Question
Topics C++ only!! Loops while Statement Description Write a program that computes the letter grades of students in a class from knowing their scores in
Topics
C++ only!!
Loops
while Statement
Description
Write a program that computes the letter grades of students in a class from knowing their scores in a test. A student test score varies from 0 to 100. For a student, the program first asks the students name and the students test score. Then, it displays the student name, the test score and the letter grade. It repeats this process for each student. The user indicates the end of student data by entering two consecutive forward slashes ( // ) when asked for the student name. At the end, the program displays a summary report including the following:
The total number of students.
The total number of students receiving grade A.
The total number of students receiving grade B.
The total number of students receiving grade C.
The total number of students receiving grade D.
The total number of students receiving grade F.
The program calculates a student's the letter grade from the student's test score as follows:
A is 90 to 100 points
B is 80 to 89 points
C is 70 to 79 points
D is 60 to 69 points
F is 0 to 59 points.
Requirements
Do this exercise using a While statement and an If/Else If statement.
Testing
For turning in the assignment, perform the test run below using the input data shown
Test Run (User input is shown in bold).
Enter Student Name
Alan
Enter Student Score
75
Alan 75 C
Enter Student Name
Bob
Enter Student Score:
90
Bob 90 A
Enter Student Name
Cathy
Enter Student Score
80
Cathy 80 B
Enter Student Name
Dave
Enter Student Score:
55
Dave 55 F
Enter Student Name
Eve
Enter Student Score
85
Eve 85 B
Enter Student Name
//
Summary Report
Total Students count 5
A student count 1
B student count: 2
C student count 1
D student 0
F students 1
Sample Code
char grade;
string name;
double score;
int aCount=0, bCount=0, cCount=0, dCount=0, fCount=0;
//Initias setup
cout << "Enter student name" << endl;
cin >> name;
//Test
while (name != "//")
{
cout << "Enter student score" << endl;
cin >> score;
if (score>=90.0){
grade = "A";
aCount++;
}
else if (score>=80.0){
grade = "B";
bCount++;
}
//more code here
cout << name << " " << score << " " << grade << endl;
//Update setup
out << "Enter student name" << endl;
cin >> name;
}
int totalCount=aCount+bCount+cCount+dCount+fCount;
//display summary report
cout<<"Total Students count " << totalCount << endl;
//more code here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
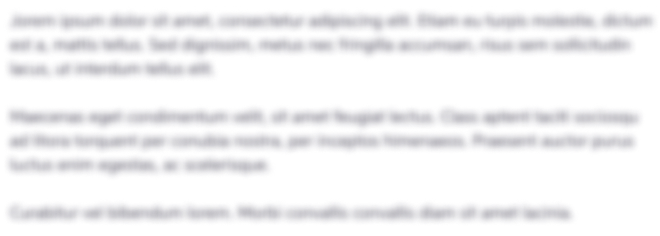
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started