Question
UALDictionary.java import java.util.ArrayList; import java.util.Collections; class UALDictionary implements Dictionary { private static final int defaultSize = 10; private ArrayList > list; // Constructors UALDictionary() {
UALDictionary.java
import java.util.ArrayList;
import java.util.Collections;
class UALDictionary
private static final int defaultSize = 10;
private ArrayList
// Constructors
UALDictionary() {
this(defaultSize);
}
UALDictionary(int sz) {
list = new ArrayList
}
public void clear() {
list.clear();
}
/** Insert an element: append to list */
public void insert(Key k, E e) {
KVpair
list.add(temp);
}
/** Remove element with key k, return element */
public E remove(Key k) {
E temp = find(k);
if (temp != null)
list.remove(new KVpair
return temp;
}
/** Remove any element */
public E removeAny() {
return list.remove(list.size()-1).value();
}
/**
* Find k using sequential search
*
* @return Record with key value k
*/
public E find(Key k) {
for (KVpair
if (k.compareTo(t.key())==0)
return t.value();
return null; // "k" does not appear in dictionary
}
public Iterable
ArrayList
for (KVpair
if (k.compareTo(t.key()) == 0)
al.add(t.value());
return al;
}
public int size(){
return list.size();
}
/** Returns an iterable collection of the dictionary. */
public Iterable
ArrayList
for(KVpair
elements.add(t.value());
return elements;
}
}
=============================================================================================
Pronunciation.java
import java.util.LinkedList;
import java.util.Scanner;
public class Pronunciation {
private String word;
private String pronounce;
Pronunciation(String p) {
int i = p.indexOf(' ');
word = p.substring(0, i);
pronounce = p.substring(i+1);
}
public String getWord() {
return word;
}
public String getPhonemes() {
return pronounce;
}
public String toString() {
String s = word + " " + pronounce;
return s;
}
}
====================================================================================
OALDictionary.java
import java.util.ArrayList;
class OALDictionary
private static final int defaultSize = 10;
private ArrayList
// Constructors
OALDictionary() {
this(defaultSize);
}
OALDictionary(int sz) {
list = new ArrayList
}
public void clear() {
list.clear();
}
/** Insert an element: append to list */
public void insert(Key k, E e) {
KVpair
int i = 0;
while ((i 0))
++i;
list.add(i, temp);
}
/** Remove element with key k, return element */
public E remove(Key k) {
E temp = find(k);
if (temp != null)
list.remove(new KVpair
return temp;
}
/** Remove any element */
public E removeAny() {
return list.remove(list.size()-1).value();
}
/**
* Find k using sequential search
*
* @return Record with key value k
*/
public E sfind(Key k) {
for (KVpair
if (k.compareTo(t.key()) == 0)
return t.value();
return null; // "k" does not appear in dictionary
}
/**
* Find k using binary search
*
* @return Record with key value k
*/
public E find(Key k) {
int low = 0;
int hi = list.size() - 1;
int mid = (low + hi) / 2;
while (low
if (k.compareTo(list.get(mid).key()) == 0)
return list.get(mid).value();
else if (k.compareTo(list.get(mid).key()) > 0)
low = mid+1;
else
hi = mid-1;
mid = (low + hi) / 2;
}
return null; // "k" does not appear in dictionary
}
/* findAll implementation using inefficient sequential search.
*
*/
public Iterable
ArrayList
for (KVpair
if (k.compareTo(t.key()) == 0)
al.add(t.value());
return al;
}
public Iterable
ArrayList
int fidx = -1;
int low = 0;
int hi = list.size() - 1;
int mid = (low + hi) / 2;
while ((low
if (k.compareTo(list.get(mid).key()) == 0)
fidx = mid;
else if (k.compareTo(list.get(mid).key()) > 0)
low = mid+1;
else
hi = mid-1;
mid = (low + hi) / 2;
}
if(fidx
int i = fidx;
for(i=fidx; i>=0;--i)
if(k.compareTo(list.get(i).key()) != 0)
break;
fidx = i+1; // the first instance of key k
for(i=fidx; i { if(k.compareTo(list.get(i).key()) == 0) al.add(list.get(i).value()); else break; } return al; } public int size() { return list.size(); } /** Returns an iterable collection of the dictionary. */ public Iterable ArrayList for (KVpair elements.add(t.value()); return elements; } } ===================================================================================== KVpair.java /** Container class for a key-value pair */ class KVpair private Key k; private E e; /** Constructors */ KVpair() { k = null; e = null; } KVpair(Key kval, E eval) { k = kval; e = eval; } /** Data member access functions */ public Key key() { return k; } public E value() { return e; } } ======================================================================================== Homophone.java import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; import java.util.ArrayList; /* * Identify 5-letter word that has the same pronunciation as the word with first * letter removed and with second letter removed. (Car Talk puzzler.) */ public class Homophone { public static void main(String[] args) { UALDictionary File file = new File("cmudict.0.7a.txt"); //File file = new File("shuffledDictionary.txt"); final int len = 5; // we start with words of length 5 characters long start = System.currentTimeMillis(); try { Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); if (line.substring(0, 3).equals(";;;")) continue; // skip comment lines Pronunciation p = new Pronunciation(line); if ((p.getWord().length() || (p.getWord().length() > len)) continue; if ((p.getWord().length() == len - 1) || (p.getWord().length() == len)) PDict.insert(p.getWord(), p); } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } long middle = System.currentTimeMillis(); System.out.println("Loaded dictionary."); for (Pronunciation p : PDict.values()) { String w = p.getWord(); if (w.length() == len) { String w1 = w.substring(1); // word obtained by removing first letter String w2 = w.substring(0, 1) + w.substring(2); // and removing second letter Pronunciation p1 = PDict.find(w1); Pronunciation p2 = PDict.find(w2); if ((p1 != null) && (p2 != null) && (p.getPhonemes().equals(p1.getPhonemes())) && (p1.getPhonemes().equals(p2.getPhonemes()))) { System.out.println("An answer is: " + w); } } } long end = System.currentTimeMillis(); System.out.println("Run times: load dictionary= " + (middle - start) + " process= " + (end - middle) + " total= " + (end - start)); } } Dictionary.java public interface Dictionary public void clear(); public void insert(Key k, E e); public E remove(Key k); // Null if none public E removeAny(); // Null if none public E find(Key k); // Null if none public Iterable public Iterable public int size(); };
Step by Step Solution
There are 3 Steps involved in it
Step: 1
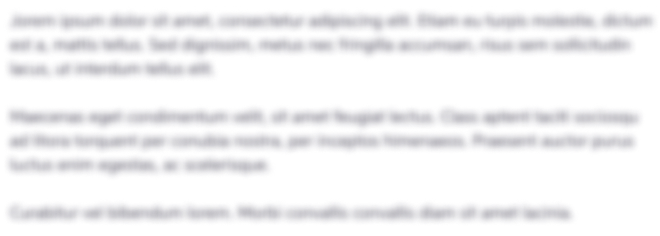
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started