Question
Topics: Separate compilation, class composition, vectors Write a program that manages a schedule of classes for a college. The program will include four classes: DigitalTime,
Topics: Separate compilation, class composition, vectors
Write a program that manages a schedule of classes for a college.
The program will include four classes: DigitalTime, TimeInterval, DaysOfWeek, and Course. The header files for DigitalTime and TimeInterval are provided. Note A description of the DaysOfWeek and Course classes are provided below. You will need to implement all four classes, and write the header file for DaysOfWeek and Course. Finally, you will write a main program that uses a vector of Course objects to implement the application described below.
The Course class:
The Course class represents a single course entry in a schedule of classes. Its member variables should include:
*A course code (a string of alpha-numeric characters)
*A section, which is a string of 3 numeric characters
*The days of the week the course meets (such as MW, TR, MWF, etc.). This is a DaysOfWeek object
*The time the course meets (such as 09:30-10:45 or 17:00-19:50). This is a TimeInterval object
*The instructor, a string of alphabetic characters which uniquely identifies an instructor
Besides the normal set/get member which you will need to implement for the Course class, and any constructors yo wish to use, you will also need to implement 2 additional member functions:
* An isOverlap member function, which takes a second course object. If the 2 courses have the same instructor and overlapping times, the is Overlap member function returns true. Times a and b overlap if the start of a is less than or equal to the end time of b and the end time of a is greater than or equal to the start time of b. Otherwise, it returns false.
* An isMatch member function, which takes a second course object. If the 2 courses have the same course code and section, the isMatch member function returns true. Otherwise, it returns false.
Use isOverlap to overload the && operator. Use isMatch to overload the == operator.
The DaysOfWeek class
Logically, a DaysOfWeek object is a six element Boolean array. Each of the days of the week, except for Sunday, is represented by a letter code: M=Monday, T=Tuesday, W=Wednesday, R=Thursday, F=Friday, S=Saturday. Each day is either present(on), or absent (off). It is possible to have a DaysOfWeek object with no days present, and such an object is produced by the default constructor for the class.
* In addition, you should implement a constructor and a set function that takes a string and, for each day of the week, sets that day to be present if its code letter (in upper or lower case) appears in the input string, and absent otherwise.
* The get member function returns a string of code letters for each day present in the object, all uppercase, in chronological order (i.e. MTWRFS).
* The input member function takes an istream object, removes the first whitespace delimited token, and sets the object using this token as input.
* The output member function takes an ostream object, and sends the results of get to that ostream.
* The isEqual member function returns true for if the host object and DaysOfWeek argument have identical present days, false otherwise.
* The isOverlap function member function returns true for if the host object and DaysOfWeek argument have at least one common present day, false otherwise. Note that this means that 2 DaysOfWeeks objects with no present days do not overlap (online courses).
* Finally, you should overload the &&, ==, and != operators as non-member functions using isOverlap for && and isEqual to implement == and !=.
Functional Requirements
Your program will be driven by a command line prompt. The valid commands are add, clear, import, export, remove, and validate. Command parameters are denoted by < >.
add
clear Delete all courses from the current schedule.
export
For example: export class.txt
import
remove
SFF Format
An SFF file contains a course entry on each line. Each course entry is of the form:
Headers:
1- dtime.h
https://onedrive.live.com/redir?resid=766E6FC39721FBBB!713170&authkey=!AGe2P8d-FlMmWrY&ithint=file%2ch
2- timelnterval.h
https://onedrive.live.com/redir?resid=766E6FC39721FBBB!713169&authkey=!ALMhsPPDBYk5f-Q&ithint=file%2ch
Step by Step Solution
There are 3 Steps involved in it
Step: 1
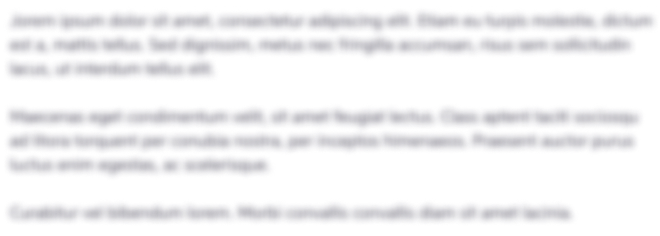
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started