Question
University (with Lambdas) Working with Polymorphic Collections This is a long, complex lab that will require you to use some very new techniques. It is
University (with Lambdas) Working with Polymorphic Collections
This is a long, complex lab that will require you to use some very new techniques. It is best started early.
Instructions:
- Create a class called Person with the following instance variables:
- The Person class should have getters and setters for all properties other than id. (Use Eclipse to help you auto-generate these.)
- Person class should also have a getter for id.
- IDs are assigned sequentially. The last ID used was 916421998. (Think carefully how you will manage this fact.)
Instance Variable Data Type id long firstName String middleName String lastName String emai String ssn String age int - In addition to these getters and setters, Person should have the following instance methods:
- toString() returns full name, like "Edgar Allen Poe" or "Sally Strickler" (if there is no middle name)
- getEmailDomain() returns the domain portion of an email (afterthe @), as in "gmai.com"
- getLast4SSN() returns the last four digits of the Social Security Number
- Add some validation to age, email and ssn. You may have done this via the setters in the past. However, this time, let's add class (static) methods to validate these fields as best you can. Give some thought to what advantages this approach would have. Consider using regular expressions, but maybe you could also be more helpful with specific feedback, for instance "The SSN needs to contain two hyphens."
- Eventually, you will want to make a polymorphic collection of Persons and loop through the collection. Depending on the run, you will want to evaluate which person is the oldest or which person is the youngest. (Perhaps you may want to evaluate Persons based on something unanticipated as well!) Since we do not know exactly how we want to evaluate a Person, we could use lambda expressions. This is the beauty of lambdas. The execution of the functional interface can vary from run to run. Therefore, you will want to create a Predicate to test whether the Person is the oldest, and a Predicate to test whether the Person is the youngest. Since we can anticipate some ways that somebody might want to evaluate a Person, we can store these ways in a public static Map in the Person class. The key should be a String, and the value should be a Predicate of Persons. The first entry in this HashMap should have the key "oldest" and, for its value, a Predicate that evaluates whether the person is the oldest in the collection. Similarly, the second entry in the HashMap should have the key of "youngest" and a corresponding Predicate You might not yet have learned how to create a public static HashMap that can be modified. The below example can help you. We can initialize a HashMap using a static block of code:
public static HashMap
When you run the program, you will prompt the user for how they want to evaluate -- by the youngest or the oldest. Using the static HashMap described here, you will be able to get the appropriate lambda expression to store in the instance variable evaluator based on user input. Give some thought as to the most appropriate way to know the oldest and the youngest people as they are being added to the collection. Each new entry in the collection could become either the youngest or the oldest person so far. EXTRA CREDIT: While the Person class has two ways to evaluate Persons, your client class (the Driver) might think of a different way, for instance whether the Person is in their 20s. See if you can create a Predicate for something new on the fly in the Driver, add it to the static HashMap, and use it when displaying your Persons.articles; static { articles = new HashMap<>(); articles.put("ar01", "Intro to Map"); articles.put("ar02", "Some article"); } - Create subclasses for Instructor and Student
- Instructors should have a Department
- Students should have a Major
- Generate getters and setters.
- Create a driver program named Driver:
- The main method, like all good main methods, should be brief. So you will use private static methods to organize your code.
- Early in your main method, you will want to create a Scanner to read user input: Scanner sc = new Scanner(System.in);
- When you are done with the scanner in main(), you should close it: sc.close();
- As discussed above, your main method should prompt the user to enter how they would like to evaluate your Persons. Something like: System.out.println("How should we evaluate?"); evaluatorType = sc.nextLine(); The answer will determine which Predicate you will use when initiating your Person objects.
- In the Driver, you are going to make a polymorphic HashMap of Persons. The key of this HashMap will be the person's id, and the value will be the Person itself.
- You should create three private static methods to make a Person, make an Instructor, and make a Student respectively. You will call these from the main() method. The method to make a Person object will prompt the user for the correct values and thus the Scanner object needs to be passed to it as a parameter. The methods to create the Student and the Instructor will just hardcode the creation of these instances. The Person, the Student, and the Instructor will all be added to your HashMap of Persons.
- When you are prompting for information about the Person, please adhere to the following validation rules
- Accept any value for the name fields
- Age must be an integer greater than 16
- Email addresses and Social Security Numbers should be validated using regular expressions
- After the collection is built, the Driver (in a separate private static method) should loop through the HashMap and display the following information:
- The person's id
- The person's full name and what kind of Person are they
- The person's email domain
- The last 4 digits of the person's Social Security Number
- How the person is evaluated, e.g., whether the person is the oldest in the collection
- The major for the Student, the department for the Instructor
Here is some sample output, to help you understand the end goal of the exercise
How should we evaluate? [Enter 'oldest' or 'youngest'] oldest Enter person's first name Rip Enter person's middle name Van Enter person's last name Winkle Enter person's email address rip@rowan.edu Enter person's SSN in ###-##-#### format 111-22-3333 Enter person's age 99
916421999 Rip Van Winkle (Person) rowan.edu 3333 oldest: true
916422000 Jane Marie Doe (Student) students.rowan.edu 4444 oldest: false Computer Science
916422001 Chia C. Chien (Instructor) rowan.edu 5555 oldest: false Math/Science
Here is another run, this time evaluating for the youngest.
How should we evaluate? [Enter 'oldest' or 'youngest'] youngest Enter person's first name Baby Enter person's middle name
Enter person's last name Yoda Enter person's email address babyoda@arvala7.com Enter person's SSN in ###-##-#### format 222-33-4444 Enter person's age 2 Enter person's age 17
916421999 Baby Yoda (Person) arvala7.com 4444 youngest: true
916422000 Jane Marie Doe (Student) students.rowan.edu 4444 youngest: false Computer Science
916422001 Chia C. Chien (Instructor) rowan.edu 5555 youngest: false Math/Science
Step by Step Solution
There are 3 Steps involved in it
Step: 1
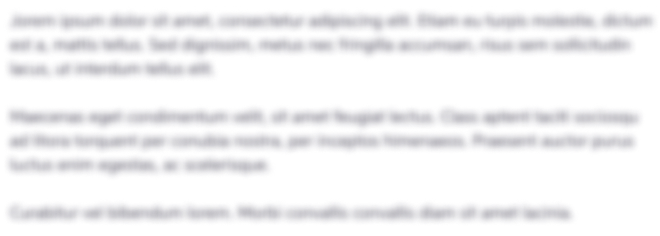
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started