Question
use Java in running and writing the codes thanks. Exercise 3 [10 Points]: Examine the Employee class (provided with this Lab). Finish the compareTo() method
use Java in running and writing the codes thanks.
Exercise 3 [10 Points]:
Examine the Employee class (provided with this Lab). Finish the compareTo() method using all three of the instance variables. Access them using the getX() methods. Compare two employees by first looking at their last names. If the names are different, return the value the String method compareTo() returns with the last names. If the names are equal, look at the first name and return the value the String method compareTo() returns. If both parts of the name are equal, return the difference of the birthYears so that the older employee precedes the younger employee.
Test your implementation using this class: EmployeeTester.java (also provided in the Lab folder). Do not modify this class.
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * * @author mrahman */
public class Employee implements Comparable
String getFirstName() { return firstName; } String getLastName() { return lastName; } int getBirthYear() { return birthYear; } public Employee( String f, String l, int year ) { firstName = f; lastName = l; birthYear = year; } public String toString(){ //finish this } public int compareTo( Employee other ) { //finish this } }
import java.util.Arrays;
class EmployeeTester { public static void main ( String[] args ) { Employee[] workers = new Employee[12]; workers[0] = new Employee( "Fred", "Adams", 1963); workers[1] = new Employee( "John", "Adams", 1959); workers[2] = new Employee( "Elmer", "Adams", 1976); workers[3] = new Employee( "Nancy", "Devon", 1963); workers[4] = new Employee( "Andrew", "Lewis", 1983); workers[5] = new Employee( "Douglas", "Page", 1981); workers[6] = new Employee( "Donald", "Wolder", 1963); workers[7] = new Employee( "Henry", "Wolder", 1972); workers[8] = new Employee( "Robert", "Wolder", 1959); workers[9] = new Employee( "Howard", "Cohen", 1933); workers[10] = new Employee( "Howard", "Cohen", 1958); workers[11] = new Employee( "Donald", "Rice", 1935); Arrays.sort( workers ); for ( int j=0; j } Exercise 4 [10 Points]: Say that you are writing a computer game that involves a hero who encounters various monsters. Monsters have several characteristics: hit points, strength, age, and name (the class Monster.java is supplied). The number of hit points a monster has is how far it is from death. A monster with a large number of hit points is hard to kill. The hero also has hit points. When the hit points of the hero reach zero, the game is over. The strength of a monster affects how many hit points the hero looses when the monster hits the hero. The age and name of the monster do not affect the outcome of battles between the monster and a hero. Can you make the necessary changes to the Monster class (supplied with this lab) and write a tester (client) class with main method that will present the hero with monsters in increasing order of difficulty. Hints: Monster needs a compareTo() method. Save your program in the MonsterClient.java file. /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * Say that you are writing a computer game that involves a hero who encounters * various monsters. Monsters have several characteristics: hit points, strength, * age, and name. The number of hit points a monster has is how far it is from * death. A monster with a large number of hit points is hard to kill. The hero * also has hit points. * When the hit points of the hero reach zero, the game is over. The strength of * a monster affects how many hit points the hero looses when the monster hits * the hero. The age and name of the monster do not affect the outcome of battles * between the monster and a hero. * * Can you make the necessary changes to the Monster class and write a tester * class with main method that will present the hero with monsters in * increasing order of difficulty. * Hints: Monster needs a compareTo() method. */ public class Monster { private int hitPoints, strength, age; private String name; public Monster( int hp, int str, int age, String nm ) { hitPoints = hp; strength = str; this.age = age; name = nm; } public int getHitPoints() { return hitPoints; } public int getStrength() { return strength; } public int getAge() { return age; } public String getName() { return name; } @Override public String toString() { return "HP: " + getHitPoints() + " \tStr: " + getStrength() + "\t" + getName(); } } Exercise 5 [10 Points]: In this exercise, you need to write a static search method that will take an array of Comparable objects and a Comparable key as parameter and it returns true if the key is present in the array, otherwise it should return false. Test your implementation with two different type of Comparable objects. One possible idea to test your code is to create two arrays, one for Monster (from exercise 4) and another for Employee (from exercise 3), then pass each one to the search method for testing. Save this file as SearchClient.java. Note: You should submit client codes (where necessary). Code should be properly commented.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
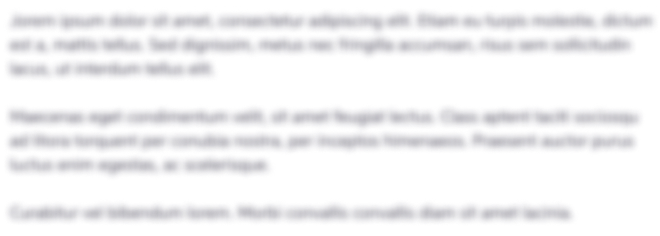
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started