Question
Trying to figure out how to take this Javascript code and make it generate a randomized password following the parameters set by the prompts (length,
Trying to figure out how to take this Javascript code and make it generate a randomized password following the parameters set by the prompts (length, lowercase, uppercase, numbers, special characters.)
function randomNum(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
function getLength() {
var entry = '';
entry = prompt ("How long will your password be?");
entry = parseInt(entry);
if (entry >= 8 && entry <= 128) {
return entry;
} else {
window.alert("Try again. Please enter a number between 8 and 128");
}
}
function gatherChoices() {
var answers = {
lowercase: confirm("Will you be using lowercase letters?"),
uppercase: confirm("How about uppercase letters?"),
numbers: confirm("Numbers?"),
specialChar: confirm("Maybe some special characters?")
};
if (answers.lowercase || answers.uppercase || answers.numbers || answers.specialChar) {
return answers;
} else {
window.alert("You shall not pass! Please make at least one character selection.")
}
}
function generatePassword() {
var length = getLength();
var answers = gatherChoices();
var characters = {
uppercase: ["A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z"],
lowercase: ["a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z"],
numbers: ["1","2","3","4","5","6","7","8","9","0"],
specialChar: ["!","@","#","$","%","^","&","*","?","+","-","="]
};
}
var generateBtn = document.querySelector("#generate");
function writePassword() {
var password = generatePassword();
var passwordText = document.querySelector("#password");
getLength.entry = generatePassword.length;
passwordText.value = password;
}
generateBtn.addEventListener("click", writePassword);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
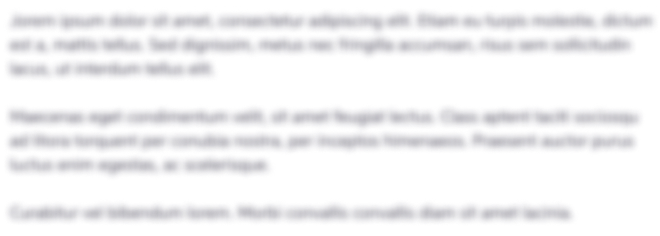
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started