Question
To begin getting ready for CS 261, you will write a C program that fills and sorts a singly linked list of integers. Make sure
To begin getting ready for CS 261, you will write a C program that fills and sorts a singly linked list of integers. Make sure your program compiles using gcc and the following list.h and test_list.c files on the ENGR server. When sorting nodes, you may not swap the values between the nodes, you must change the pointers on the nodes to swap them.
list.h
struct node { int val; struct node *next; }; int length(struct node *); //get the length of the list void print(struct node *, int); //print a certain number of elements from the list starting with the first node struct node * push(struct node *, int); //put at front struct node * append(struct node *, int); //put at back struct node * clear(struct node *); //delete entire list struct node * remove_node(struct node *, int); //delete a particular node struct node * sort_ascending(struct node *); //sort the nodes in ascending order struct node * sort_descending(struct node *); //sort the nodes in descending order //insert into a specific location in the list struct node * insert_middle(struct node *, int val, int idx);
test_list.c
#include "list.h" #include #include int main (){ char ans[2]; int num; struct node *head = NULL; do { do { printf("Enter a number: "); scanf("%d", &num); head = push(head, num);//Can change to append printf("Do you want another num (y or n): "); scanf("%1s",ans); } while(ans[0] == 'y'); printf("Sort ascending or descending (a or d)? "); scanf("%1s",ans); Dec. 4 if(ans[0] == 'a') head=sort_ascending(head); else if(ans[0] == 'd') head=sort_descending(head); print(head, length(head)); printf("Do you want to do this again (y or n)? "); scanf("%1s",ans); head = clear(head); } while(ans[0] == 'y'); return 0; }
For example: Enter a number: 100 Do you want another num (y or n): y Enter a number: 30 Do you want another num (y or n): y Enter a number: 50 Do you want another num (y or n): y Enter a number: 10 Do you want another num (y or n): n Sort ascending or descending (a or d)? a Your linked list is: 10 30 50 100 Do you want to do this again (y or n)? n
Step by Step Solution
There are 3 Steps involved in it
Step: 1
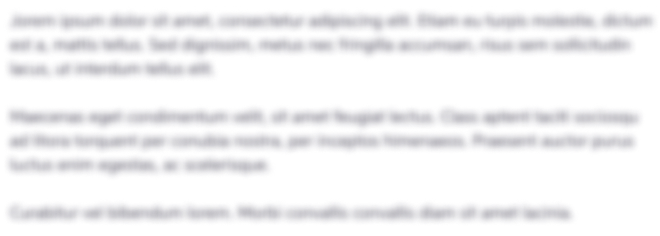
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started