Question
Tortoise and the Hare (expanded) in Java Using the code for Animal.java, Hare.java and Dog.java below complete the assignment. 1. Create an object, Race, with
Tortoise and the Hare (expanded) in Java Using the code for Animal.java, Hare.java and Dog.java below complete the assignment. 1. Create an object, Race, with the following attributes: String event the name of the event int length the length of the race (default of 1000) ArrayList
****Animal.java**** import java.util.*; public class Animal{ public String name; public String species; public double runningSpeed; public double variationOfSpeed; public double currentPosition; public int currentTime; Random random = new Random(); public double roundSpeed; public Animal(String name_, String species_, double runningSpeed_, double variationOfSpeed_, int currentTime_, double currentPosition_){ this.name = name_; this.species = species_; this.runningSpeed = runningSpeed_; this.variationOfSpeed = variationOfSpeed_; this.currentTime = 0; this.currentPosition = currentPosition_; } public Animal(String name_, String species_, double runningSpeed_, double variationOfSpeed_, int currentTime_){ this.name = name_; this.species = species_; this.runningSpeed = runningSpeed_; this.variationOfSpeed = variationOfSpeed_; this.currentTime = 0; } public Animal(String name_, String species_, double runningSpeed_){ this.name = name_; this.species = species_; this.runningSpeed = runningSpeed_; this.variationOfSpeed = 0.1*runningSpeed; this.currentTime = 0; } public String getName(){ return name; } public String getSpecies(){ return species; } public double getRunningSpeed(){ return runningSpeed; } public double getVariationOfSpeed(){ return variationOfSpeed; } public int getCurrentTime(){ return currentTime; } public double getCurrentPosition(){ return currentPosition; } public void setRunningSpeed(double runningSpeed_){ this.runningSpeed = runningSpeed_; } public void setVariationOfSpeed(double variationOfSpeed_){ this.variationOfSpeed = variationOfSpeed_; } public void setCurrentPosition(double currentPosition_){ this.currentPosition = currentPosition_; } public double updatePosition(){ this.roundSpeed = runningSpeed + variationOfSpeed*(random.nextBoolean() ? 1 : -1 ); this.currentPosition = roundSpeed + currentPosition; currentTime += 1; return currentPosition; } }
****Hare.java**** import java.util.*; public final class Hare extends Animal{ private int napStartTime; private int napDuration; public boolean napping; public Hare(String name_, String species_, double runningSpeed_, double variationOfSpeed_, int currentTime_, double currentPosition_, int napStartTime_, int napDuration_, boolean napping_){ super(name_, species_, runningSpeed_, variationOfSpeed_, currentTime_, currentPosition_); this.napStartTime = napStartTime_; this.napDuration = napDuration_; this.napping = napping_; } public double updatePosition(){ if (napping == true){ currentTime += 1; } else{ this.roundSpeed = runningSpeed + variationOfSpeed*(random.nextBoolean() ? 1 : -1 ); this.currentPosition = roundSpeed + currentPosition; currentTime += 1; } return currentPosition; } }
****Dog.java**** import java.util.