Question
Type setup q5 to obtain the program used in this quiz. A makefile has been supplied that includes -g as one of the compiler options.
Type setup q5 to obtain the program used in this quiz.
A makefile has been supplied that includes -g as one of the compiler options. You can build the program simply by typing
make
To run the resulting executable program in the debugger, type:
gdb quiz5 As written, the program has no compile time errors, compile time warnings, nor run time errors. Using the debugger, answer the questions given below. In order to answer the questions, you will need to set breakpoints in the program, single step through the executing program, examine values of variables and change the values of variables.
In order to insure that your answers match the correct answers, this program must be run on turing/hopper. Answers obtained running on Linux / macOS on your machine at home will be different.
The program requires user input. You'll know you've reached this point in the debugger when you hit return and don't get a debugger prompt (gdb). Type in the desired input at this point and hit enter. Be very careful about the input values. An incorrect input value will lead to completely different answers.
The seed values are based on a sequence of dates (2/14/2021, 2/15/2021, etc.). Be sure to use the seed values given or your answers will differ greatly from the correct answers.
When answering questions, your response should consist solely of an integer number and nothing else. Do not include additional text like "array[10] is 5" or "index 15 of the array". Blackboard is looking for exact matches and any additional text beyond the correct number will be marked as wrong.
ALL QUESTIONS ARE DIFFERENT PARTS OF THE SAME INTIAL CODE/QUESTION. ANSWER AS MANY AS YOU CAN
Source:
GDB Debugger Reference Compiling with Debugging Symbols Pass the -g flag to your compiler: z1234567@turing:~/csci241/Assign1$ g++ -Wall std=c++11 -g -o assign1 assign1.cpp Note: If you have a larger program with several files, each must be compiled with the -g flag, and it must also be set when you link. If you have written a makefile, you can easily add the -g flag to the list of compiler variables: # # PROGRAM: assign1 # PROGRAMMER: Ima Coder # LOGON ID: z1234567 # DATE DUE: 9/14/2027 # # Compiler variables CXX = g++ CXXFLAGS = -Wall -std=c++11 g # Rule to link object code files to create executable file assign1: assign1.o $(CXX) $(CXXFLAGS) -o assign1 assign1.o ... Setting Your Default Editor Its very handy to be able to edit your source files from within the gdb debugger using the edit command. To enable this capability, you must specify a value for the shell environment variable EDITOR. Change to your home directory and open the file .bash_profile in a text editor. Add the following line to the end of the file: export EDITOR="/usr/binano" Note that you can specify a different pathname if you want a different editor such as Vim ("/usr/bin/vim") or Emacs ("/usr/bin/emacs"). Save the file and exit. Then, either log out and log back in, or type the command source .bash_profile Starting the Debugger Start the debugger with your executable program name as the first argument. For example, if the name of the executable file is assign1, then you need to type: gdb assign1 Heres an example of what youll typically see when the debugger starts: z1234567@turing:~/csci241/Assign1$ gdb assign1 GNU gdb (Debian 7.7.1+dfsg-5) 7.7.1 Copyright (C) 2014 Free Software Foundation, Inc. License GPLv3+: GNU GPL version 3 or later This is free software: you are free to change and redistribute it. There is NO WARRANTY, to the extent permitted by law. Type "show copying" and "show warranty" for details. This GDB was configured as "x86_64-linux-gnu". Type "show configuration" for configuration details. For bug reporting instructions, please see: . Find the GDB manual and other documentation resources online at: . For help, type "help". Type "apropos word" to search for commands related to "word"... Reading symbols from assign1...done. (gdb) You can now type debugger commands at the gdb prompt. Some of the most commonly used gdb commands are listed on the next two pages. This list is not exhaustive, and there are many other commands and options available. Command Description Examples Getting help help Get a list of classes of debugger commands. help help class-name Get a list of all commands in the specified command class. help breakpoints help command Get documentation for a specific command within a class. help break Setting command-line arguments and redirection set args arg1 arg2 Specify command-line arguments. You may also use this command to redirect input or output for the program. set args in.txt 20 set args in.txt > out.txt set args Cancel previous command-line arguments and redirection. set args Executing the program run Run the program you are debugging. The program will run until it terminates or it hits a breakpoint. run continue Continue executing program being debugged after it has hit a breakpoint. Execution will continue until termination or the next breakpoint. This command may be abbreviated as c. c finish Continue executing until the current function returns. finish next Execute next program statement, stepping over subroutine calls. This command may be abbreviated as n. n next n Step over next n program statements. next 3 n 4 step Execute next program statement, stepping into subroutine calls. This command may be abbreviated as s. s step n Step into next n program statements. step 2 kill Kill execution of program being debugged. kill Breakpoints break n Set a breakpoint on line n of the current source file. The command tbreak can be used instead to set a temporary breakpoint that will only