Question
Unit 7 Assignment Grading Information: This Program is due on Date Specified. Comments are REQUIRED; flow charts and pseudocode are NOT REQUIRED. Directions Points The
Unit 7 Assignment Grading Information: This Program is due on Date Specified. Comments are REQUIRED; flow charts and pseudocode are NOT REQUIRED. Directions Points The files must be called (driver) (handles house variables and methods) Proper coding conventions required the first letter of the class start with a capital letter and the first letter of each additional word start with a capital letter. Only submit the .java files needed to make the program run. Do not submit the .class file or any other file. 5% Style Components Include properly formatted prologue, comments, indenting, and other style elements as shown in Chapter 2 starting page 64 and Appendix 5 page 881-892. 5% Topics covered in chapter Topics with * are covered in this assignment. Ensure you use every item listed below with an * in your completed assignment. *Relationships between reference variables and objects Reference assignment Memory space Equality of Objects Swap data in objects *Method call chaining *Object creating and initialization Calling one constructor from inside another Class variable usage Class methods Class constants Basic Requirements Write a driver and house class that gets input and using method call chaining creates 2 houses, imports the data from a text file, and outputs the results. Refer to chapter 3 on how to read in a file. LiFiUnit7.java Provide a driver class that demonstrates this house class. You the code below as the contents of you main method. Ensure you use the code provided exactly as given 20% inside your main method except for renaming your House class. Scanner stdIn = new Scanner(System.in); Scanner stdInFile = new Scanner(new File("LiFiUnit7HouseData.txt")); LiFiUnit7House_SU_2016 house1, house2; //New houses //Create house 1 using default constructor house1 = new LiFiUnit7House_SU_2016(); house1.print(); //print house 1 with default values String street, city, state, zipCode; int number; System.out.println("Importing Number."); number = stdInFile.nextInt(); stdInFile.nextLine(); System.out.println("Importing Street."); street = stdInFile.nextLine(); System.out.println("Importing City."); city = stdInFile.nextLine(); System.out.println("Importing State."); state = stdInFile.nextLine(); System.out.println("Importing ZipCode."); zipCode = stdInFile.nextLine(); System.out.println(); //use method call chaining to set values //and print results for house 1 house1.setNumber(number).setStreet(street) .setCity(city).setState(state) .setZipCode(zipCode).print(); System.out.println("Importing Number."); number = stdInFile.nextInt(); stdInFile.nextLine(); System.out.println("Importing Street."); street = stdInFile.nextLine(); System.out.println("Importing City."); city = stdInFile.nextLine(); System.out.println("Importing State."); state = stdInFile.nextLine(); System.out.println("Importing ZipCode."); zipCode = stdInFile.nextLine(); System.out.println(); //create house 2 using 5 parameter constructor house2 = new LiFiUnit7House_SU_2016(number, street, city, state, zipCode); //print house 2 house2.print(); This demonstration driver does not call all accessor and mutator methods but it is normal to create them regardless of an immediate use. They may be needed in the future. Sample output is provided below. Be sure to mimic it exactly except for values entered. LiFiUnit7House.java Write a House class called LiFiUnit7House.java that implements the following methods. setNumber receives the house number setStreet receives the street name setCity receives the city name setState receives the state name setZipCode receives the zip code Separate accessor methods for each instance variable utilized. 30% Method Call Chaining Pay attention to implement method call chaining as prescribed in the main method given. This is the main part of the program for this week. 40% NOTE: Complete your activity and submit it by clicking Submit Assignment Total Percentage 100% Data for Text File (name it LiFiUnit7House.txt) 8700 NW River Park Dr. Parkville MO 64152 1600 Pennsylvania Ave NW Washington DC 20500 Sample Your output for House 1 and House 2 should match the sample below. House Information Number: 0 Street: No Street City: No City State: No State Zip: No Zip Code Importing Number. Importing Street. Importing City. Importing State. Importing ZipCode. House Information Number: 8700 Street: NW River Park Dr. City: Parkville State: MO Zip: 64152 Importing Number. Importing Street. Importing City. Importing State. Importing ZipCode. House Information Number: 1600 Street: Pennsylvania Ave NW City: Washington State: DC Zip: 20500 Expert Answer Anonymous Anonymous answered this Was this answer helpful? 