*; public final class Dog extends Animal{ private int squirrelLocation; public boolean squirrel; public Random squirrelRange = new Random (); public Dog(String name_, String species_, double runningSpeed_, double variationOfSpeed_, int currentTime_, double currentPosition_, int squirrelLocation_, boolean squirrel_){ super(name_, species_, runningSpeed_, variationOfSpeed_, currentTime_, currentPosition_); this.squirrelLocation = squirrelLocation_; this.squirrel = squirrel_; } public boolean toggleSquirrel(){ this.squirrel = !this.squirrel; return squirrel; } public double updatePosition() { if ((squirrelLocation Example Output Event: The Tortoise v. the Hare v the Dog Raced tance {feet): 1000 Contestant 3peedy the Tortoise Running speed Variation of speed 0.2 Contestant 2: Thumper the Hare Running speed Variation of speed: 2.0 8tart nap Nap duration 12.0 20 second 472 second Contestant 3 Doug the Dog Running speed Variation of speed: 1.0 quirrel location 200 feet .0 Aand ,re , re OFF ! ! ! - 3peedy I[TortoiseI Thumpe1 (Hre) Doug Dog I Time I Position 3peedPosition 3peed Conments Fosition 3peed Comnents I 0.0 2.1 2.1 1.8 I 3.9 2.0 I 5.9 2.2 I 0.0 12.3 12.3 14.0 26.3 13.1 39.4 13.2 0.0 8.5 8.5 7.2 15.7 8.1 23. 7.2 fomitted for space) 20 I 21 I 22I 23 I 24 I 25I 2I 27I 28 I 36.1 2.0240.1 00 nap-0 162.1 8.1 38.1 1.8240.1 0.0 nap-1 1702 7.6 39.9 2.1240.1 0.0 nap-2 43.0 2.0240.1 00 nap-3 181 8.1 45.0 1.8240.1 00 nap-4 194.2 7.9 46.8 1.8240.1 0.0 nap-5 202.1 -3.4 squirrel I 48. 2.0240.1 00 nap- 198.7 7.B squirrel I 50.6 2.11 240.1 0.0 nap-7 190.9 6.2squirrell 52.7 1.9240.1 0.0 nap-8 197.1 -2.1 squirrel I 54. 1.8240.1 00 nap-9 195.0 0.2 squirrel I I 29 (omitted for space) 1 494 992.0 2.0240.1 14.0 nap-472281.2 -2.1 squirrel 495 994.0 18 256.0 13.0 496 995.8 19 269.0 12.5 1 279.1 .1 squirrel I 287.2 7.1 294.3 7.0 301.3 8. 997.7 2.1281 497 I 498 999.8 18 94. 12.1 499 1001. 18 06.7 12.3 309.9 8.2 Race over in 499 econd Example Output Event: The Tortoise v. the Hare v the Dog Raced tance {feet): 1000 Contestant 3peedy the Tortoise Running speed Variation of speed 0.2 Contestant 2: Thumper the Hare Running speed Variation of speed: 2.0 8tart nap Nap duration 12.0 20 second 472 second Contestant 3 Doug the Dog Running speed Variation of speed: 1.0 quirrel location 200 feet .0 Aand ,re , re OFF ! ! ! - 3peedy I[TortoiseI Thumpe1 (Hre) Doug Dog I Time I Position 3peedPosition 3peed Conments Fosition 3peed Comnents I 0.0 2.1 2.1 1.8 I 3.9 2.0 I 5.9 2.2 I 0.0 12.3 12.3 14.0 26.3 13.1 39.4 13.2 0.0 8.5 8.5 7.2 15.7 8.1 23. 7.2 fomitted for space) 20 I 21 I 22I 23 I 24 I 25I 2I 27I 28 I 36.1 2.0240.1 00 nap-0 162.1 8.1 38.1 1.8240.1 0.0 nap-1 1702 7.6 39.9 2.1240.1 0.0 nap-2 43.0 2.0240.1 00 nap-3 181 8.1 45.0 1.8240.1 00 nap-4 194.2 7.9 46.8 1.8240.1 0.0 nap-5 202.1 -3.4 squirrel I 48. 2.0240.1 00 nap- 198.7 7.B squirrel I 50.6 2.11 240.1 0.0 nap-7 190.9 6.2squirrell 52.7 1.9240.1 0.0 nap-8 197.1 -2.1 squirrel I 54. 1.8240.1 00 nap-9 195.0 0.2 squirrel I I 29 (omitted for space) 1 494 992.0 2.0240.1 14.0 nap-472281.2 -2.1 squirrel 495 994.0 18 256.0 13.0 496 995.8 19 269.0 12.5 1 279.1 .1 squirrel I 287.2 7.1 294.3 7.0 301.3 8. 997.7 2.1281 497 I 498 999.8 18 94. 12.1 499 1001. 18 06.7 12.3 309.9 8.2 Race over in 499 econd
Step by Step Solution
There are 3 Steps involved in it
Step: 1
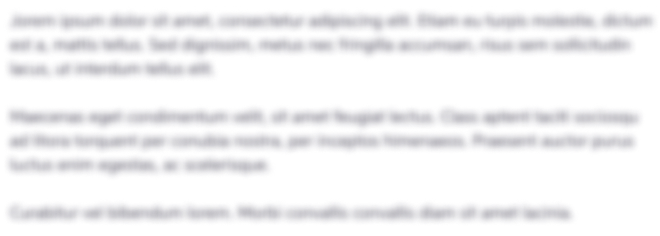
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started