Question
* use data structure and object oriented programming language to solve the followin you are required to do the following : Add the following nonmember
*use data structure and object oriented programming language to solve the followin
you are required to do the following :
Add the following nonmember functions A nonmember function that read the Linked List info from a text file (info.txt) A nonmember function that write the linked List info to a new text file (new.txt) SeparateList: this function takes a linkedListType of students and two ArrayListType of students; if the student GPA>3 add it to List1 objects otherwise add it to List2, use the following prototype: void SeparateList (LinkedListType * obj, ArrayListType&List1, ArrayListType&List1);
* if you have a 1) class Student and 2) linkedListType.h and 3) ArrayListType.h
suppose that class student has a private attribute (double gpa ) and has setters and getters and whatever ....
suppose the ArrayListType has the following (suppose you knoe the implementation )
class ArrayListType { template
public: // Constructor ArrayListType(int size=10); ArrayListType(const ArrayListType& obj); const ArrayListType& operator=(const ArrayListType &obj); bool isEmpty() const; bool isFull() const; int listSize() const; int maxListSize() const; void print() const; bool isItemAtEqual(int location, const Type& item) const; void insertAt(int location, const Type& insertItem); void insertEnd(const Type& insertItem); void removeAt(int location); void replaceAt(int location, const Type& repItem); void retrieveAt(int location, Type& retItem) const; void clearList(); int seqSearch(const Type& item) const; void insert(const Type& insertItem); void remove(const Type& removeItem); int& operator[](int index); const int& operator[](int index) const;
private: int length; int maxSize; Type *List;
};
linkedListType.h
#include using namespace std;
#ifndef LINKEDLISTTYPE_H_INCLUDED #define LINKEDLISTTYPE_H_INCLUDED //Definition of the node template struct nodeType { Type info; nodeType *link; };
template class linkedListType { public: void initializeList(); bool isEmptyList() const; void print() const; int length() const; void destroyList(); Type front() const; Type back() const; bool search(const Type& searchItem) const; void insertFirst(const Type& newItem); void insertLast(const Type& newItem); void deleteNode(const Type& deleteItem); nodeType* begin(); nodeType* end(); linkedListType(); ~linkedListType(); linkedListType(const linkedListType& otherList); const linkedListType& operator= (const linkedListType&);//no
protected: int count; nodeType *first; nodeType *last; private: void copyList(const linkedListType& otherList); };
template bool linkedListType::isEmptyList() const { return (first == NULL); }
template linkedListType::linkedListType() //default constructor { first = NULL; last = NULL; count = 0; }
template void linkedListType::destroyList() { nodeType *temp; //pointer to deallocate the memory //occupied by the node { temp = first; //set temp to the current node first = first->link; //advance first to the next node delete temp; //deallocate the memory occupied by temp } last = NULL; //initialize last to NULL; first has already //been set to NULL by the while loop count = 0; }
template void linkedListType::initializeList() { destroyList(); //if the list has any nodes, delete them }
template void linkedListType::print() const { nodeType *current; //pointer to traverse the list current = first; //set current so that it points to //the first node while (current != NULL) //while more data to print { cout << current->info << " "; current = current->link; } }//end print
template int linkedListType::length() const { return count; }
template Type linkedListType::front() const { assert(first != NULL); return first->info; //return the info of the first node }//end front
template Type linkedListType::back() const { assert(last != NULL); return last->info; //return the info of the last node }//end back
template nodeType* linkedListType::begin() { return first; }
template nodeType* linkedListType::end() { return last; }
template void linkedListType::copyList (const linkedListType& otherList) { nodeType *newNode; //pointer to create a node nodeType *current; //pointer to traverse the list if (first != NULL) //if the list is nonempty, make it empty destroyList(); if (otherList.first == NULL) //otherList is empty { first = NULL; last = NULL; count = 0; } else { current = otherList.first; //current points to the //list to be copied count = otherList.count; //copy the first node first = new nodeType; //create the node first->info = current->info; //copy the info first->link = NULL; //set the link field of //the node to NULL last = first; //make last point to the //first node current = current->link; //make current point to //the next node //copy the remaining list while (current != NULL) { newNode = new nodeType; //create a node newNode->info = current->info; //copy the info newNode->link = NULL; //set the link of //newNode to NULL last->link = newNode; //attach newNode after last last = newNode; //make last point to //the actual last node current = current->link; //make current point //to the next node }//end while }//end else }//end copyList
template linkedListType::~linkedListType() //destructor { destroyList(); }
template linkedListType::linkedListType (const linkedListType& otherList) { first = NULL; copyList(otherList); }//end copy constructor
template const linkedListType& linkedListType::operator= (const linkedListType& otherList) { if (this != &otherList) //avoid self-copy { copyList(otherList); }//end else return *this; }
template bool linkedListType:: search(const Type& searchItem) const { nodeType *current; //pointer to traverse the list bool found = false; current = first; //set current to point to the first //node in the list while (current != NULL && !found) //search the list if (current->info == searchItem) //searchItem is found found = true; else current = current->link; //make current point to //the next node return found; }//end search
template void linkedListType::insertFirst(const Type& newItem) { nodeType *newNode; //pointer to create the new node newNode = new nodeType; //create the new node newNode->info = newItem; //store the new item in the node newNode->link = first; //insert newNode before first first = newNode; //make first point to the //actual first node count++; //increment count if (last == NULL) //if the list was empty, newNode is also //the last node in the list last = newNode; }//end insertFirst
template void linkedListType::insertLast(const Type& newItem) { nodeType *newNode; //pointer to create the new node newNode = new nodeType; //create the new node newNode->info = newItem; //store the new item in the node newNode->link = NULL; //set the link field of newNode //to NULL if (first == NULL) //if the list is empty, newNode is //both the first and last node { first = newNode; last = newNode; count++; //increment count } else //the list is not empty, insert newNode after last { last->link = newNode; //insert newNode after last last = newNode; //make last point to the actual //last node in the list count++; //increment count } }//end insertLast
template void linkedListType::deleteNode(const Type& deleteItem) { nodeType *current; //pointer to traverse the list nodeType *trailCurrent; //pointer just before current bool found; if (first == NULL) //Case 1; the list is empty cout << "Cannot delete from an empty list." << endl; else { if (first->info == deleteItem) //Case 2 { current = first; first = first->link; count--; if (first == NULL) //the list has only one node last = NULL; delete current; } else //search the list for the node with the given info { found = false; trailCurrent = first; //set trailCurrent to point //to the first node current = first->link; //set current to point to //the second node while (current != NULL && !found) { if (current->info != deleteItem) { trailCurrent = current; current = current-> link; } else found = true; }//end while
if (found) //Case 3; if found, delete the node { trailCurrent->link = current->link; count--; if (last == current) //node to be deleted //was the last node last = trailCurrent; //update the value //of last delete current; //delete the node from the list } else cout << "The item to be deleted is not in " << "the list." << endl; }//end else }//end else }//end deleteNode #endif // LINKEDLISTTYPE_H_INCLUDED
Step by Step Solution
There are 3 Steps involved in it
Step: 1
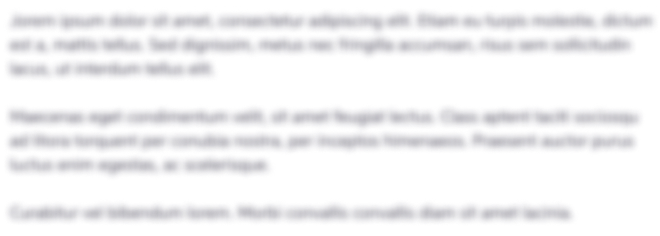
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started