0 0 2,484 answers import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; /** * @author * */ public class LiFiUnit7 { /** * @param args * @throws FileNotFoundException */ public static void main(String[] args) throws FileNotFoundException { // TODO Auto-generated method stub Scanner stdIn = new Scanner(System.in); Scanner stdInFile = new Scanner(new File("LiFiUnit7HouseData.txt")); LiFiUnit7House house1, house2; // New houses // Create house 1 using default constructor house1 = new LiFiUnit7House(); house1.print(); // print house 1 with // default values String street, city, state, zipCode; int number; System.out.println("Importing Number."); number = stdInFile.nextInt(); stdInFile.nextLine(); System.out.println("Importing Street."); street = stdInFile.nextLine(); System.out.println("Importing City."); city = stdInFile.nextLine(); System.out.println("Importing State."); state = stdInFile.nextLine(); System.out.println("Importing ZipCode."); zipCode = stdInFile.nextLine(); System.out.println(); // use method call chaining to set values //and print results for house house1.setNumber(number).setStreet(street).setCity(city) .setState(state).setZipCode(zipCode).print(); System.out.println("Importing Number."); number = stdInFile.nextInt(); stdInFile.nextLine(); System.out.println("Importing Street."); street = stdInFile.nextLine(); System.out.println("Importing City."); city = stdInFile.nextLine(); System.out.println("Importing State."); state = stdInFile.nextLine(); System.out.println("Importing ZipCode."); zipCode = stdInFile.nextLine(); System.out.println(); // create house 2 using 5 parameter constructor house2 = new LiFiUnit7House(number, street, city, state, zipCode); // print house 2 house2.print(); } } /** * */ /** * @author * */ public class LiFiUnit7House { String street, city, state, zipCode; int number; /** * parameterized constructor * * @param street * @param city * @param state * @param zipCode * @param number */ public LiFiUnit7House(int number, String street, String city, String state, String zipCode) { this.street = street; this.city = city; this.state = state; this.zipCode = zipCode; this.number = number; } /** * default constructor */ public LiFiUnit7House() { this.street = ""; this.city = ""; this.state = ""; this.zipCode = ""; this.number = 0; } /** * @return the street */ public String getStreet() { return street; } /** * @return the city */ public String getCity() { return city; } /** * @return the state */ public String getState() { return state; } /** * @return the zipCode */ public String getZipCode() { return zipCode; } /** * @return the number */ public int getNumber() { return number; } /** * @param street * the street to set */ public LiFiUnit7House setStreet(String street) { this.street = street; return this; } /** * @param city * the city to set */ public LiFiUnit7House setCity(String city) { this.city = city; return this; } /** * @param state * the state to set */ public LiFiUnit7House setState(String state) { this.state = state; return this; } /** * @param zipCode * the zipCode to set */ public LiFiUnit7House setZipCode(String zipCode) { this.zipCode = zipCode; return this; } /** * @param number * the number to set */ public LiFiUnit7House setNumber(int number) { this.number = number; return this; } public void print() { System.out.println(this); } /* * (non-Javadoc) * * @see java.lang.Object#toString() */ @Override public String toString() { return " Street=" + street + " City=" + city + " State=" + state + " ZipCode=" + zipCode + " Number=" + number; } } LiFiUnit7HouseData.txt 8700 NW River Park Dr. Parkville MO 64152 1600 Pennsylvania Ave NW Washington DC 20500 OUTPUT: Street= City= State= ZipCode= Number=0 Importing Number. Importing Street. Importing City. Importing State. Importing ZipCode. Street=NW River Park Dr. City=Parkville State=MO ZipCode=64152 Number=8700 Importing Number. Importing Street. Importing City. Importing State. Importing ZipCode. Street=Pennsylvania Ave NW City=Washington State=DC ZipCode=20500 Number=1600 I got this answer from here but its giving me this error idk whats going on. This code is giving me errors that says this idk whats going on. Exception in thread "main" java.util.InputMismatchException at java.base/java.util.Scanner.throwFor(Scanner.java:860) at java.base/java.util.Scanner.next(Scanner.java:1497) at java.base/java.util.Scanner.nextInt(Scanner.java:2161) at java.base/java.util.Scanner.nextInt(Scanner.java:2115) at MJUnit7.main(MJUnit7.java:30) can somebody help me fix this. Thanks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
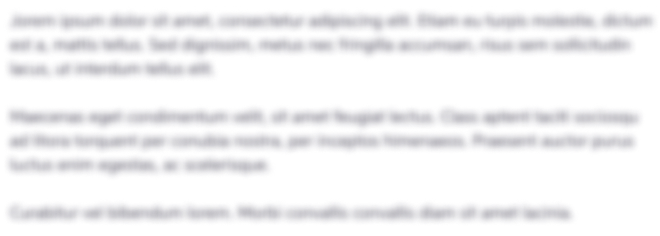
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started