Question
use python: singly linked list class 1. Re-implement the remove method so that it works correctly in the case where the item is not in
use python: singly linked list class
1. Re-implement the remove method so that it works correctly in the case where the item is not in the list
Code
class Node: def __init__(self,initdata): self.data = initdata self.next = None
def getData(self): return self.data
def getNext(self): return self.next
def setData(self,newdata): self.data = newdata
def setNext(self,newnext): self.next = newnext
class UnorderedList:
def __init__(self): self.head = None
def isEmpty(self): return self.head == None
def add(self,item): temp = Node(item) temp.setNext(self.head) self.head = temp
def size(self): current = self.head count = 0 while current != None: count = count + 1 current = current.getNext()
return count
def search(self,item): current = self.head found = False while current != None and not found: if current.getData() == item: found = True else: current = current.getNext()
return found
def remove(self,item): current = self.head previous = None found = False while not found: if current.getData() == item: found = True else: previous = current current = current.getNext()
if previous == None: self.head = current.getNext() else: previous.setNext(current.getNext())
Step by Step Solution
There are 3 Steps involved in it
Step: 1
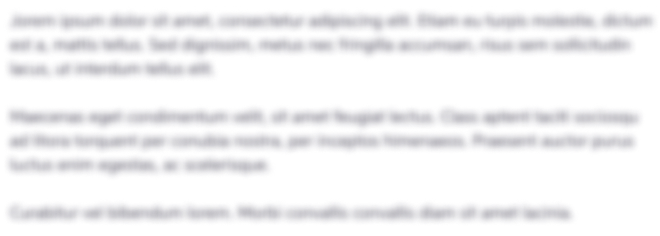
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started