Question
USE PYTHON3; DO NOT IMPORT ANY PACKAGES Please follow the directions carefully and pay attention to the bolded directions as well. Thank you! 1. We
USE PYTHON3; DO NOT IMPORT ANY PACKAGES
Please follow the directions carefully and pay attention to the bolded directions as well. Thank you!
1. We will learn space efficiency while creating our code. Solve the following with their respective rules.
a)
Write a function that will reverse the last half of the list parameter WITHOUT creating a new list. That is, reverse all the items in the list starting from the middle (len//2) within the list itself. (in place.) Do not use lst[::-1] to do it (or a similar approach). Think of a way to manipulate a given list without creating another list. You should not have a return statement, because your function will mutate a given list.
def reverse_half(lst):
"""
Given input list lst, reverse the last half of the list,
starting from the middle (len//2). You must do this in-place.
You may not use lst[::-1] or similar techniques.
Do not return anything.
>>> lst1 = [0, 1, 2, 3, 4, 5, 6]
>>> reverse_half(lst1)
>>> lst1
[0, 1, 2, 6, 5, 4, 3]
>>> lst2 = []
>>> reverse_half(lst2)
>>> lst2
[]
>>> lst3 = [0, 1, 2, 3, 4, 5, 6, 7]
>>> reverse_half(lst3)
>>> lst3
[0, 1, 2, 3, 7, 6, 5, 4]
"""
# YOUR CODE GOES HERE #
b)
Write a function that takes in two lists of equal length and swaps their elements at even or odd indices, depending on the 'even' boolean parameter that is passed in. If even is True, swap the elements at the even indices. If even is False, swap the elements at the odd indices. You should not have a return statement, because your function will mutate a given list.
def split_even_odd(lst1, lst2, even=True):
"""
Given two lists of equal length lst1 and lst2,
if even is True, swap all of the values at the even indices.
If even is False, swap all of the values at the odd indices.
Do not return anything. You cannot use another list.
>>> lst1 = [0, 1, 2, 3, 4]
>>> lst2 = [5, 6, 7, 8, 9]
>>> split_even_odd(lst1, lst2, True)
>>> lst1
[5, 1, 7, 3, 9]
>>> lst2
[0, 6, 2, 8, 4]
>>> split_even_odd(lst1, lst2, False)
>>> lst1
[5, 6, 7, 8, 9]
>>> lst2
[0, 1, 2, 3, 4]
"""
# YOUR CODE GOES HERE #
c)
Write a function that takes in two dictionaries, dict1 and dict2. The purpose of the function is to mutate both dictionaries such that dict1 has only odd length keys and dict2 has only even length keys by moving the elements around. If both dictionaries have the same key, add their values.
For example:
dict1 = {'a': 10, 'b': 5, 'ab': 7}
dict2 = {'ab': 20, 'b': 1, 'z': 0, 'hihi': 1}
Since dict1 has a and b as odd length keys, you keep them in dict1. dict2 z, which is odd length, so you move it in dict1. Also, dict1 and dict2 share the same key: b. It should be in dict1 one but update the value to 5+1 = 6. Therefore dict1 is: {'a': 10, 'b': 6, 'z': 0} (order might be different!)
All even length keys should end up in the second dictionary: {'ab': 27,'hihi':1}
Do not return anything. You cannot use another dictionary. You can assume that all keys will be strings, and all values will be integers.
def separate_dicts(dict1, dict2):
"""
Given two dictionaries dict1 and dict2,
mutate the dictionaries such that all keys with odd length
are kept/moved into dict1 and all keys with even length
are kept/moved into dict2.
If there are items with the same key, add their values together.
Do not return anything. You cannot use another dictionary.
>>> dict1 = {'a': 10, 'b': 5, 'ab': 7}
>>> dict2 = {'ab': 20, 'b': 1, 'z': 0, 'hihi': 1}
>>> separate_dicts(dict1, dict2)
>>> sorted(dict1)
['a', 'b', 'z']
>>> [dict1[i] for i in sorted(dict1)]
[10, 6, 0]
>>> sorted(dict2)
['ab', 'hihi']
>>> [dict2[i] for i in sorted(dict2)]
[27, 1]
"""
# YOUR CODE GOES HERE #
Step by Step Solution
There are 3 Steps involved in it
Step: 1
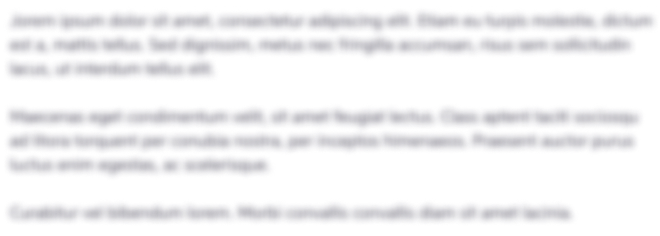
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started