Question
Unix Questions: 1) Congratulations, you have been offered an internship position by the Galactic Empire! You will be working with the Death Star team as
Unix Questions:
1) Congratulations, you have been offered an internship position by the Galactic Empire! You will be working with the Death Star team as a test engineer, making sure that the Empire's proudest piece of machinery is fully operational so that the Emperor does not become the laughingstock of those Rebel Scum. Knowing that the `DeathStar` class has 4 constructors and 84 public methods, in general, how many units are there to be tested?
2) One of the methods you are testing is for the Death Star to make hologram calls. The method will take in a contact number as parameter, and returns the `ConnectionStream` object if the call is connected and `null` if the call never was picked up. Complete the following test:
public void testMakeHologramCall() { // construct a new Death Star DeathStar ds = new DeathStar();
// call Darth Vader String contact = "1 (202) 358-0001";
// this method should return null since Darth Vader never picks up calls ConnectionStream stream = ds.makeHologramCall(contact);
// make assertion and fill in the blank (in the *BRACKETS* below) _________________________
}
``` What goes in the underlined space?
3)
Another method, `jumpToLightSpeed`, takes in a coordinate as the parameter for the destination, and throws a `HyperDriveOfflineException` if the hyperdrive appears to be offline.
public void testJumpToLightSpeed(Coordinate destination) {
// construct a new Death Star DeathStar ds = new DeathStar();
// turn off hyperdrive ds.hyperDrive.off();
// destination: Planet Alderaan Coordinate alderaan = LocationUtil.getCoordinate("Alderaan");
// trying to jump to light speed should throw an exception try { ds.jumpToLightSpeed(alderaan);
// FIXME: POSITION A
} catch (HyperDriveOfflineException e) { System.err.println(e);
// FIXME: POSITION B
} finally { // FIXME: POSITION C } } ``` Choose the best place(s) to add the `fail()` statement where it says FIXME (i.e. 1, 2, or 3)
4) Now you're looking to save some time when coordinating the next raid on a Rebel base. To do this, you want to create a Makefile to automatically update the Galactic Fleet. In general, what is the default target inside a Makefile?
5) Assuming that you want the Makefile to recompile the test only if `DeathStar.java` or `DeathStarTester.java` have been updated since the last time it was compiled. what goes to the dependency list below?
DeathStarTester.class: __________________________ javac DeathStarTester.java ``` What goes in the underlined space above?
6) Explain what the following lines do in a Makefile:
.SUFFIXES: .java .class .java.class: javac $<
7) Your impressive work at your internship has won you a return offer at the Galactic Empire. You were but the student; now you are the master! You are responsible for all Imperial Vehicles's software reliability. The vehicle classes include: * ATAT.java * ATST.java * DeathStar.java * ImperialShuttle.java * StarDestroyer.java * TIEBomber.java * TIEFighter.java For each of these classes, there is a Tester class that contains all unit tests for the corrsponding vehicle named as `(Vehicle)Tester.java`, where (Vehicle) can be replaced by any of the vehicle class name. Write a Makefile that does the following: a. When type in `make (Vehicle).class`, the corresponding vehicle class is built, if and only if its Java source file has been updated since the last time it was built. b. When type in `make` or `make vehicles`, each vehicle class will be built (ATAT.class, ATST.class, etc.) if and only if the corresponding Java source file has been updated since the last time that class was built. c. When type in `make (Vehicle)Tester.class`, the corresponding tester class is built, if and only if (Vehicle).java or (Vehicle)Tester.java has been updated since the last time the tester was built. d. When type in `make tests`, all tester classes will be built (ATATTester.class, ATSTTester.class, etc.), if and only if the corresponding Java source file for that tester or the tested vehicle has been updated since the last time the tester was built. e. When type in `make clean`, all class files will be removed. Hints: You are encouraged to use variables to organize your code and increase readability. And feel free to create dummy classes for testing purposes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
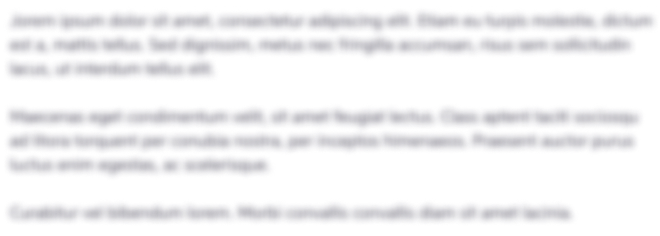
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started