Question
Translating Reading Frames Given a sequence of DNA, it is necessary to examine all six reading frames of the DNA to find the coding regions
Translating Reading Frames
Given a sequence of DNA, it is necessary to examine all six reading frames of the DNA to find the coding regions the cell uses to make proteins.
Very often we won't know where, in the DNA we are studying, the cell begins translating DNA into protein. Since we don't know where the translation begins, we have to consider the six possible reading frames when looking for coding regions.
Your task is to write a program to translate a DNA sequence, given in a GenBank file format called sequence.gb, into all six reading frames as output.
Read the file from the following URL:
http://web.njit.edu/~kapleau/teach/current/bnfo135/sequence.gb
Warning! The file may be changed at any time. Your program must read from the above URL.
Output should be to the screen in the form of "Reading Frame n" where n is a number between 1 and 6 inclusive followed by the translation of the given reading frame.
What follows is an example of output:
--- Reading Frame 1 --- YKMASLIYRQLLTNSYSVDLHDEIEQIGSEKTQNVTVNPGPFAQTRYAPVNWGHGEINDSTTVEPVLDGP YQPTTFKPPNDYWLLISSNTDGVVYESTNNSDFWTAVIAVEPHVSQTNRQYVLFGENKQFNLENSSDKWK FFEMFKGSSQSDFSNRRTLTSNNRLVGMLKYGGRVWTFHGETPRATTDSSNTADLNNISIIIHSEFYIIP RSQESKCNEYINNGLPPIQNTRNVVPLSLSSRSIQYRRAQVNEDITISKTSLWKEMQYNRDIIIRFKFGN SVIKLGGLGYKWSEISYK --- Reading Frame 2 --- IKWLRSFIDNFSLIHIR_TCMTK_NRLDRRKLKM_R_IQAHLHRLDTLQLIGDTERLMIQLQWNQF_MVL INQLHSNHPMIIGCLLVQIQME_SMRVQIIVTFGQQLSLLNHMSVKQIGNMFYLVKISSLT_KIVQINGN FSKCLKVVVRVIFLIDGL_PLIIDL_EC_NMVEEYGHFMVKHQELLLIVRILRI_IIYQL_FIQSFILFQ DPKNLNVMNILIMVYHQFKILEM_FHYLYHPDLFNIEEHKLMKILQFQKLHYGKKCNIIEIL__DLNLVI VS_N_EDWVINGQKYHT --- Reading Frame 3 --- _NGFAHL_TTSH_FIFGRLA_RNRTDWIGENSKCDGKSRPICTD_IRSS_LGTRRD__FNYSGTSFRWSL STNYIQTTQ_LLAAY_FKYRWSSL_EYK___LLDSSYRC_TTCQSNK_AICFIW_K_AV_LRK_FR_MEI FRNV_R__SE_FF__TDFNL___TCRNAKIWWKSMDISW_NTKSYY__FEYCGFK_YINYNSFRVLYYSK IPRI_M__IY__WFTTNSKY_KCSSIISIIQIYSI_KSTS__RYYNFKNFIMERNAI__RYYNKI_IW__ CHKIRRIGL_MVRNIIQ --- Reading Frame 4 --- FV_YF_PFITQSS_FYDTITKFKSYYNISIILHFFP__SF_NCNIFINLCSSILNRSG__R_WNYISSIL NWW_TIINIFITFRFLGSWNNIKL_MNYN_YII_IRSIRTISSSSWCFTMKCPYSSTIF_HSYKSIIRG_ SPSIRKITLTTTFKHFEKFPFI_TIF_VKLLIFTK_NILPICLTDMWFNSDNCCPKVTIICTLIDYSICI _TNKQPIIIGWFECSWLIRTI_NWFHCS_IINLSVSPINWSVSSLCKWAWIYRHILSFLRSNLFYFVMQV YRI_ISEKLSINERSHFI --- Reading Frame 5 --- LYDISDHL_PNPPNFMTLLPNLNLIIISLLYCISFHNEVFEIVISSLTCALLY_IDLDDRDNGTTFLVF_ IGGKPLLIYSLHLDSWDLGII_NSE_IIIDILFKSAVFELSVVALGVSP_NVHTLPPYFSIPTSLLLEVK VRLLEKSL_LLPLNISKNFHLSELFSKLNCLFSPNKTYCLFV_LTCGSTAITAVQKSLLFVLS_TTPSVF ELISSQ_SLGGLNVVG__GPSKTGSTVVESLISPCPQLTGAYLVCANGPGFTVTF_VFSDPICSISSCKS TEYELVRSCL_MSEAIL --- Reading Frame 6 --- CMIFLTIYNPILLIL_HYYQI_ILL_YLYYIAFLSIMKFLKL_YLH_LVLFYIE_IWMIEIMELHF_YFE LVVNHY_YIHYI_ILGILE_YKTLNEL_LIYYLNPQYSNYQ__LLVFHHEMSILFHHILAFLQVYY_RLK SVY_KNHSDYYL_TFRKISIYLNYFLS_TAYFHQIKHIAYLFD_HVVQQR_LLSKSHYYLYSHRLLHLYL N__AANNHWVV_M_LVDKDHLKLVPL_LNH_SLRVPN_LERI_SVQMGLDLPSHFEFSPIQSVLFRHASL PNMN__EVVYK_AKPFY
*use this code to start*
from urllib.request import urlopen
def dna2rna(seq):
''' The dna2rna function converts a sequence of DNA, given as a
parameter and returns an RNA sequence.
