Question
To prevent trivialization of the assignment, only import the following classes. java.util.ArrayList java.util.NoSuchElementException Provided Files Node.java - Each element of the LinkedList should be a
To prevent trivialization of the assignment, only import the following classes.
java.util.ArrayList java.util.NoSuchElementException
Provided Files
Node.java - Each element of the LinkedList should be a Node with a data pointer to the next Node. The only elements in a Node should be T data and Node next. use knowledge of lists and generics.
public class Node {
private T data;
private Node next; public Node(T data, Node next) {
this.data = data;
this.next = next;
}
public T getData() { return this.data;
}
public Node getNext() {
return this.next;
}
public void setData(T data) {
this.data = data;
}
public void setNext(Node next) {
this.next = next;
}
}
LinkedList.java
Variables
Variables must not be directly modified outside the class in which they are declared, unless in the description of the variable. No more instance variables are needed.
Node head- this represents the first node in the LinkedList
int size- this represents the number of nodes in the LinkedList
Constructors
A constructor that takes in nothing that sets theheadto null and thesizeto 0 to instantiate aLinkedList.
Methods
Do not make any other methods than those specified. Any extra methods will result in point deductions.
addAtIndex(T data, int index) oMethod that adds another Node at the specified index in the LinkedListoIf the index is out of bounds (outside of [0, size] inclusive), then throw an
IllegalArgumentExceptionwith an appropriate messageoDoes not return anything.
removeFromIndex(int index) oThis method removes the Node at the index that is specified. oIf the index is out of bounds (outside of [0, size] exclusive), then throw an
IllegalArgumentExceptionwith an appropriate message oReturns the data that was removed. (Note: the data, not the Node)
clear() oThis method will clear out the entire linked list oIf the LinkedList is already clear, throw a NoSuchElementException with an appropriate messageoReturns nothing oHint: You can perform this in 2 lines of code
get(int index) oFind the data at the specified index in the LinkedList oIf the index is out of bounds (outside of [0, size] exclusive), then throw an
IllegalArgumentExceptionwith an appropriate messageoReturns the data that is in the specified index
isEmpty() oThis method will return true or false based on if the LinkedList is currently empty.oReturns a boolean indicating that the LinkedList is empty oHint: You can perform this with one line of code
toArrayList() oConvert the LinkedList to an ArrayList oTraverse through your LinkedList and add all the data contained in each Node to an ArrayListoData should appear in the same exact order and the ArrayList should be the same size oReturns the ArrayList that was made oThe ArrayList should be parameterized so that it is an ArrayList.
fizzBuzzLinkedList() oReturns a new LinkedList that stores elements of type String, following a similar idea to the well-
known FizzBuzz coding problem oThe returned LinkedList will be of the same size as the one this method is called on. Each element
will be mapped into the new LinkedList as follows:
We define position as starting from 1. That is, the head of the linked list is at position 1,
the element next to the head is a position 2, and a LinkedList of size s will have its last
element at position s.
If the element is in a position that is multiple of 3 but not of 5, the element in the new
LinkedList will be"Fizz"
If the element is in a position that is multiple of 5 but not of 3, the element in the new
LinkedList will be"Buzz"
If the element is in a position that is both multiple of 3 and 5, the element in the new
LinkedList will be"FizzBuzz"
Otherwise, the element in the new LinkedList will be the toString of the element in the
original position, prefixed with"[# of position]: [toString]"(number colon space
toString)
oExample:
Original Linked List (showing toString of each element, LinkedList could be of any type):"A"->"B"->"C"->...->"O"->"P"
New LinkedList (always parameterized with type String):"1: A"->"2: B"->"Fizz"->"4: D"->"Buzz"->"Fizz"->"7: G"->"8: H"->"Fizz"->"Buzz"->"11: K"->"Fizz"->"13: M"->"14: N"->"FizzBuzz"->"16: P"
Hint: the head, size, and the next pointers are updated when necessary. If head is not updated when it needs to be, data will be lost and the LinkedList will not be updated correctly!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Solution for the above question is Parameterized Node class code public class Node private T data pr...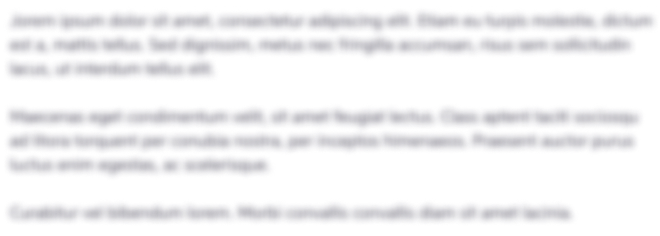
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started