Answered step by step
Verified Expert Solution
Question
1 Approved Answer
use it if you need public class Patient implements Comparable { //attributes private int order; private String name; private boolean emergencyCase; //constructor public Patient(int order,
use it if you need
public class Patient implements Comparable
//attributes private int order; private String name; private boolean emergencyCase; //constructor public Patient(int order, String name, boolean emergencyCase) { this.order = order; this.name = name; this.emergencyCase = emergencyCase; } //compareTo public int compareTo(Patient other) { if(this.isEmergencyCase() && !other.isEmergencyCase()) return -1; //place this first else if(!this.isEmergencyCase() && other.isEmergencyCase()) return 1; //place other first else //if both are emergency or both are not emergency return this.getOrder()-other.getOrder(); //place smaller order first } //getters and setters public int getOrder() { return order; } public void setOrder(int order) { this.order = order; } public String getName() { return name; } public void setName(String name) { this.name = name; } public boolean isEmergencyCase() { return emergencyCase; } public void setEmergencyCase(boolean emergencyCase) { this.emergencyCase = emergencyCase; } public String toString() { return (isEmergencyCase()?"*":"") + name; } }
import java.util.Comparator; public class PatientComparator implements Comparator{ public int compare(Patient p1, Patient p2) { if(p1.isEmergencyCase() && !p2.isEmergencyCase()) return -1; //place p1 first else if(!p1.isEmergencyCase() && p2.isEmergencyCase()) return 1; //place p2 first else //if both are emergency or both are not emergency return p1.getOrder()-p2.getOrder(); //place smaller order first } }
import java.util.ArrayList; public class PatientTestQ12 { public static void main(String[] args) { ArrayListQ3. [15 marksJ Write a program that obtains the execution time of the three sort algorithms used in the above Sorter class (i e., bubble sort, selection sort, and insertion sort). Your program should print out the time required to sort array lists of N patients, where N ranges from 5,000 to 50,000 with an increment of 5,000 (see sample run below). Every time increment N, your program should recreate unsorted array lists of N random patients and then sort them. A random patient should have a random id between 0 and N and random Most questions are based on taken from the exercisesand examples listed in the textbook used by the course. emergency case (true or false for emergencyCase). Don't worry much about creating a random name for each patient. Instead, use the name "anonymous" (or any other name of your choice) for all patients In order to properly compare the performance of the three sorting algorithms in Sorter, you need to have them work on three identical array lists. Start by creating an array list with random patients, then clone twice. Finally, sort each array list (original and clones) with a different algorithm and print the sorting time. Repeat this process for different values of N When you submit your code, you need submit two screen shots of the output of two runs of your code (similar to the ones given below)list = new ArrayList(5); list.add(new Patient(1, "p1", false)); list.add(new Patient(2, "p2", false)); list.add(new Patient(3, "p3", true)); list.add(new Patient(4, "p4", false)); list.add(new Patient(5, "p5", true)); //before sorting System.out.printf("%-15s%25s ", "Before sorting", list); //should be [p1, p2, p3, p4, p5] //try bubble sort methods for Q1 //Sorter.bubbleSort(list); //Sorter.bubbleSort(list, new PatientComparator()); //other sort methods for Q2 //Sorter.selectionSort(list); //Sorter.insertionSort(list); //after sorting System.out.printf("%-15s%25s ", "After sorting", list); //should be [p3, p5, p1, p2, p4] } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
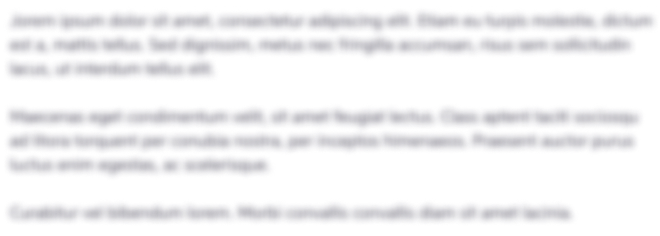
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started