Question
USE PYTHON3; DO NOT IMPORT ANY PACKAGES I only need all of part d (core functionality) , which has three sections(can_handle, can_handle_task_sequence, and handle_two_tasks). Please
USE PYTHON3; DO NOT IMPORT ANY PACKAGES
I only need all of part d (core functionality), which has three sections(can_handle, can_handle_task_sequence, and handle_two_tasks). Please do all three sections. I just put parts a through c to make sure that you had all the necessary info. Also make sure to pay attention to the requirements of assert statements in part d. Thank you!
Instance attributes of PersonalSchedule class:
last_name (str): Given by the constructor's argument. After initialization, last_name indicates the last name of the student.
first_name (str): Given by the constructors argument. After initialization, first_name indicates the first name of the student.
avaliable_time (list of list of ints): Given by the constructors argument. After initialization, available_time indicates the available time slot for a person to complete a task. It has the following structure: [[start_time1, end_time1, focus_level1], [start_time2, end_time2, focus_level2], ...]
What the code looks like in the editor:
def can_handle(self, task): """ This function determines whether this person can handle the given task. Requirement: Input validation Parameters: task (Task): A task that this person needs to handle. Returns: True if there exists a time interval in the schedule that can properly handle the task with the required focus level, False otherwise. """ # YOUR CODE STARTS HERE #
def can_handle_task_sequence(self, task_sequence): """ Given a list of tasks, this function determines whether this person can handle this task sequence. Requirement: Input validation Parameters: task_sequence (list[Task]): A list of tasks this person needs to handle. Returns: True if all tasks can be properly handled, False otherwise. To simplify the question, we assume that multitasking is possible, i.e., a person can handle multiple tasks in a single time interval. """ # YOUR CODE STARTS HERE #
def handle_two_tasks(self, task1, task2): """ Given two tasks, the function determines whether this personal can handle them together. Requirement: Input validation Parameters: task1 (Task): The first task. task2 (Task): The second task. Returns: True if two tasks can be merged and handled by this PersonalSchedule, False otherwise. """ # YOUR CODE STARTS HERE #
A) Constructor (_init_): last_name (str): Last name of student. This string must have at least 2 characters but not necessarily capitalized. first_name (str): First name of student. This string must have at least 2 characters but not necessarily capitalized. available_time: list of lists of ints. Note: Assert statements are not required for constructors/getters/ string representations. You can assume that PersonalSchedule objects are always initialized correctly. We assume that there is no overlapping in the available_time intervals B) Getters: a) get_first_name(self) Getter method that returns the first_name. This method creates the data abstraction. Example: return Marina if the PersonalSchedule's first_name is Marina. b) get_last_name(self) Getter method that returns the last_name. This method creates the data abstraction. Example: return Langlois if the PersonalSchedule's last_name is Langlois. c) get_available_time(self) Getter method that returns the available_time. This method creates the data abstraction. Example: return [[1, 10, 20], [12, 19, 30], [20, 29, 8]] if available_time is [[1, 10, 20), (12, 19, 30], [20, 29, 8]] Note: All these getter methods require one line. C) String Representation Method a) __str__(self) In order to print a string representation of an object we need to write a special method (will be covered in class later). This method enables us to use print statements in the class. The special method __str_) is given to you in the starter code. It uses the getter methods that you have developed earlier. D)Core Functionality a) can_handle(self, task) Checks if a person can handle a given task. We say that a person can handle a given task if: There is such an interval in avaliable_time where a person can deal with the given task focus_level(during the interval) >= focus_level_required for that task. Requirement: assert statements Parameters: task is the Task object Returns: True if the task can be handled successfully, False otherwise. Example: available_time is [[1, 3, 20], [4, 6, 30], [9, 12, 8]] task is Task(4, 5, 32, "Classification") The function will return false, because focus_level is not greater than focus_level_required for all appropriate intervals. You can also refer to the last page of the writeup to get a better understanding of whether the task can be handled. b) can_handle_task_sequence (self, task_sequence): This function is similar to the function above. Only this time you are given a list of tasks to check if they can be handled. Requirement: assert statements Parameters: task_sequence (a list of tasks): all the tasks that person wants to handle. Each task is an instance of class Task. Returns: True if all tasks in the list can be handled, False otherwise. Note: You can assume