Question
Understanding Sequential Statements Summary In this lab, you complete a Java program with the provided data files. The program calculates the amount of tax withheld
Understanding Sequential Statements
Summary
In this lab, you complete a Java program with the provided data files. The program calculates the amount of tax withheld from an employees weekly salary, the tax deduction to which the employee is entitled for each dependent, and the employees take-home pay. The program output includes state tax withheld, federal tax withheld, dependent tax deductions, salary, and take-home pay.
Instructions
-
Ensure the file named Payroll.java is open.
-
Variables have been declared and initialized for you as needed, the output statements have been written and the input object has been declared and initialized for you. Read the code carefully before you proceed to the next step.
-
Write the Java code needed to perform the following:
- Calculate state withholding tax (stateTax) at 6.5 percent
- Calculate federal withholding tax (federalTax) at 28.0 percent.
- Calculate dependent deductions (dependentDeduction) at 2.5 percent of the employees salary for each dependent.
-
- Calculate total withholding (totalWithholding) as stateTax + federalTax + dependentDeduction.
- Calculate take-home pay (takeHomePay) as salary minus total withholding.
- Compile and execute your program by clicking the Run button. You should get the following output:
State Tax: $81.25 Federal Tax: $350.00000000000006 Dependents: $62.5 Salary: $1250 Take-Home Pay: $756.25
- In this program, the variables salary and numDependents are initialized with the values 1250.00 and 2. To make this program more flexible, modify it to accept interactive input for salary and numDependents.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
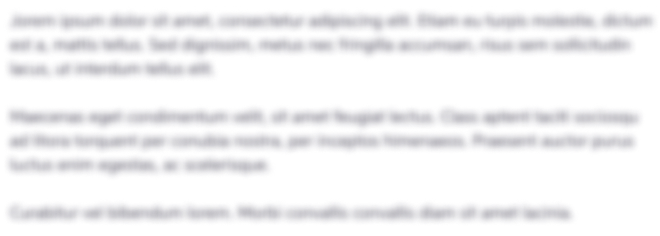
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started