Question
Use Console I/O (Scanners and System.out) for all input/output in this lab. Do not hard code data file paths or filenames; always ask the user
Use Console I/O (Scanners and System.out) for all input/output in this lab.
Do not hard code data file paths or filenames; always ask the user for them.
For this lab you will create a MonsterAttack class that represents one monster attack, an AttackMonitor class that aggregates data about attacks, and an MonsterAttackDriver class that instantiates an AttackMonitor. One of the key functions of AttackMonitor is to read and write data using a text file, but you will complete this part in a future lab. Note that nearly all the methods in AttackMonitor and MonsterAttack will be instance, not static, methods.
Part I - MonsterAttack class
Each object of this class represents information about a single attack. The data on each attack includes an integer attack id, three ints representing the day of the month, the month, and the year of the attack, the name of the monster involved (assume that each attack involves only one monster), the location of the attack (String), and the name of the person reporting the attack (String).
MonsterAttack needs a constructor that sets all the instance fields listed above using its parameters. It should take the three components of the date as a single String in the format MM/DD/YYYY (eg, 01/31/2010). Use String's split method to split this String on the slashes, parse the resulting Strings to ints, and use them to set the values of the month, day, and year.
Monster attack also needs getters and setters for all the data fields and a toString() that uses the instance data to create and return a String similar to this: "Attack # 125: Godzilla attacked Tokyo on 6/25/2009. Reported by Kyohei Yamane."
"(Add a method to AttackMonitor that saves the list of attacks to a comma separated values file. Iterate through the list, and for each attack, get each field using the getters from MonsterAttack. Write each value to the file, following each one except the last with a comma. Save the date as a single String in the format MM/DD/YYYY. After you have written out all the data for one attack, write out a newline. Add an item to the main menu that calls this method. )"
""Add a method that clears the list of monster attacks, then uses a Scanner to read data from a .csv file, uses it to instantiate MonsterAttack objects, and adds the attacks to the list. This method must be able to read the files you write out in the method described above. You will need to use String's split() method here. Add an item to the main menu that calls this method. Make sure you can input attack data, save to a file, quit the program, start the program again, read your output file, and show the data from the file. ""
Part II - AttackMonitor
Create an AttackMonitor class that keeps track of the MonsterAttack objects. It should contain an array list (not an array) of MonsterAttacks and an instance method called monitor() with a menu that allows the user to choose to quit or to do any of the tasks listed below, which must all be implemented in separate methods. After doing any of these tasks, the program should return to the menu, so that the user can continue working on any series of tasks until he/she chooses to quit. Write instance, not static, methods to do each of the following:
Input information on a MonsterAttack. Get the data from the user, call the MonsterAttack constructor using the input data as arguments, and add the new attack to the list of attacks.
Show the current list of attacks by iterating through the list, calling the toString() of each attack, and outputting the result. Write this method so that the program does not crash if the list is empty.
Choose an attack from the array list and delete it. The easiest way to do this is to call the method that shows the information, then ask the user for the id number of the attack to delete. Use linear search to find the attack with that id and delete it. The id number may not be the same as the index in the list. Make sure the application does not crash if the user enters an id that does not appear in the list.
AttackMonitor must also have a method to create a MonsterAttack using method parameters and add it to the list. In other words, this method will take parameters for the data fields, rather than taking them as user input. You will call this method from the Driver to create test data without needing to type it.
Part III: MonsterAttackDriver
The driver class should instantiate an AttackMonitor and call its monitor() method.
In order to make the application easier to test, the driver should provide hard coded data on three attacks. Use the data to create the three attacks and add them to the list in the monitor. Use a separate method for this and call it from main().
Turn in a working executable .jar file and your .java code files
Step by Step Solution
There are 3 Steps involved in it
Step: 1
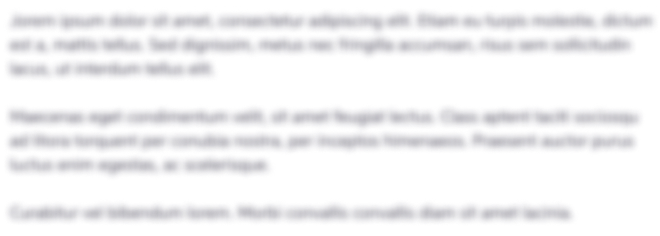
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started