this student with a name, 0 of test score one, and 0 * of test score two. */
public Student(String name) {
//Initialize this students name //Initialize this students test one with 0 //Initialize this students test two with 0
}
Compile Student.java first, and then compile StudentTest.java. Fix the compiler errors if any.
Write another method a getter for name. Dot notation objectReference.method(?) is always used to invoke an instance method. If student student1 is created, student1s name can be retrieved as follows:
objectReference.method(?)
student1.getName();
The returned name can be stored as follows:
String n; n = student1.getName();
In order to use this getter in StudentTest.java , it must be created in Student.java./**
* Returns the name of this student.
*/
public String getName() {
//Returns the name of this student
}
Compile Student.java first, and then compile StudentTest.java. Fix the compiler errors if any.
Repeat for each additional method. Keep in mind, a setter method changes one instance variables value to
be a new value provided when this method is called. A setter doesnt return any value. Here is an example
of setName.
/** * Changes the name of this student. */
public void setName(String name) {
//Changes the name of this student
}
5. Here are some hints for equals method.
Each student is created with three values, the content. Therefore, students should be compared by name, test one, and test two. If they are all the same, students are equal.
Operator == should be used to compare integers such as test one scores and test two scores. Method equals
or equalsIgnoreCase in String class should be used to compare String objects such as names. The result
should be the combined results of three comparisons.
/** * Indicates if this student is equal to some other object. This student is equal * to some other student if they have same names, same test one scores and same * test two scores.
*/
public boolean equals(Object obj) {
... return name comparison && testOne comparison && testTwo comparison;
}
Javadoc comments must be included for instance variables and instance methods.
The following shows you how much Javadoc comments you should write for each instance variables and each methods in Student.java. You need to complete the rest of the class.
/** * Represents a college student with a name, a score on test one, and * a score on test two. */
public class Student {
/** * The name of this student */
private String name;
/** * The test one of this student */
private int testOne;
/** * The test two of this student */
private int testTwo;
/**
* Constructs a student with given name, 0 for both test scores.
*/
public Student(String name) {
...}
/** * Returns the name of this student. */ public String getName() {
...}
/** * Changes the name of this student to be n. */
public void setName(String name) {
...}
...}
The following shows you how much comments you should write for main methods in StudentTest.java./**
* You have to add comments here.
*/
public class StudentTest {
/** * You have to add comments here. */
public static void main(String[] args) {
//comments are needed here.
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
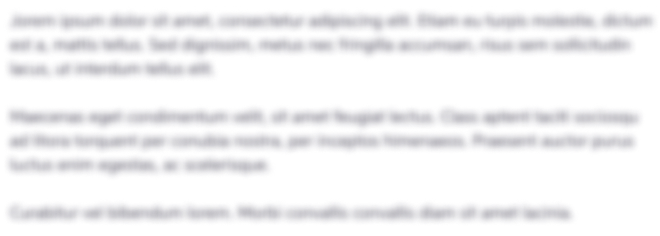
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started