Question
Use Python 2.7 In this programming task, you will implement a TCP client-server program in Python. Your client will send an encrypted message to the
Use Python 2.7
In this programming task, you will implement a TCP client-server program in Python. Your client will send an encrypted message to the server and the server should display the decrypted message. Use Caesar cipher for encryption and decryption at the client and server, respectively.
Your client program should encrypt the following input message using the provided key, print the encrypted message, and send the encrypted message to the server. For the received message, your server should decrypt it using the same key and print the decrypted message. Input message: I have built my life around you but time makes you bolder even children get older and I am getting older too Key: 13
Problem # 2 [5 Points] In this programming task, you will implement a TCP client-server program in Python. Your client will send an encrypted message to the server and the server should display the decrypted message. Use SDES (Simplified DES) cipher for encryption and decryption at the client and server, respectively.
Your client program should encrypt the following input message using the provided key and number of rounds, print the encrypted message, and send the encrypted message to the server. For the received message, your server should decrypt it using the same key and number of rounds, and print the decrypted message. Refer to the explanation provided in the Appendix below.
Input message: I have built my life around you but time makes you bolder even children get older and I am getting older too
Key: 101101001
Number of SDES rounds/iterations: 2
Hint: SDES operates only on binary input, so you need to convert the input message to binary before encryption and back to ASCII from binary after decryption.
Sample code snippet for binary-ASCII conversion:
import binascii Conversion to binary from ASCII
binary_input=bin(int(binascii.hexlify(input_message),16))
binary_input = binary_input [:1] + binary_input [2:]
Conversion to ASCII from binary
binary_output = int(binary_output,2)
output_message = binascii.unhexlify('%x' % binary_output)
Problem # 3 (Extra Credit) [5 Points] In this programming task, you will write a Python program to decrypt (crack) the encrypted input without prior knowledge of the encryption key.
a. The following text is encrypted using Caeser cipher. Your program should crack it, and print the decrypted message and the associated key.
Lbh ner havdhr va gur jbeyq n sevraq yvxr ab bgure Vg vf fb nznmvat gung jr unir fb zhpu va pbzzba fb lbhe unccvarff vf nyfb zvar
b. The following binary string is encrypted using SDES cipher. Your program should decrypt (crack) it, and print the decrypted message and the associated key.
011001100110100101101110011010010111001101101000001000000110011001101001011100 100111001101110100
Appendix: Simplified DES
Simplified DES is a bock cipher.
Data Block Size: In Simplified DES, encryption/decryption is done on data blocks of 12 bits. The plaintext is divided into blocks of 12 bits and the algorithm is applied to each block.
Key: The key size is 9 bits. The round key is 8 bits. The first 8 bits of K is shifted with 1 bit clockwise for every round.
Example: If K = 111000111
Then K1 = 11100011 and K3 = 10001111
Algorithm:
The block of 12 bits is written in the form L0R0, where L0 consists of the first 6 bits and R0 consists of the last 6 bits. The i th round of the algorithm transforms an input Li-1Ri-1 to the output LiRi using an 8-bit round key Ki derived from K. One Round of a SDES The Function f (Ri-1, Ki) The output for the i th round is found as follows. Li = Ri-1 and Ri = Li-1 f (Ri-1, Ki)
This operation is performed for a certain number of rounds, say n, and produces LnRn. The ciphertext will be RnLn. Encryption and decryption are done the same way except the keys are selected in the reverse order. The keys for encryption will be K1, K2 Knand for decryption will be Kn, Kn-1 K1.
Function f(Ri-1,Ki): - The function f(Ri-1,Ki), depicted in the Figure below, is described in following steps.
1. The 6-bits are expanded using the following expansion function. The expansion function takes 6-bit input and produces an 8-bit output. This output is the input for the two S-boxes. 2. The Expansion Function, E(Ri-1) 2. The 8-bit output from the previous step is Exclusive-ORed ( ) with the round key Ki
3. The 8-bit output is divided into two blocks. The first block consists of the first 4 bits and the last four bits make the second block. The first block is the input for the first S-box (S1) and the second block is the input for the second S-box (S2).
4. The S-boxes take 4 bits as input and produce 3bits of output. The first bit of the input is used to select the row from the S-box, 0 for the first row and 1 for the second row. The last 3 bits are used to select the column. Example: Let the output from the expander function be 11010010. So 1101 will be the input for the S1 box and 0010 will be the input for the S2 box. The output from the S1 box will be 111, the first bit of the input is 1 so select the second row and 101 will select the 6th column. Similarly the output from the S2 box will be 110.
5. The output from the S-boxes is combined to form a single block of 6 bits. These 6 bits will be the output of the functionf(Ri-1,Ki). In our example we have the S1 output 111 and S2 output 110. So the output for the function f(Ri-1,Ki) will be 111110, the S1 output followed by the S2 output. The S1 and S2 boxes are shown below. S1-Box S2-Box 101 010 001 110 011 100 111 000 001 100 110 010 000 111 101 011 100 000 110 101 111 001 011 010 101 011 000 111 110 010 001 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
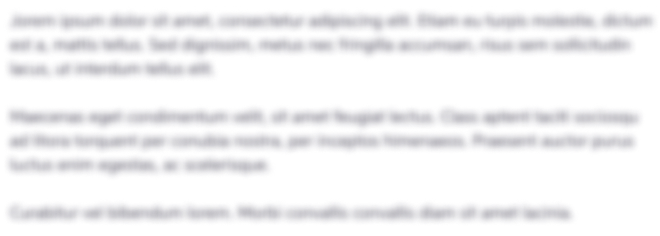
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started