be triggered once. break 51 break filename:n Set a breakpoint on line n of the specified source file. break other.cpp:32 break function-name Set a breakpoint at the beginning of the specified function. break buildArray break function-name Set a breakpoint at the beginning of the specified template function. break compare break class-name::function-name Set a breakpoint at the beginning of the specified C++ member function. break Date::print break class-name::function-name Set a breakpoint at the beginning of the specified C++ template member function. break Stack::push info breakpoints Show status of breakpoints. Can optionally be followed by a list of specific breakpoint numbers; defaults to all breakpoints info breakpoints info breakpoints 1 3 disable breakpoints n1 n2 Disable specified breakpoint numbers. Defaults to all. May be abbreviated as disable. disable 3 enable breakpoints n1 n2 Enable specified breakpoint numbers. Defaults to all. May be abbreviated as enable. enable 2 3 delete breakpoints n1 n2 Delete specified breakpoint numbers. Defaults to all. May be abbreviated as delete. delete 1 Command Description Examples Watchpoints watch expression A watchpoint stops execution of your program whenever the value of the specified expression changes. A watchpoint is a specific type of breakpoint and can be enabled, disabled, or deleted using the same commands. watch playerName watch location expression Evaluates expression and watches the memory location to which it refers. -location may be abbreviated as -l. watch -l ptrName info watchpoints Show status of watchpoints only. Can optionally be followed by a list of specific watchpoint numbers; defaults to all watchpoints info watchpoints info watchpoints 1 3 Examining and modifying variables whatis expression Print the data type of the specified expression. whatis num print expression Print the current value of the specified expression or variable name. Can be abbreviated as p. print num p providerArray[3] set var variable = expression Set the specified variable to the specified expression. set var x = 3 display expression Print value of expression each time debugger stops. display num info display Lists expressions to display when program stops, with code numbers. info display disable display n1 n2 Disable specified display expression code numbers. Defaults to all. disable 3 enable display n1 n2 Enable specified display expression code numbers. Defaults to all. enable 2 3 undisplay n1 n2 Cancel the specified display expression code numbers. Defaults to all. undisplay 1 info locals Prints values of local variables in the current stack frame. info locals Listing source code list List ten more lines after or around previous listing. list list n List ten lines around the specified line. Line number arguments may be preceded by a filename. list 51 list Provider.cpp:20 list function List ten lines around the specified function. list buildArray list class-name::function-name List ten lines around the specified C++ member function. list Provider::print list + List the ten lines after the previous listing. list + list - List the ten lines before the previous listing. list - list x,y List the specified range of line numbers. list 10,35 Program stack backtrace Print backtrace of all program stack frames. May be abbreviated as bt. bt backtrace full Print backtrace of all program stack frames, including local variables. bt full Other commands make Run the make program using the rest of the line as arguments. make make clean file executable-filename Use executable-filename as program to be debugged. file assign1 edit Edit a source or header file. edit edit filename:n Edit at the specified line number in the specified file. edit Date.cpp:10 edit function Edit at the beginning of the specified function. May be optionally preceded by a filename. edit buildArray edit sorts.cpp:compare edit class-name::function-name Edit at the beginning of the specified C++ member function. edit Provider::print quit Exit the debugger. May be abbreviated q. quit How to Debug Using gdb The backtrace command can give you an immediate sense of the sequence of method calls that resulted in your runtime error. That should help you to localize the last statement that executed before your program abnormally terminated. Unfortunately, the last statement executed by your program is not necessarily the one with a bug. Mistakes earlier in the program may not manifest immediately, particularly when it comes to a runtime error like a segmentation fault. Its also entirely possible to fix one runtime error only to reveal another one. Usually, you will need to create at least one breakpoint in order to do anything useful. If you suspect that a particular function is causing your runtime error, place a breakpoint on that function. Alternatively, use the list command to list your source code and place a breakpoint on a specific line number. If you have no idea where the error is happening, start by putting a breakpoint on one of the first lines inside your main() function. Use run to make the program run until it hits your first breakpoint. Remember that the lines displayed in gdb as the program is executing represent the next statement to be executed. Advance line-by-line through the program code using the next or step commands or continue running it until your next breakpoint by using the continue command. As you step through the program, you can examine the values of variables using the print and display commands or info locals. To find the bug that is causing your runtime error, you need to know what the values of your variables should be at any given point in the program as well as what the values actually are. Using good test data can make this much easier!
