Question
Use python Part 2 Calculate the moving average. (40 points) Start a new program temp_win2.py from your working version of temp_win1.py. Since you know that
Use python
Part 2 Calculate the moving average. (40 points)
Start a new program temp_win2.py from your working version of temp_win1.py.
Since you know that you are correctly getting the file name and the window parameter from the user, you can now comment out the lines that print:
Analyzing NorCal-1880-2018.csv
Using 60 for the window parameter.
Before calculating the moving average you will need to read the temperature data into a list so your program can read the same value over and over when sliding the window for the moving average. Change the loop in your program that reads the year and temperature data from the file into two lists: a list holding the years and a list holding the floating point temperatures. These lists will make it possible to repeatedly access the data when computing the average.
Moving average calculation
The moving average at position i in the list is calculated as the sum of the temperature values from position i-k to position i+k divided by the total width of the window (2*k + 1). Below is an example of the calculation for k = 5 and i = 5.
The boxed below illustrate a window at position i = 5 with width k = 5 in the temps list:
sliding window: | i-5 | i-4 | i-3 | i-2 | i-1 | i | i+1 | i+2 | i+3 | i+4 | i+5 |
temps values: | 0.59 | 0.41 | 0.34 | -0.22 | 1.03 | 0.23 | -0.06 | -0.40 | 0.48 | 2.63 | 0.18 |
temps indices: | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
ave = (temps[i-k] + ...temps[i-1] + temps[i] + temps[i+1]... + temps[i+k])/(2*k+1)
year = years[i]
Your calculation will not look exactly like this. You will need to implement the sum using a nested loop or using the sum() function on a slice of the list.
Also note is that this calculation is not valid near the ends of the list. You should write a loop that only calculates the average for valid years. In other words if temps[i-k] can not have an index of less than 0, then i cannot be less than k. In the example above i cannot be less than 5. A similar constraint holds for the other end of the list.
Write a loop to compute the average temperature for each valid year. The window position i will move, or slide, from the lowest valid year to the highest valid year. Your loop should print the year and the average separated by a comma. Format the printed temperature to four decimal places using the format method.
Here is an example of the output your program should produce when the user chooses to average over k=60 years; there are not too many rows since there are only 10 years in the middle of the century when there are 60 years before and 60 years after over which to average.
Temperature anomaly filename:NorCal-1880-2018.csv Enter an integer between 0 and 60:60 1940,-0.2331 1941,-0.2169 1942,-0.2150 1943,-0.2228 1944,-0.2107 1945,-0.1796 1946,-0.1667 1947,-0.1582 1948,-0.1585 1949,-0.1492 1950,-0.1711 1951,-0.1688 1952,-0.1490 1953,-0.1556 1954,-0.1548 1955,-0.1580 1956,-0.1420 1957,-0.1101 1958,-0.1017
temp_1 file and read_file is based on:
while True: try: in_name = input("Temperature anomaly filename:") fhand = open(in_name) break except: print("Could not open",in_name) print("Analyzing", in_name)
for i in range(5): fhand.readline()
for line in fhand: line = line.strip() (year, temp) = line.split(",") year = int(year) temp = float(temp)
done=False while not done: x=input("Enter an integer between 0 and 60:") if not x.isdigit(): print("Enter an integer.") else: k=int(x) if k<1 or k>60: print("Enter an integer up to 60.") else: done=True print("Using {0} for the window parameter.".format(k))
the database is :
38.5 deg N, 121.7 deg E Temperature Anomalies, July Units: Degrees Celsius Base Period: 1981-2010 Missing: -9999 Year,Value 1880,-1.56 1881,-0.08 1882,-0.30 1883,-1.44 1884,-2.29 1885,-1.95 1886,-0.63 1887,0.63 1888,-0.72 1889,2.99 1890,-0.50 1891,-1.36 1892,1.03 1893,-0.16 1894,-0.01 1895,-1.46 1896,-1.23 1897,-0.83 1898,-0.91 1899,1.53 1900,1.08 1901,0.24 1902,-1.08 1903,-1.10 1904,-0.83 1905,-0.31 1906,-0.22 1907,-1.02 1908,-1.05 1909,-0.65 1910,-0.65 1911,-1.06 1912,-0.65 1913,-2.32 1914,1.12 1915,-0.21 1916,-0.57 1917,0.41 1918,-0.13 1919,0.48 1920,0.67 1921,0.21 1922,0.65 1923,-0.60 1924,-1.00 1925,-1.22 1926,-1.10 1927,-0.03 1928,0.61 1929,0.54 1930,0.66 1931,-1.57 1932,0.15 1933,0.83 1934,-0.35 1935,0.23 1936,-0.66 1937,0.17 1938,0.56 1939,0.31 1940,0.09 1941,-0.60 1942,-0.51 1943,-0.86 1944,-0.09 1945,-1.19 1946,0.77 1947,-0.72 1948,-0.33 1949,-0.53 1950,0.08 1951,0.73 1952,-0.31 1953,0.26 1954,-1.90 1955,-0.72 1956,-0.95 1957,-1.12 1958,1.28 1959,1.29 1960,0.28 1961,2.33 1962,0.32 1963,-0.25 1964,-0.13 1965,-0.80 1966,0.10 1967,1.23 1968,-0.06 1969,-1.19 1970,-1.51 1971,0.28 1972,-0.15 1973,1.32 1974,-0.72 1975,0.49 1976,-1.43 1977,-1.51 1978,1.61 1979,-0.74 1980,-1.41 1981,-0.09 1982,-0.33 1983,-0.88 1984,-0.06 1985,0.57 1986,-0.83 1987,-0.77 1988,-0.21 1989,-0.13 1990,-0.54 1991,-0.10 1992,-0.21 1993,-1.80 1994,1.47 1995,-0.08 1996,-1.28 1997,2.20 1998,0.49 1999,-0.37 2000,0.73 2001,0.40 2002,0.15 2003,-1.25 2004,0.03 2005,1.47 2006,-0.39 2007,0.40 2008,0.59 2009,0.41 2010,0.34 2011,-0.22 2012,1.03 2013,0.23 2014,-0.06 2015,-0.40 2016,0.48 2017,2.63 2018,0.18
Step by Step Solution
There are 3 Steps involved in it
Step: 1
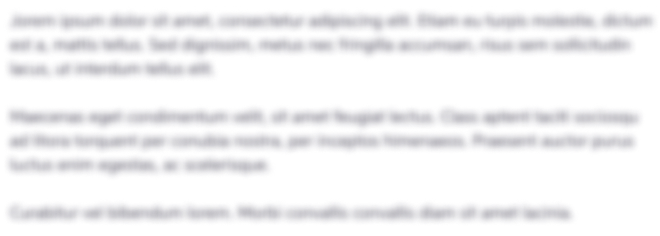
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started