Answered step by step
Verified Expert Solution
Question
1 Approved Answer
*tutoring pursposes only* Add a method to class Graph to write a code that the graph is either an undirected graph or a connected graph.
*tutoring pursposes only* Add a method to class Graph to write a code that the graph is either an undirected graph or a connected graph.
attached is the class graph
please explain how if you can. thanks
class Graph:
def __init__(self, directed=False):
"""Create an empty graph (undirected, by default).
Graph is directed if optional parameter is set to True.
"""
self._outgoing = {}
# Only create second map for directed graph; use alias for undirected
if directed:
self._incoming = {}
else:
self._incoming = self._outgoing
def _validate_vertex(self, v):
"""Verify that v is a Vertex of this graph."""
if not isinstance(v, Vertex):
raise TypeError('Vertex expected')
if v not in self._outgoing:
raise ValueError('Vertex does not belong to this graph.')
def is_directed(self):
"""Return True if this is a directed graph; False if undirected.
Property is based on the original declaration of the graph, not its contents.
"""
return self._incoming is not self._outgoing # directed if maps are distinct
def vertex_count(self):
"""Return the number of vertices in the graph."""
return len(self._outgoing)
#
def vertices(self):
return self._outgoing.keys()
#
def vertices_labels(self):
vertices = self.vertices()
set_of_labels = set()
for i in vertices:
set_of_labels.update(set(i.__str__()))
return set_of_labels
def printVertices(self):
set_of_labels = self.vertices_labels()
print(set_of_labels)
def edge_count(self):
"""Return the number of edges in the graph."""
total = sum(len(self._outgoing[v]) for v in self._outgoing)
#for undirected graphs, make sure not to double-count edges
return total if self.is_directed() else total // 2
#
def edges(self):
"""Return a set of all edges of the graph."""
result = set() # avoid double-reporting edges of undirected graph
for dict in self._outgoing.values():
for edge in dict.values():
result.update(edge._label) # add edges to resulting set
return result
#
def get_edge(self, u, v):
"""Return the edge from u to v, or None if not adjacent."""
self._validate_vertex(u)
self._validate_vertex(v)
return self._outgoing[u].get(v) # returns None if v not adjacent
#
def degree(self, v, outgoing=True):
"""Return number of (outgoing) edges incident to vertex v in the graph.
If graph is directed, optional parameter used to count incoming edges.
"""
self._validate_vertex(v)
adj = self._outgoing if outgoing else self._incoming
return len(adj[v])
def incident_edges(self, v, outgoing=True):
"""Return all (outgoing) edges incident to vertex v in the graph.
If graph is directed, optional parameter used to request incoming edges.
"""
self._validate_vertex(v)
adj = self._outgoing if outgoing else self._incoming
for edge in adj[v].values():
#Changed yield edge.__str__()
yield edge
#
def insert_vertex(self, v):
#"""Insert and return a new vertex with element x."""
if not isinstance(v, Vertex):
raise TypeError('Vertex expected')
if v._label in self.vertices_labels() or v._label in self.edges():
raise ValueError('Labels must be unique')
self._outgoing[v] = {}
if self.is_directed():
self._incoming[v] = {} # need distinct map for incoming edges
return v
def insert_edge(self, e):
"""Insert and return an edge e to the graph.
Raise a ValueError if u and v are not vertices of the graph.
Raise a ValueError if u and v are already adjacent.
"""
u = e._origin
v = e._destination
if u not in self._outgoing or v not in self._outgoing:
raise ValueError('u and v most be vertices in the graph')
if self.get_edge(u, v) is not None: # includes error checking
raise ValueError('u and v are already adjacent')
if e._label in self.vertices() or e._label in self.edges():
raise ValueError('Labels must be unique')
self._outgoing[u][v] = e
self._incoming[v][u] = e
def remove_edge(self, e):
u = e._origin
v = e._destination
if u not in self._outgoing or v not in self._outgoing:
raise ValueError('u and v most be vertices in the graph')
#if self.get_edge(u, v) is None: # includes error checking
#raise ValueError('u and v are already adjacent')
del self._outgoing[u][v]
del self._incoming[v][u]
if __name__ == "__main__":
u = Vertex("u")
v = Vertex("v")
w = Vertex("w")
x = Vertex("x")
g1 = Graph()
g1.insert_vertex(u)
g1.insert_vertex(v)
g1.insert_vertex(w)
g1.insert_vertex(x)
e = Edge(u, v, "e")
f = Edge(u, w, "f")
g1.insert_edge(e)
g1.insert_edge(f)
#print(g1.edges())
#g1.remove_edge(f)
#print(g1.edges())
#print(g1._validate_vertex(e))
#print(g1.is_directed())
#print(g1.vertex_count())
#print(g1.edge_count())
#print(g1.vertices())
#g1.printVertices()
#print(g1._outgoing)
#print(g1.edges())
#print(g1.get_edge(u, v))
#print(g1.degree(v))
#for edge in g1.incident_edges(u, outgoing=True):
Step by Step Solution
There are 3 Steps involved in it
Step: 1
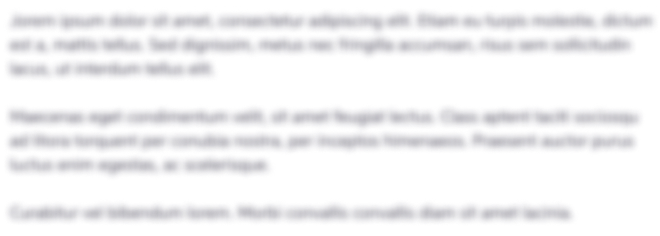
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started