'''
return ''
codon2aa = {'aaa': 'K', 'aac': 'N', 'aag': 'K', 'aau': 'N',
'aca': 'T', 'acc': 'T', 'acg': 'T', 'acu': 'T',
'aga': 'R', 'agc': 'S', 'agg': 'R', 'agu': 'S',
'aua': 'I', 'auc': 'I', 'aug': 'M', 'auu': 'I',
'caa': 'Q', 'cac': 'H', 'cag': 'Q', 'cau': 'H',
'cca': 'P', 'ccc': 'P', 'ccg': 'P', 'ccu': 'P',
'cga': 'R', 'cgc': 'R', 'cgg': 'R', 'cgu': 'R',
'cua': 'L', 'cuc': 'L', 'cug': 'L', 'cuu': 'L',
'gaa': 'E', 'gac': 'D', 'gag': 'E', 'gau': 'D',
'gca': 'A', 'gcc': 'A', 'gcg': 'A', 'gcu': 'A',
'gga': 'G', 'ggc': 'G', 'ggg': 'G', 'ggu': 'G',
'gua': 'V', 'guc': 'V', 'gug': 'V', 'guu': 'V',
'uaa': '_', 'uac': 'Y', 'uag': '_', 'uau': 'Y',
'uca': 'S', 'ucc': 'S', 'ucg': 'S', 'ucu': 'S',
'uga': '_', 'ugc': 'C', 'ugg': 'W', 'ugu': 'C',
'uua': 'L', 'uuc': 'F', 'uug': 'L', 'uuu': 'F'}
if __name__ == '__main__':
with urlopen('http://web.njit.edu/~kapleau/teach/current/bnfo135/sequence.gb') as conn:
data = conn.readlines()
lines = [line.strip() for line in [datum.decode() for datum in data]]
flag = False
dna = ''
for line in lines:
## if the flag is 'True', append the line to 'dna'.
## if the word "ORIGIN" is in the line, set 'flag' to 'True'
pass
## gets rid of any non-dna character.
dna = dna.translate(str.maketrans('acgt', 'acgt', '0123456789 /'))
## calls the dna2rna function
rna = dna2rna(dna)
## process the first 3 reading frames
for i in range(3):
## create a variable 'seq' and assign it the rna to process
seq = ''
amino = ''
while len(seq) >= 3:
## use the codon2aa table to append an amino acid to 'amino'
## update 'seq' to the next codon
pass
print('--- Reading Frame %i ---' % (i+1), amino, sep=' ')
## compute the reverse complement of 'rna' and assign the result
## back into the 'rna' variable
## process the next 3 reading frames. hint: just like the first 3
for i in range(3):
## same as the first 3
print('--- Reading Frame %i ---' % (i+4), amino, sep=' ')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
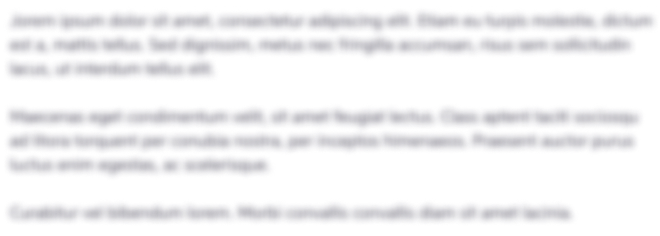
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started