Question
// TO DO: add your implementation and JavaDocs. public class ThreeTenDynArray { //default initial capacity / minimum capacity private static final int MIN_CAPACITY = 2;
// TO DO: add your implementation and JavaDocs.
public class ThreeTenDynArray
// ADD MORE PRIVATE MEMBERS HERE IF NEEDED! @SuppressWarnings("unchecked") public ThreeTenDynArray() { // Constructor // Initial capacity of the storage should be MIN_CAPACITY // Hint: Can't remember how to make an array of generic Ts? It's in the textbook...
}
@SuppressWarnings("unchecked") public ThreeTenDynArray(int initCapacity) { // Constructor
// Initial capacity of the storage should be initCapacity.
// - Throw IllegalArgumentException if initCapacity is smaller than // MIN_CAPACITY 2 // - Use this _exact_ error message for the exception // (quotes are not part of the message): // "Capacity must be at least 2!" }
public int size() { // Report the current number of elements // O(1) return -1; //default return, remove/change as needed } public int capacity() { // Report max number of elements of the current storage // (subject to change since this is a _dynamic_ ) // O(1) return -1; //default return, remove/change as needed }
public T set(int index, T value) { // Replace the item at the given index to be the given value. // Return the old item at that index. // Note: You cannot add new items (i.e. cannot increase size) with this method. // O(1) // - Throw IndexOutOfBoundsException if index is not valid // - Use this code to produce the correct error message for // the exception (do not use a different message): // "Index: " + index + " out of bounds!" // - Throw IllegalArgumentException if value is null. // - Use this _exact_ error message for the exception // (quotes are not part of the message): // "Cannot include null values!"
return null; //default return, remove/change as needed }
public T get(int index) { // Return the item at the given index // O(1) // Use the exception (and error message) described in set() // for invalid indicies. return null; //default return, remove/change as needed }
@SuppressWarnings("unchecked") public void append(T value) { // Append an element to the end of the storage. // Double the capacity if no space available. // Code reuse! Consider using setCapacity (see below). // For a null value, use the same exception and message // as set(). // You can assume we will never need to grow the capacity to a value // beyond Integer.MAX_VALUE/4. No need to check or test that boundary // value when you grow the capacity. // Amortized O(1) // - Throw IllegalArgumentException if value is null. // - Use the same error message as set().
}
@SuppressWarnings("unchecked") public void insert(int index, T value) { // Insert the given value at the given index and shift elements if needed. // You _can_ append items with this method.
// Double capacity if no space available. // Assume the same as append() for the upper bound of capacity. // Code reuse! Consider using setCapacity (see below). // For an invalid index or a null value, use the same exception and message // as set(). However, remember that the condition of the exception is // different (a different invalid range for indexes). // O(N) where N is the number of elements in the storage } @SuppressWarnings("unchecked") public T remove(int index) { // Remove and return the element at the given index. Shift elements // to ensure no gap. Throw an exception when there is an invalid // index (see set(), get(), etc. above). // Halve capacity (rounding down) of the storage if the number of elements falls // below or at 1/4 of the capacity. // However, capacity should NOT go below MIN_CAPACITY. // Code reuse! Consider using setCapacity (see below). // O(N) where N is the number of elements currently in the storage return null; //default return, remove/change as needed
}
@SuppressWarnings("unchecked") public boolean setCapacity(int newCapacity) { // Change the max number of items allowed before next expansion to newCapacity. // No other changes to the current values in storage // (i.e. they should all keep the same index). // Capacity should not be changed if: // - newCapacity is below MIN_CAPACITY; or // - newCapacity is not large enough to accommodate current number of items // Return false if newCapacity cannot be applied; return true otherwise. // Special case: if newCapacity is identical to the current max number of items, // no need to change anything but still return true. // O(N) where N is the number of elements in the array return false; //default return, remove/change as needed } public int firstIndexOf(T value) { // Return the index of the first occurrence of value or -1 if not found. // NOTES: - Remember null is not a valid item in list. // - Remember to use .equals for comparison of non-null values. // O(N) where N is the number of elements in the list return -2; //default return, remove/change as needed }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
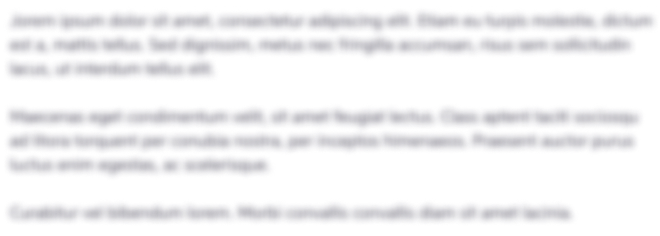
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started