Question
TOPICS: Status, Interfaces DESCRIPTION Write a Java program that takes objects of different classes and provide them the ability to be treated as objects of
TOPICS: Status, Interfaces
DESCRIPTION
Write a Java program that takes objects of different classes and provide them the ability to be treated as objects of a common type. Do this for objects of the following three classes:
Rectangle
Sibling
Student
For accomplishing the above, do the following:
Create an interface Status
Implement the interface Status in each of the above three classes.
Interface Status
The interface Status will contain method header for the following two methods:
Method getStatus
The interface Status will contain header for a method getStatus. The header will be as below:
public String getStatus ( )
Method displayStatus
The interface Status will contain header for a method getStatus. The header will be as below:
public void displayStatus ( )
Implementing Interface Status
In each of the above three classes, implement the interface Status. For this purpose, provide a body for each of the methods in the interface Status.
Method getStatus
This method should return String containing the Status of the object. The status of the object will include the following:
The name of the class.
The name of each instance variable and its value.
For example, for an object of class Rectangle of length 3 and width 2, the String returned as below:
Rectangle Length=3, Width=2
Method displayStatus
This method should display the status of the object. The status of the object should include the following:
The name of the class.
The name of each instance variable and its value.
For example, for an object of class Rectangle of length 3 and width 2, output should be as below:
Rectangle
Length=3, Width=2
IMPLEMENTATION
Create the following interface and classes:
Interface Status
Create an interface named Status containing the following methods:
String getStatus ( )
This method returns the status as a String
void displayStatus ( )
This method displays the status.
class Rectangle
Create a class Rectangle. (Use this class from a previous exercise).
class Sibling
Create a class Sibling. (Use this class from a previous exercise).
class Student
Create a class Student. (Use this class from a previous exercise).
Modifications To The Above Classes
In each of the above classes, implement the interface Status. For this purpose, do the following:
In the class header, add the phrase: implements Status
Add the method getStatus ( ) in the body of the class.
This method returns the status as a String. The string returned includes:
The name of the class on one line.
The name of all instance variables along with their values on a second line.
For example, for an object of class Rectangle of length 3 and width 2, the String returned should be as below:
Rectangle Length=3, Width=2
Add the method displayStatus ( ) in the body of the class.
This method displays the status including the following:
The name of the class on one line.
The name of all instance variables along with their values on a second line.
For example, for an object of class Rectangle of length 3 and width 2, output should be as below:
Rectangle
Length=3, Width=2
For implementing the method displayStatus, you can have this method call the method getStatus and then display the String returned by that method.
class TestStatus
Create a class TestStatus containing the method main ( ). The main method should provide the following:
Create an array of size 6 for storing Status references. (Compiler allows you to create such an array).This array will be populated with references of different objects later.
Create three Rectangle objects, initialize them with data values provided by the user and store their references in the first 3 elements of the Status array. (You can do this because the class Rectangle implements the interface Status and thus a Rectangle object is both of type Rectangle and Status).
Create two Sibling objects, initialize them with data values provided by the user and store their references in the next two elements of the Status array. (You can do this because the class Sibling implements the interface Status and thus a Sibling object is both of type Sibling and Status).
Create one Student objects, initialize it with data values provided by the user and store its reference in the next one element of the Status array. (You can do this because the class Student implements the interface Status and thus a Student object is both of type Student and Status).
Set up a loop to iterate through the array of Status references. (Each reference in the Status array will point to an object).
During each pass through the loop,
Call the method displayStatus ( ) to display status of each object.
Set up a second loop to iterate through the array of Status references. (Each reference in the Status array will point to an object).
During each pass through the loop,
Call the method getStatus ( ) to get the Status of each object as a String, and accumulate the output into a String.
On exit from the loop, display the accumulated output.
ALGORITHMS
Implementing Interface Methods
In each class, first implement the method getStatus that returns the status as a String.
Then implement the method displayStatus. From within the method displayStatus, call the method getStatus and display the string returned by it.
INPUT/OUTPUT
For input/output, you may use either File IO or JOptionPane mechanism.
Input Data:
Data for three Rectangle objects:
3 2
6 4
30 20
Data for two Sibling objects:
Jack 21 130
Judy 24 118
Data for one Student Object:
1,John Adam,3,93,91,100
Output Data:
The output should show the status of each of the object one after the other as below.
Rectangle
length=3, width=2
Rectangle
length=6, width=4
Rectangle
length=30, width=20
Sibling
name=Jack, age=21, weight=130
Sibling
name=Judy, age=24, weight=118
Student
name=John Adam, scores=93, 91, 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
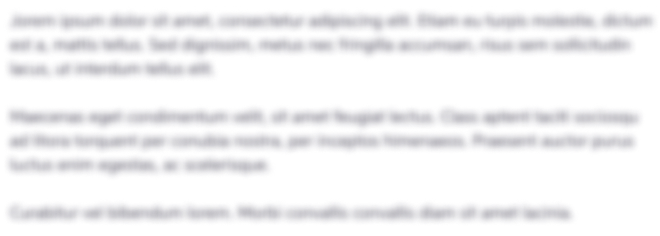
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started