Question
USE C++ Step 1: Overload the less than operator ( = 0 && position < maxSize); list[position] = item; length++; } #endif ***rectangleType2.h file*** #ifndef
USE C++
Step 1: Overload the less than operator (<) for the rectangleType class
- The less than operator is used by the sort() method of the listType class
- Since the less than operator is used in a method of the template class, it needs to be overloaded in the rectangleType class
- Define less than to be the area of the first object less than the area of the second object
Step 2: Write a main program to include the following
- Create a listType object. You must supply rectangleType as the class parameter for the listType class template. Use a parameter of 5 to create the listType object
- Create 5 rectangleType objects providing value for the length and width of each object
- Insert the 5 rectangleType objects into the listType object
- Print the listType object
- Sort the listType object
- Print the listType object again after the sort
INCLUDED FILES BELOW
***listType.h file***
#ifndef H_listType #define H_listType #include
template
bool isFull() const; //Function to determine whether the list is full. //Postcondition: Returns true if the list is full, // otherwise it returns false. int getLength() const; //Function to return the number of elements in the list. //Postcondition: The value of length is returned.
int getMaxSize() const; //Function to return the maximum number of elements //that can be stored in the list. //Postcondition: The value of maxSize is returned.
void sort(); //Function to sort the list. //Postcondition: The list elements are in ascending order.
void print() const; //Outputs the elements of the list.
void insertAt(const elemType& item, int position); //Function to insert item in the list at the location //specified by position. //Postcondition: list[position] = item; length++; // If position is out of range, the program // is aborted.
listType(int listSize = 50); //Constructor //Creates an array of the size specified by the //parameter listSize; the default array size is 50. //Postcondition: list contains the base address of the // array; length = 0; maxsize = listSize;
~listType(); //Destructor //Deletes all the elements of the list. //Postcondition: The array list is deleted.
private: int maxSize; //variable to store the maximum size //of the list int length; //variable to store the number of elements //in the list elemType *list; //pointer to the array that holds the //list elements };
template
template
template
template
//Constructor; the default array size is 50 template
template
template
for (i = 0; i < length; i++) { min = i; for (j = i + 1; j < length; ++j) if (list[j] < list[min]) min = j;
temp = list[i]; list[i] = list[min]; list[min] = temp; }//end for }//end sort
template
template
#endif
***rectangleType2.h file***
#ifndef H_rectangleType #define H_rectangleType #include
class rectangleType { public: void setDimension(double l, double w); double getLength() const; double getWidth() const; double area() const; double perimeter() const; void print() const;
friend ostream& operator << (ostream&, const rectangleType &); rectangleType operator+(const rectangleType&) const; //Overload the operator + rectangleType operator*(const rectangleType&) const; //Overload the operator *
bool operator==(const rectangleType&) const; //Overload the operator == bool operator!=(const rectangleType&) const; //Overload the operator !=
rectangleType(); rectangleType(double l, double w);
private: double length; double width; };
#endif
***rectangleTypeImp2.cpp file***
#ifndef H_rectangleType #define H_rectangleType #include
class rectangleType { public: void setDimension(double l, double w); double getLength() const; double getWidth() const; double area() const; double perimeter() const; void print() const;
friend ostream& operator << (ostream&, const rectangleType &); rectangleType operator+(const rectangleType&) const; //Overload the operator + rectangleType operator*(const rectangleType&) const; //Overload the operator *
bool operator==(const rectangleType&) const; //Overload the operator == bool operator!=(const rectangleType&) const; //Overload the operator !=
rectangleType(); rectangleType(double l, double w);
private: double length; double width; };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
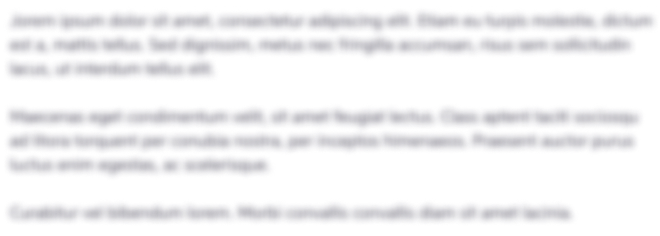
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started