QUESTION 1 Set a breakpoint on line 21. Run the program. Advance through the program using the next command and enter a seed value of 2142021. After calling the shuffle() function, what is the value of array[8]? QUESTION 2 Restart the program and run it with a seed value of 2152021. After calling the fill() function but before calling the shuffle () function, what is the value of array(13]? QUESTION 3 Restart the program and run it with a seed value of 2162021. Step into the fill() function. Inside the loop, when i == 14, what is the value of index after assignment? (i.e., on Line 40 after executing the statement index = rand() $ size;) QUESTION 4 Restart the program and run it with a seed value of 2172021. Step into the fill() function. Set a watchpoint on array[29] (you can use the gdb command watch to do this). Continue the execution of the program. When it stops, what is the new value of array[29]? QUESTION 5 Continue the execution of the program. When it stops for the second time, what is the new value of array[29]? After answering, delete the watchpoint using the delete command. QUESTION 6 Restart the program and run it with a seed value of 2182021. After the call to fill() and before the call to shuffle (), replace the value in array[26] with a -1. (Use the gdb command set var to do this.) Verify that your change is in place. After calling shuffle (), what is the subscript of the array element that contains the -1? QUESTION 7 Restart the program and run it with a seed value of 2192021. Step into the shuffle () function. When j == 7, what is the value of the expression first - array after Line 51 has been executed? QUESTION 8 Restart the program and run it with a seed value of 2202021. Step into the shuffle() function. When j == 4, what is the value of the expression second - array after Line 52 has been executed? QUESTION 1 Set a breakpoint on line 21. Run the program. Advance through the program using the next command and enter a seed value of 2142021. After calling the shuffle() function, what is the value of array[8]? QUESTION 2 Restart the program and run it with a seed value of 2152021. After calling the fill() function but before calling the shuffle () function, what is the value of array(13]? QUESTION 3 Restart the program and run it with a seed value of 2162021. Step into the fill() function. Inside the loop, when i == 14, what is the value of index after assignment? (i.e., on Line 40 after executing the statement index = rand() $ size;) QUESTION 4 Restart the program and run it with a seed value of 2172021. Step into the fill() function. Set a watchpoint on array[29] (you can use the gdb command watch to do this). Continue the execution of the program. When it stops, what is the new value of array[29]? QUESTION 5 Continue the execution of the program. When it stops for the second time, what is the new value of array[29]? After answering, delete the watchpoint using the delete command. QUESTION 6 Restart the program and run it with a seed value of 2182021. After the call to fill() and before the call to shuffle (), replace the value in array[26] with a -1. (Use the gdb command set var to do this.) Verify that your change is in place. After calling shuffle (), what is the subscript of the array element that contains the -1? QUESTION 7 Restart the program and run it with a seed value of 2192021. Step into the shuffle () function. When j == 7, what is the value of the expression first - array after Line 51 has been executed? QUESTION 8 Restart the program and run it with a seed value of 2202021. Step into the shuffle() function. When j == 4, what is the value of the expression second - array after Line 52 has been executedStep by Step Solution
There are 3 Steps involved in it
Step: 1
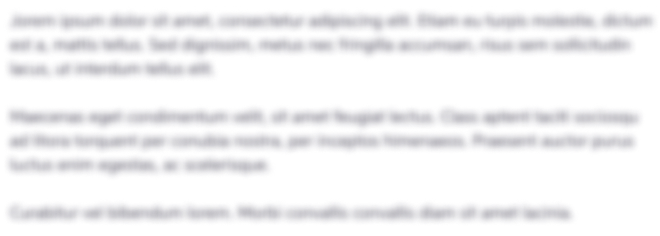
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started