that each interval in available_time can handle more than one task. You can view more examples at the end of the write up. c) handle_two_tasks(self, taski, task2) This function returns True only under the condition that - The two tasks can be merged The merged task can be handled by the PersonalSchedule Otherwise, it will return false Requirement: assert statements Parameters taski (Task): the first task to handle task2 (Task): the second task to handle Returns: handle_two_tasks returns True if the two tasks can be merged, and the PersonalSchedule can handle the merged task. Example: taski is (2, 5, 20, "Chopping potatoes") task1 is (4, 6, 20, "Chopping potatoes") avaliable_time = [[1, 10, 39], [14, 23, 100]] The function will return True since those two tasks can be merged, and it can be handled in the avaliable_time. taski is (2, 15, 20, "Chopping potatoes") taski is (10, 200, 20, "Chopping potatoes") avaliable_time is [[1, 19, 39],[14, 23, 100]] The function will return false since after merging those two tasks, the start_time = 2, and end_time = 200, and this huge task cannot be handled by the PersonalSchedule any more. A) Constructor (_init_): last_name (str): Last name of student. This string must have at least 2 characters but not necessarily capitalized. first_name (str): First name of student. This string must have at least 2 characters but not necessarily capitalized. available_time: list of lists of ints. Note: Assert statements are not required for constructors/getters/ string representations. You can assume that PersonalSchedule objects are always initialized correctly. We assume that there is no overlapping in the available_time intervals B) Getters: a) get_first_name(self) Getter method that returns the first_name. This method creates the data abstraction. Example: return Marina if the PersonalSchedule's first_name is Marina. b) get_last_name(self) Getter method that returns the last_name. This method creates the data abstraction. Example: return Langlois if the PersonalSchedule's last_name is Langlois. c) get_available_time(self) Getter method that returns the available_time. This method creates the data abstraction. Example: return [[1, 10, 20], [12, 19, 30], [20, 29, 8]] if available_time is [[1, 10, 20), (12, 19, 30], [20, 29, 8]] Note: All these getter methods require one line. C) String Representation Method a) __str__(self) In order to print a string representation of an object we need to write a special method (will be covered in class later). This method enables us to use print statements in the class. The special method __str_) is given to you in the starter code. It uses the getter methods that you have developed earlier. D)Core Functionality a) can_handle(self, task) Checks if a person can handle a given task. We say that a person can handle a given task if: There is such an interval in avaliable_time where a person can deal with the given task focus_level(during the interval) >= focus_level_required for that task. Requirement: assert statements Parameters: task is the Task object Returns: True if the task can be handled successfully, False otherwise. Example: available_time is [[1, 3, 20], [4, 6, 30], [9, 12, 8]] task is Task(4, 5, 32, "Classification") The function will return false, because focus_level is not greater than focus_level_required for all appropriate intervals. You can also refer to the last page of the writeup to get a better understanding of whether the task can be handled. b) can_handle_task_sequence (self, task_sequence): This function is similar to the function above. Only this time you are given a list of tasks to check if they can be handled. Requirement: assert statements Parameters: task_sequence (a list of tasks): all the tasks that person wants to handle. Each task is an instance of class Task. Returns: True if all tasks in the list can be handled, False otherwise. Note: You can assume that each interval in available_time can handle more than one task. You can view more examples at the end of the write up. c) handle_two_tasks(self, taski, task2) This function returns True only under the condition that - The two tasks can be merged The merged task can be handled by the PersonalSchedule Otherwise, it will return false Requirement: assert statements Parameters taski (Task): the first task to handle task2 (Task): the second task to handle Returns: handle_two_tasks returns True if the two tasks can be merged, and the PersonalSchedule can handle the merged task. Example: taski is (2, 5, 20, "Chopping potatoes") task1 is (4, 6, 20, "Chopping potatoes") avaliable_time = [[1, 10, 39], [14, 23, 100]] The function will return True since those two tasks can be merged, and it can be handled in the avaliable_time. taski is (2, 15, 20, "Chopping potatoes") taski is (10, 200, 20, "Chopping potatoes") avaliable_time is [[1, 19, 39],[14, 23, 100]] The function will return false since after merging those two tasks, the start_time = 2, and end_time = 200, and this huge task cannot be handled by the PersonalSchedule any moreStep by Step Solution
There are 3 Steps involved in it
Step: 1
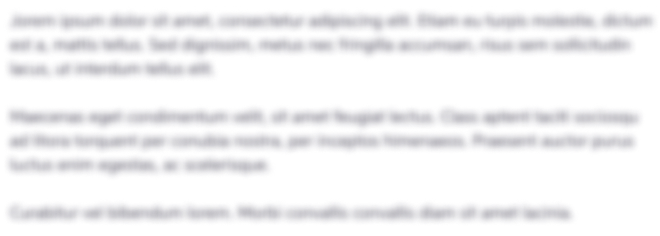
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started