Question
trying to get this code public class Clock { private int hours; private int minutes; private int seconds; public Clock() { this.hours = 0; this.minutes
trying to get this code
public class Clock {
private int hours; private int minutes; private int seconds;
public Clock() { this.hours = 0; this.minutes = 0; this.seconds = 0; }
public Clock(int hours, int minutes, int seconds) { this.hours = hours; this.minutes = minutes; this.seconds = seconds; }
public int getHours() { return hours; }
public void setHours(int hours) { this.hours = hours; }
public int getMinutes() { return minutes; }
public void setMinutes(int minutes) { this.minutes = minutes; }
public int getSeconds() { return seconds; }
public void setSeconds(int seconds) { this.seconds = seconds; }
@Override public String toString() { return hours + ":" + minutes + ":" + seconds; } }
class ExtClock extends Clock { private String timeZone; public ExtClock() { setTime(0, 0, 0, "unknown"); } public ExtClock(int hours, int minutes, int seconds, String zone) { super(hours, minutes, seconds); timeZone = zone; } public void setTime(int hours, int minutes, int seconds, String zone) { timeZone = zone; } public void setTimeZone(String zone) { timeZone = zone; } public String getTimeZone() { return timeZone; } @Override public String toString() { return (super.toString() + " " + timeZone); } }//end of class
class TestClock { public static void main(String[] args) { ExtClock clockOne = new ExtClock(7, 30, 22, "Mountain"); ExtClock clockTwo = new ExtClock(); ExtClock clockThree = new ExtClock(14, 7, 35, "Central"); ExtClock clockFour = new ExtClock(); System.out.println(clockOne); System.out.println(clockTwo); System.out.println(clockThree); System.out.println(clockFour);
}//end of main }//end of class
trying to call this code from a java file or add this code to current code so that the ouotput also shows the current time and date
import java.util.*;
class GetCurrentDateAndTime
{
static void main(String args[])
{
int day, month, year;
int second, minute, hour;
GregorianCalendar date = new GregorianCalendar();
day = date.get(Calendar.DAY_OF_MONTH);
month = date.get(Calendar.MONTH);
year = date.get(Calendar.YEAR);
second = date.get(Calendar.SECOND);
minute = date.get(Calendar.MINUTE);
hour = date.get(Calendar.HOUR);
System.out.println("Current date is "+day+"/"+(month+1)+"/"+year);
System.out.println("Current time is "+hour+" : "+minute+" : "+second);
}
}
java file name GetCurrentDateAndTime.java
Step by Step Solution
There are 3 Steps involved in it
Step: 1
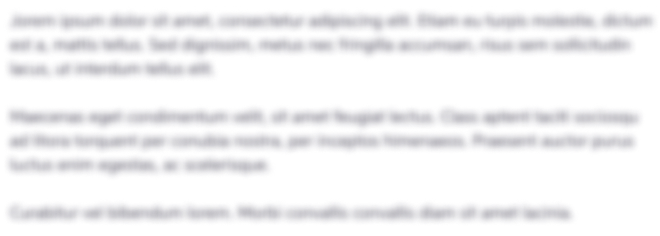
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started