Question
use inheritance with the following 2 classes, create a 3rd class called three dimensional shape and adapt the provided main _________________ #include #include using namespace
use inheritance with the following 2 classes, create a 3rd class called "three dimensional shape" and adapt the provided main
_________________
#include
#include
using namespace::std;
class Shape
{
protected:
string geometricFigureName;
string color;
public:
Shape();
Shape(string aGeometricFigureName, string aColor);
~Shape();
void setGeometricFigureName(string aGeometricFigureName);
void setColor(string aColor);
string getGeometricFigureName() const;
string getColor() const;
friend istream &operator >> (istream &, Shape &obj);
friend ostream &operator << (ostream &, Shape &obj);
};
____________________shape functions
#include "class 1.h"
Shape::Shape()
{
setGeometricFigureName("");
setColor("");
}
Shape::Shape(string aGometricFigureName, string aColor)
{
setGeometricFigureName(aGometricFigureName);
setColor(aColor);
}
Shape::~Shape()
{
cout << " This is shape destructor " << endl;
cout << endl;
}
void Shape::setColor(string aColor) { color = aColor; }
void Shape::setGeometricFigureName(string aGometricFigureName)
{
geometricFigureName = aGometricFigureName;
}
string Shape::getColor() const { return color; }
string Shape::getGeometricFigureName() const { return geometricFigureName; }
ostream &operator << (ostream &output, Shape &obj)
{
output << "Geometric Figure Name:" << obj.getGeometricFigureName() << endl;
output << "Color:" << obj.getColor() << endl;
return output;
}
_____________two dimensional shape class
#include
using namespace std;
#include "fun1.h"
class TwoDimensionalShape : public Shape
{
protected:
double area;
double perimeter;
public:
TwoDimensionalShape();
TwoDimensionalShape(double anArea, double aPerimeter);
TwoDimensionalShape(double anArea, double aPerimeter, string aGometricFigureName, string aColor);
~TwoDimensionalShape();
void setArea(double);
void setPerimeter(double);
double getArea() const;
double getPerimeter() const;
friend ostream &operator << (ostream& output, TwoDimensionalShape &obj);
};
____________________two dimensional shape efunctions
#include
using namespace::std;
#include "class2.h"
TwoDimensionalShape::TwoDimensionalShape() : Shape()
{
setArea(0.0);
setPerimeter(0.0);
setColor("");
setGeometricFigureName("");
}
TwoDimensionalShape::TwoDimensionalShape(double anArea, double aPerimeter)
{ setArea(anArea); setPerimeter(aPerimeter);
}
TwoDimensionalShape::TwoDimensionalShape(double anArea, double aPerimeter, string aGometricFigureName, string anColor): Shape(aGometricFigureName, anColor)
{ setArea(anArea); setPerimeter(aPerimeter);
}
TwoDimensionalShape::~TwoDimensionalShape() {
cout << "This is destructor de TwoDimensional" << endl;
cout << endl; }
void TwoDimensionalShape::setArea(double anArea) { area = anArea; }
void TwoDimensionalShape::setPerimeter(double aPerimeter) { perimeter = aPerimeter; }
double TwoDimensionalShape::getArea() const { return area; }
double TwoDimensionalShape::getPerimeter() const { return perimeter; }
ostream &operator << (ostream &output, TwoDimensionalShape &obj)
{
output << "Area:" << obj.getArea() << endl;
output << "Perimeter:" << obj.getPerimeter() << endl;
return output;
}
______________main
#include "fun3.h"
int main()
{
Shape shape1;
Shape *ShapePtr = nullptr;
TwoDimensionalShape TwoDimensionalShape1;
TwoDimensionalShape *TwoDimensionalShapePtr = nullptr;
Circle Circle1(5.7,6,7,"Circulo","Azul");
Circle Circle2(10, 6, 7);
//Circle1.display();
cout << Circle1;
cout << endl;
//Circle2.display();
cout << Circle2;
cout << endl;
ShapePtr = &Circle1;
//ShapePtr->display();
cout << *ShapePtr;
cout << endl;
TwoDimensionalShapePtr = &Circle1;
//TwoDimensionalShapePtr->display();
cout << *TwoDimensionalShapePtr;
cout << endl;
ShapePtr = &Circle2;
// ShapePtr->display();
cout << ShapePtr;
cout << endl;
TwoDimensionalShapePtr = &Circle2;
//TwoDimensionalShapePtr->display();
cout << *TwoDimensionalShapePtr;
cout << endl;
return (0);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
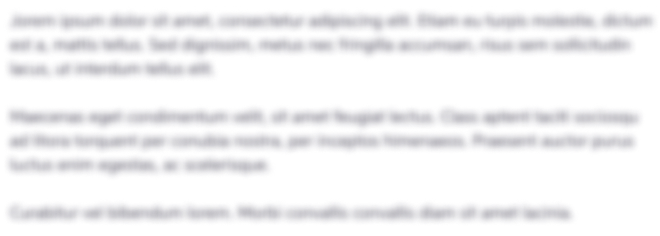
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started