Question
Topics This assignment will give you practice with classes, inheritance, and thinking/designing code with an Object-Oriented approach. Instructions You are going to write a program
Topics
This assignment will give you practice with classes, inheritance, and thinking/designing code with an Object-Oriented approach.
Instructions
You are going to write a program that will allow us to create some ASCII art using an OOP approach. You will need to define two classes (SegmentedLine and SegmentedBox) and then use those classes in combination with a provided class (SegmentedTriangle) in order to produce ASCII art of your own creativity.
SegmentedLine class
You are going to create a class that represents a line that is composed of several "segments" comprising the width of the line. The width might be provided to you, or it might be a random number generated by the program itself. Additionally, the segmented line will be comprised of a particular symbol.
As an example, here is a segmented line with a 5 segment width and a symbol of "&":
&&&&&
Here is a segmented line that has a 20 segment width and a symbol of "-":
--------------------
You are to define a SegmentedLine class according to the specification below. A SegmentedLine should have:
- A class constant for the highest number of segments that a line may contain.
- Fields for number of segments (the width of the line) and the symbol for each segment.
- Three constructors:
- No parameter constructor: generates a random line made up of "-" (dash) symbols. The number of segments should be randomly assigned from 1 to the highest number of segments allowed.
- Single parameter constructor: takes a number of segments and creates a line of that many segments using the symbol "-" (dash) for each segment.
- Two parameter constructor: takes a number of segments and a symbol and creates a segmented line using that information.
- Accessors for both the width and the symbol of the line.
- A mutator for the symbol only.
- A toString that allows for the segmented line to be returned as a String.
- Note that this String representation of the SegmentedLine should not end with a newline character.
Tester Code
After you create (or as you create) your SegmentedLine class, you should write tester code to make sure that it performs as expected. You will need to turn this code in. In this file, everything can be done in the main - it just needs to appropriately test all the functionality of your class by creating an object and then calling various methods on it.
SegmentedBox class
Next you are going to create a subclass of SegmentedLine that inherits the fields and behaviors, adds some new behaviors, and overrides some functionality.
A SegmentedBox is very similar to a SegmentedLine except that it has a height in addition to a width.
For example, here is a SegmentedBox that has a width of 10, a height of 5, and uses the symbol "0":
0000000000 0000000000 0000000000 0000000000 0000000000
Another example. A SegmentedBox with a width of 2, a height of 7, and a symbol "#":
## ## ## ## ## ## ##
You are to define a SegmentedLine class according to the specification below. A SegmentedLine should have:
- Inherit everything from SegmentedLine
- A new field for the height of a SegmentedBox
- Three constructors:
- No parameter constructor: generates a random box made up of "-" (dash) symbols. Both the width and height should be randomly assigned from 1 to the highest number of segments allowed.
- Two parameter constructor: takes a width and height and creates a box of that many segments using the symbol "-" (dash) for each segment.
- Three parameter constructor: takes a width, height, and symbol and creates a segmented box using that information.
- Accessors for the height of the box
- No new mutators
- Override the functionality of toString that allows for the segmented box to be returned as a String.
- Note that this String representation of the SegmentedBox should not end with a newline character.
You should add some testing code to your tester file to test that objects of this type work as expected.
Creating Art in your Main
The final part of this assignment is to design a main that utilizes Objects of type SegmentedLine and SegmentedBox to create something in ASCII art. I am providing you with an additional file that should created a SegmentedTriangle (if everything in your files works properly) and a sample Main
file that utilizes these three objects to create an ASCII art picture of a RocketShip.
Your task is to create YOUR OWN MAIN that:
- creates art that is different from mine
- declares at least one object of each SegmentedLine and SegmentedBox (using SegmentedTriangle is optional)
- changes the symbol of at least one of the objects
Everything in this file may be done in the main, as it is going to be a very short file.
Sample Output
You can download my SegmentedTriangle.java and SegmentedMain.java
. When placed in the same folder as your SegmentedLine and SegmentedBox, my code should produce the following:
!! !!!! !!!!!! !!!!!!!! !!!!!!!!!! ---------- |||||||||| |||||||||| |||||||||| |||||||||| ---------- |||||||||| |||||||||| |||||||||| |||||||||| ---------- ********** ********** ********** ********** ---------- ^^ ^^^^ ^^^^^^ ^^^^^^^^ ^^^^^^^^^^
At the top of your main, answer the following:
In addition to writing code for this assignment, you should also answer the following questions. Make sure that your answers are thorough and detailed. Answers should be approximately 4-7 sentences per question.
- What is the advantage of designing code using an OOP approach? How do we see these advantages in this particular assignment?
- Describe the relationship between the files SegmentedLine, SegmentedBox, SegmentedTriangle, and SegmentedMain using the following vocabulary: superclass, subclass, inherits, creates object(s)/instance(s).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
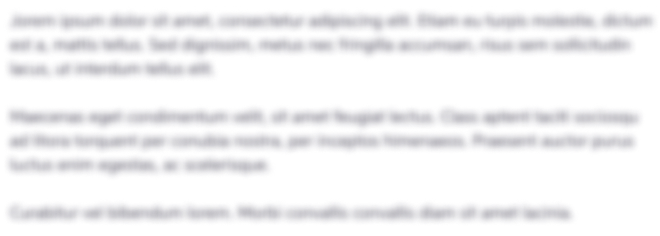
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started