Question
UnreliableCar File: unreliable_car.py and unreliable_car_test.py Let's make our own derived class for an UnreliableCar that inherits from Car. UnreliableCar has an additional attribute: reliability: a
UnreliableCar
File: unreliable_car.py and unreliable_car_test.py
Let's make our own derived class for an UnreliableCar that inherits from Car.
UnreliableCar has an additional attribute:
reliability: a float between 0 and 100, that represents the percentage chance that the drive method will actually drive the car
UnreliableCar should override the following methods:
__init__(self, name, fuel, reliability)
call the Car's version of __init__, and then set the reliability to the value passed in
drive(self, distance)
generate a random number between 0 and 100, and only drive the car if that number is less than the car's reliability. Don't store the random number! It's not a self/instance variable that you want to remember, it's a temporary value you generate and use once, every time you call drive().
Super Important Note: the drive method in the base class Car always returns the distance driven, so your derived class UnreliableCar must also return a distance. You must match the "signature" of any method you override. This is true, even if your UnreliableCar drives 0 km.
""" CP1404/CP5632 Practical Car class """ from prac_09.car import Car class Taxi(Car): """Specialised version of a Car that includes fare costs.""" def __init__(self, name, fuel, price_per_km): """Initialise a Taxi instance, based on parent class Car.""" super().__init__(name, fuel) self.price_per_km = price_per_km self.current_fare_distance = 0 def __str__(self): """Return a string like a Car but with current fare distance.""" return f"{super().__str__()}, {self.current_fare_distance}km on current fare, ${self.price_per_km:.2f}/km" def get_fare(self): """Return the price for the taxi trip.""" return self.price_per_km * self.current_fare_distance def start_fare(self): """Begin a new fare.""" self.current_fare_distance = 0 def drive(self, distance): """Drive like parent Car but calculate fare distance as well.""" distance_driven = super().drive(distance) self.current_fare_distance += distance_driven return distance_driven
test.py
my_taxi = Taxi("Prius 1", 100, 1.23) my_taxi.drive(40) print(my_taxi) print("Current fare: ${:.2f}".format(my_taxi.get_fare())) my_taxi.start_fare() my_taxi.drive(100) print(my_taxi) print("Current fare: ${:.2f}".format(my_taxi.get_fare()))
python
Step by Step Solution
There are 3 Steps involved in it
Step: 1
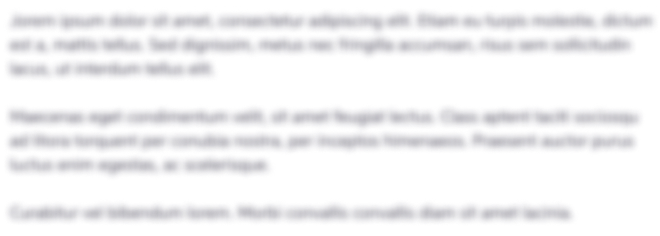
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started