Question
Three-Card High-Card For this assignment, implement the class that is described below along with your main function in a single C++ source file. Your source
Three-Card High-Card
For this assignment, implement the class that is described below along with your main function in a single C++ source file. Your source file should include cards.h (via #include cards.h) and link with the code in cards.cpp. Use the cards library (version 1) that was developed in class.
Create a class called ThreeCardHand that represents a players hand in the game that is described below. Objects of the class should be capable of storing up to three Card objects.
The class ThreeCardHand should be written to support a game called Three-Card High-Card. In this simple game, each player is dealt three cards. The winner of the game is the player who has the highest card (as determined by comparison of Card objects as we defined in class) in their hand. If two players have the same high card, then the second highest card is used to determine the winner. If the two highest cards are the same, then the highest third card determines the winner. If all three cards have the same value, the two hands are tied.
In addition to implementing the ThreeCardHand class, write a program that uses your class to simulate playing the game 10 times between two players. For each simulation of the game, start with a newly shuffled deck of cards (Deck object). Deal three cards to each player, display both hands, and state which hand wins.
Build your ThreeCardHand class with the following public interface:
class ThreeCardHand {
public:
ThreeCardHand ();
void clear (); // removes all cards from the hand
void insert (const Card& c); // inserts a card into the hand
std::string str () const; // returns string representation of all cards in the hand
bool eq (const Card& c); // true if hand object is equal to the given hand object
bool gt (const Card& c); // true if hand object is greater than the given hand object
// other comparison objects, if you choose to implement them
};
Implementation of the class (private members) is up to you. You may include additional public members if you choose, but include the functions shown above at a minimum.
Cards Library (version 1)
-------------------main.cpp--------------------------------
#include "cards.h"
#include
#include
#include
using namespace std;
int main() {
srand(time(0));
Deck d;
//Card c;
//for (int i = 0; i < 52; i++) {
// c = d.deal();
// cout << c.str() << endl;
//}
for (int i = 0; i < 10; i++) {
Card c1 = d.deal();
Card c2 = d.deal();
if (c1.eq(c2))
cout << c1.str() << " equals " << c2.str() << endl;
else if (c1.gt(c2))
cout << c1.str() << " greater than " << c2.str() << endl;
else
cout << c1.str() << " less than " << c2.str() << endl;
}
system("pause");
return 0;
}
-------------------cards.cpp------------------------------
#include "cards.h"
#include
Card::Card() {
suit_index = 4;
value_index = 13;
}
Card::Card(int card_index) {
suit_index = card_index % 4;
value_index = card_index / 4;
}
char Card::suit() const {
static const char suits[] = { 'H','S','D','C','X' };
return suits[suit_index];
}
char Card::value() const {
static const char values[] = { '2','3','4','5','6','7','8',
'9','T','J','Q','K','A','X' };
return values[value_index];
}
std::string Card::str() const {
std::string s;
s += value();
s += suit();
return s;
}
Deck::Deck() {
//for (int i = 0; i < 52; i++)
// cards[i] = Card(i);
for (int i = 0; i < 52; i++) {
Card c(i);
cards[i] = c;
}
//used_count = 0;
shuffle();
}
Card Deck::deal() {
return cards[used_count++];
}
void Deck::shuffle() {
for (int i = 0; i < 52; i++)
std::swap(cards[i], cards[rand() % 52]);
used_count = 0;
}
----------------------cards.h-------------------------------
#pragma once
#include
class Card {
private:
int suit_index, value_index;
public:
Card();
Card(int card_index);
char suit() const;
char value() const;
std::string str() const;
bool eq(const Card& c) const { return value_index == c.value_index; }
bool gt(const Card& c) const { return value_index > c.value_index; }
};
class Deck {
private:
Card cards[52];
int used_count;
public:
Deck();
Card deal();
void shuffle();
int size() const { return 52 - used_count; }
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
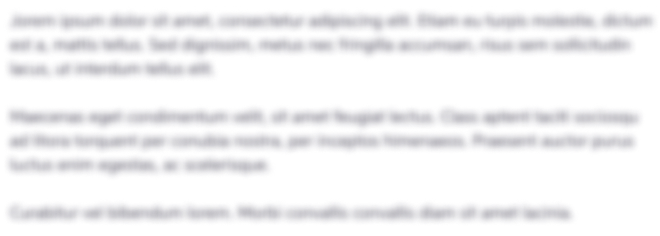
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started