Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Use Python for the following problem in the picture provided and provide comments # This class represents a single node in the linked list class
Use Python for the following problem in the picture provided and provide comments # This class represents a single node in the linked list class Node: # Constructor def initself data, nextnode: # Each Node object keeps track of two things: the data it's holding, # and a reference to the next node self.data data self.nextnode nextnode # This class represents a linked list class LinkedList: # Constructor def initself: # The list just needs to keep track of its head node. From there, we # can get to anywhere else in the list! self.head None # The size attribute keeps track of how many nodes are in the list. We # need to update this whenever nodes are added or removed. self.size # str method def strself: # This string stores the result to return result 'head # Traverse the list, starting from the head temp self.head while temp None: # Add the current node's data to the result result ftempdata # Advance temp to the next node in the list temp temp.nextnode result 'None' return result # Returns the element at a certain nonnegative index of the linked list def getself index: # Make sure that the index is valid if index self.size : # Traverse the list to get to that index temp self.head for i in rangeindex: temp temp.nextnode # Return the data stored inside that node return temp.data else: raise IndexErrorfInvalid index provided, index must be between and selfsize # Replaces the element at a certain nonnegative index with a new value def setself index, newdata: # Make sure that the index is valid if index self.size : # Traverse the list to get to that index temp self.head for i in rangeindex: temp temp.nextnode # Update the data of that node with newdata temp.data newdata else: raise IndexErrorfInvalid index provided, index must be between and selfsize # Adds newdata to the head of the list def addtoheadself newdata: # Create a new node that contains newdata. The new node's next points # to the current head of the list. newnode Nodenewdata, self.head # Update the list's head reference to point to the new node self.head newnode # We could combine both actions above into a single line: # self.head Nodenewdata, self.head # Update the size attribute of the linked list self.size # Adds newdata at the specified index of the list def addself newdata, index: # If index is call the previously written addtohead method if index : self.addtoheadnewdata # Make sure that the index is valid. Valid indices do include the # size of the list, since that would be adding a new node at the end of # the list. elif index self.size: # Get to the insertion point of the new node temp self.head for i in rangeindex : temp temp.nextnode # Create the new node, and change the list references to insert # that node newnode Nodenewdata, temp.nextnode temp.nextnode newnode # We could combine both actions above into a single line: # temp.nextnode Nodenewdata, temp.nextnode # Update the size attribute of the linked list self.size else: raise IndexErrorfInvalid index provided, index must be between and selfsize # Deletes the head node from the list, and returns the data that was deleted def deletefromheadself: # Make sure the list isn't empty if self.head None: temp self.head.data self.head self.head.nextnode self.size return temp else: # Handle this however desired probably should raise some kind of # exception pass
Use Python for the following problem in the picture provided and provide comments
# This class represents a single node in the linked list
class Node:
# Constructor
def initself data, nextnode:
# Each Node object keeps track of two things: the data it's holding,
# and a reference to the next node
self.data data
self.nextnode nextnode
# This class represents a linked list
class LinkedList:
# Constructor
def initself:
# The list just needs to keep track of its head node. From there, we
# can get to anywhere else in the list!
self.head None
# The size attribute keeps track of how many nodes are in the list. We
# need to update this whenever nodes are added or removed.
self.size
# str method
def strself:
# This string stores the result to return
result 'head
# Traverse the list, starting from the head
temp self.head
while temp None:
# Add the current node's data to the result
result ftempdata
# Advance temp to the next node in the list
temp temp.nextnode
result 'None'
return result
# Returns the element at a certain nonnegative index of the linked list
def getself index:
# Make sure that the index is valid
if index self.size :
# Traverse the list to get to that index
temp self.head
for i in rangeindex:
temp temp.nextnode
# Return the data stored inside that node
return temp.data
else:
raise IndexErrorfInvalid index provided, index must be between and selfsize
# Replaces the element at a certain nonnegative index with a new value
def setself index, newdata:
# Make sure that the index is valid
if index self.size :
# Traverse the list to get to that index
temp self.head
for i in rangeindex:
temp temp.nextnode
# Update the data of that node with newdata
temp.data newdata
else:
raise IndexErrorfInvalid index provided, index must be between and selfsize
# Adds newdata to the head of the list
def addtoheadself newdata:
# Create a new node that contains newdata. The new node's next points
# to the current head of the list.
newnode Nodenewdata, self.head
# Update the list's head reference to point to the new node
self.head newnode
# We could combine both actions above into a single line:
# self.head Nodenewdata, self.head
# Update the size attribute of the linked list
self.size
# Adds newdata at the specified index of the list
def addself newdata, index:
# If index is call the previously written addtohead method
if index :
self.addtoheadnewdata
# Make sure that the index is valid. Valid indices do include the
# size of the list, since that would be adding a new node at the end of
# the list.
elif index self.size:
# Get to the insertion point of the new node
temp self.head
for i in rangeindex :
temp temp.nextnode
# Create the new node, and change the list references to insert
# that node
newnode Nodenewdata, temp.nextnode
temp.nextnode newnode
# We could combine both actions above into a single line:
# temp.nextnode Nodenewdata, temp.nextnode
# Update the size attribute of the linked list
self.size
else:
raise IndexErrorfInvalid index provided, index must be between and selfsize
# Deletes the head node from the list, and returns the data that was deleted
def deletefromheadself:
# Make sure the list isn't empty
if self.head None:
temp self.head.data
self.head self.head.nextnode
self.size
return temp
else:
# Handle this however desired probably should raise some kind of
# exception
pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
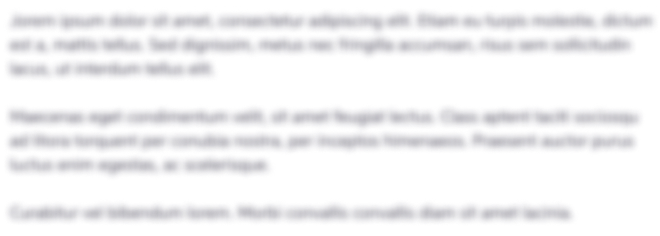
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started