Question
Topics: abstract class, interface, package, serializable, factory design pattern The Problem Suppose you are a software engineer working for a small software consulting company called
Topics: abstract class, interface, package, serializable, factory design pattern
The Problem
Suppose you are a software engineer working for a small software consulting company called J Software. A high school has contracted your company to computerize their tests. Your company would provide software for the client to create tests that students can take on computers. Your project lead has analyzed the requirements and came up with some Java interfaces for type of questions the program must support and has assigned to you to implement the solution. The implementation of the interfaces will also be used to created the two programs that will be used by the school. The first program is for creating questions for tests and the second program is for administering the tests to students.
The Interfaces
The interface code is provided. Below are the interfaces provided for you: Type of questions interfaces o IMultipleChoiceQuestion o ITrueFalseQuestion o IFillInBlanksQuestion o IShortAnswerQuestion o IQuestion Question set and question factory interfaces o IQuestionSet (a collection of questions) o IQuestionFactory (a factory to creating questions)
IMultipleChoiceQuestion:
package com.jsoftware.test.api;
/**
* Interface for multiple choice question. A multiple choice question has
* a question and a list of choices. To check if a user has select the
* correct choice, the choice is convert to an index and is matched to the
* correct index in the question.
*
*/
public interface IMultipleChoiceQuestion extends IQuestion{
/**
* Check answer.
* @param index The index of the choice.
* @return True if answer is correct.
*/
public boolean checkAnswer(int index);
}
ITrueFalseQuestion:
/*
The interface for true false question.
*/
package com.jsoftware.test.api;
/**
*
*/
public interface ITrueFalseQuestion extends IQuestion{
/**
* Check if the answer is correct.
* @param answer The user's answer as as a boolean
* @return true if correct.
*/
public boolean checkAnswer(boolean answer);
}
IFillInBlanksQuestion:
package com.jsoftware.test.api;
/**
* Interface for fill in the blanks questions. A question may contain one or
* more blanks. The answers are a list of words. The answer is correct only if
* all the keywords match provided by the user matches the correct answer keywords.
*
*/
public interface IFillInBlanksQuestion extends IQuestion{
/**
* A fill in the blank question may contain multiple blanks.
* Each blank must match each answer.
* @param keywords An array of answer the user provides.
* @return
*/
public boolean checkAnswer(String[] keywords);
}
IShortAnswerQuestion:
package com.jsoftware.test.api;
public interface IShortAnswerQuestion extends IQuestion{
/**
* Check answer for short answer question.
* @param answer The user's answer as as a boolean
* @return true if correct.
*/
public boolean checkAnswer(String answer);
}
IQuestion:
package com.jsoftware.test.api;
public interface IQuestion {
/**
* Return a formatted question as a string. It should include options if
* the question is a multiple choice or true/false question.
*
* Multiple choice example
* Which of the following is not a primitive type in Java?
* 1) double
* 2) int
* 3) String
* 4) boolean
*
* True false example:
* int is not a primitive data type in Java. True/False?
*/
public String getQuestion();
}
IQuestionSet (a collection of questions):
package com.jsoftware.test.api;
/**
* This interface represents a set of question.
*
*/
public interface IQuestionSet {
/**
* Create an empty test set.
* @return return an instance of a test set.
*/
public IQuestionSet emptyTestSet();
/**
* return a test set consisting of a random questions.
* @param size The number of random questions.
* @return The test set instance containing the random questions.
*/
public IQuestionSet randomSample(int size);
/**
* add a question to the test set.
* @param question The question
* @return True if successful.
*/
public boolean add(IQuestion question);
/**
*
* @param index Remove question using index
* @return true if index is valid
*/
public boolean remove(int index);
/**
* Retrieving a question using an index
* @param index
* @return the question if index is valid, null otherwise.
*/
public IQuestion getQuestion(int index);
/**
* Return the number of questions in this test set.
* @return number of questions.
*/
public int size();
}
IQuestionFactory (a factory to creating questions):
package com.jsoftware.test.api;
import java.io.IOException;
public interface IQuestionFactory {
/**
* creates a multiple choice question.
* @param question The question
* @param choices The choices as an array
* @param answer The index to the answer in the choices array
* @return An instance of a multiple choice question.
*/
public IQuestion makeMultipleChoice(String question, String[] choices, int answer);
/**
* creates a true-false question.
* @param question The question.
* @param answer The answer
* @return an instance of a true-false question
*/
public IQuestion makeTrueFalse(String question, boolean answer);
/**
* Creates a fill-in-the-blank question.
* @param question The question, including the blanks
* @param answers Array of answers to the blanks
* @return an instance of a fill-in-the-blank question
*/
public IQuestion makeFillInBlank(String question, String [] answers);
/**
* Create a short-answer question.
* @param question The question.
* @param keywords The answers as a list of key words.
* @return an instance of a short answer question.
*/
public IQuestion makeShortAnswer(String question, String[] keywords);
/**
* load a question set using the given filename.
* @param filename The file containing the test set.
* @return A Test set
* @throws IOException if can't load the file.
*/
public IQuestionSet load(String filename) throws IOException;
/**
* Save the test set using the given filename.
* @param testSet The test set to be stored.
* @param filename The filename to be used.
* @return true if save is successful
*/
public boolean save(IQuestionSet testSet, String filename);
/**
* Create an empty test set.
*
* @return an empty test set.
*/
public IQuestionSet makeEmptyQuestionSet();
}
The IQuestionSet represents a collection of questions which serves as a test. For example, a user may create a set of questions named Midterm that has 20 questions in Java. The number of questions supported depends on the implementation. For this exercise, you should use ArrayList as it will simplify your coding. The UML diagram above shows the operations IQuestionSet supports. You will use Serializable interface to support saving your question set.
Implement the extra credit questions to your program.
To encourage you from using the implementation classes directory, you will implement the IQuestionFactory. The factory knows how to create the questions. Your program does not care what the name of the classes of the questions are. It only cares about the method provides by the interfaces. This is the concept of abstraction.
The interfaces should guide you in your implementation of the interfaces. The interfaces contain comments that describe what it is and what each method does. You will find the interfaces in the package com.jsoftware.test.api. You will add your implementation of the interfaces in the package com.jsoftware.test.impl.
The Programs
You will write two console programs that uses the interfaces and your implementation. The first program is named TestMaker which allows users to create test. The second program is named TestTaker lets a user loads an existing test and take it. These programs should be in the package com.jsoftware.test.
To prevent your programs from being dependent on the question implementation, the only class it should know about is the question factory implementation. That makes your program very independent from the implementation. Changes to the names of implementation classes will not affect your program as it doesnt even care about their constructors. This makes your program much more maintainable.
Your solution should implement multiple-choice question, true false question, question factory, question set and the extra credit questions.
Your program must compile and execute. Delivering software that does not compile or run will not make the client happy and you would not get new business from them. Thus, we can not accept programs that do not compile or does not run.
My run on Net Beans:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
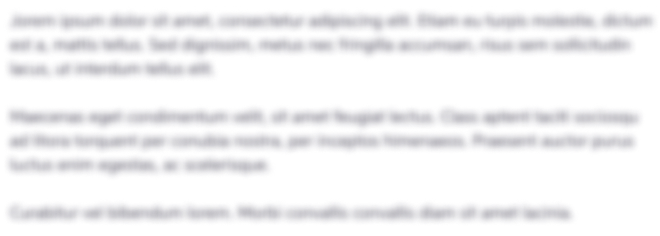
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started