Question
Use Random Access Files to store the data in Binary Form. This means, you should not use an Arrays to store the data in the
Use Random Access Files to store the data in Binary Form. This means, you should not use an Arrays to store the data in the memory. Instead, when the user wants to create a new Employee/Department, you write it to the file right away. Also when the user says he/she wants to edit an Employee/Department, ask the user to enter EmployeeID/DepartmentID. Read the data from the file and display it to the user. Allow the user to enter new data and write it back to the file in the same position inside the file.
//This program displays a menu for the user.
#include
#include
#include
#include
#include
using namespace std;
//1st Class declaration
class Department
{
private:
string departmentID;
string departmentName;
string departmentHeadName;
public:
Department()
{}
Department(string ID, string name)
{
departmentID=" ";
departmentName=" ";
departmentHeadName=" ";
}
Department(string ID, string name, string head)
{
departmentID=ID;
departmentName=name;
departmentHeadName=head;
}
void setDepartmentID(string ID)
{
departmentID=ID;
}
void setDepartmentName(string name)
{
departmentName=name;
}
void setDepartmentHeadName(string head)
{
departmentHeadName=head;
}
string getDepartmentID()
{
return departmentID;
}
string getDepartmentName()
{
return departmentName;
}
string getDepartmentHeadName()
{
return departmentHeadName;
}
};
//2nd Class declaration
class Employee
{
private:
string employeeID;
string employeeName;
double employeeSalary;
int employeeAge;
string employeeDepartmentID;
public:
Employee()
{}
Employee(string ID, string name)
{
employeeID=ID;
employeeName=name;
}
Employee(string ID, string name, int age)
{
employeeID=ID;
employeeName=name;
employeeAge=age;
}
Employee(string ID, string name, int age, double salary)
{
employeeID=ID;
employeeName=name;
employeeAge=age;
employeeSalary=salary;
}
Employee (string ID, string name, int age, double salary, string department)
{
employeeID=ID;
employeeName=name;
employeeAge=age;
employeeSalary=salary;
employeeDepartmentID=department;
}
void setEmployeeID(string ID)
{
employeeID=ID;
}
void setEmployeeName(string name)
{
employeeName=name;
}
void setEmployeeAge(int age)
{
employeeAge=age;
}
void setEmployeeSalary(double salary)
{
employeeSalary=salary;
}
void setEmployeeDepartment(string department)
{
employeeDepartmentID=department;
}
string getEmployeeID()
{
return employeeID;
}
string getEmployeeName()
{
return employeeName;
}
int getEmployeeAge()
{
return employeeAge;
}
double getEmployeeSalary()
{
return employeeSalary;
}
string getEmployeeDepartment()
{
return employeeDepartmentID;
}
};
//Main function
int main()
{
//Variables
Employee e[7]; //Employee array with size 7
Department d[5]; //Department array with size 5
int choice=0;
int employeeAge=0;
int i=0;
int j=0;
string departmentID;
string departmentName;
string departmentHeadName;
string employeeID;
string employeeName;
string employeeDepartmentID;
double employeeSalary=0.0;
ifstream inputFile;
ofstream outputFile;
string dhn;
string Id;
string dn;
string emPd;
double es;
int ea;
string en;
string ed;
do
{
cout<<" 1. Create Department 2. Create Employee";
cout<<" 3. Write DEPARTMENT data to file 4. Retrieve DEPARTMENT data from file";
cout<<" 5. Write EMPLOYEE data to file 6. Retrieve EMPLOYEE data from file";
cout<<" 7. Display Report 8. Exit Enter your choice: ";
cin>>choice;
switch(choice)
{
case 1: if(i<3)
{
cout<<" Enter the department ID: ";
cin.ignore(); //Skip the newline character
getline(cin,departmentID);
d[i].setDepartmentID(departmentID);
cout<<" Enter the department name: ";
getline(cin,departmentName);
d[i].setDepartmentName(departmentName);
cout<<" Enter the department head name: ";
getline(cin,departmentHeadName);
d[i].setDepartmentHeadName(departmentHeadName);
i++;
}
else
cout<<" The array is full, you can not add any more departments ";
break;
case 2: if(j<7)
{
cout<<" Enter the employee ID: ";
cin.ignore();
getline(cin,employeeID);
e[j].setEmployeeID(employeeID);
cout<<" Enter the employee name: ";
getline(cin,employeeName);
e[j].setEmployeeName(employeeName);
cout<<" Enter department ID: ";
getline(cin,departmentID);
e[j].setEmployeeDepartment(departmentID);
cout<<" Enter the employee age: ";
cin>>employeeAge;
e[j].setEmployeeAge(employeeAge);
cout<<" Enter the employee salary: $";
cin>>employeeSalary;
e[j].setEmployeeSalary(employeeSalary);
j++;
}
else
cout<<" The array is full, you can not add any more Employees ";
break;
case 3: cout<<" Writing DEPARTMENT data to file ";
{
ofstream outputFile;
outputFile.open("output.txt");
for(int k=0;k<3;k++)
{
outputFile<<" "< outputFile<<" "< outputFile<<" "< } outputFile.close(); break; } case 4: cout<<" Reading DEPARTMENT data from file "; { ifstream inputFile; inputFile.open("output.txt"); for(int k=0;k<3;k++) { cout<<' '; inputFile>>dn; cout<<"Department Head Name: "< inputFile>>Id; cout<<"Department ID: "< inputFile>>dhn; cout<<"Department Name: "< } inputFile.close(); break; } case 5: cout<<" Writing EMPLOYEE data to file "; { ofstream outputFile; outputFile.open("output.txt"); for(int l=0;l<7;l++) { outputFile<<" "< outputFile<<" "< outputFile<<" "< outputFile<<" "< outputFile<<" "< } outputFile.close(); break; } case 6: cout<<" Reading EMPLOYEE data from file "; { ifstream inputFile; inputFile.open("output.txt"); for(int l=0;l<7;l++) { cout<<' '; inputFile>>emPd; cout<<"Employee Department ID: "< inputFile>>es; cout<<"Employee Salary: $"< inputFile>>ea; cout<<"Employee Age: "< inputFile>>en; cout<<"Employee Name: "< inputFile>>ed; cout<<"Employee ID: "< } inputFile.close(); break; } case 7: cout<<" Report: "; { double totSalary=0; for(j=0;j<7;j++) { totSalary=totSalary+e[j].getEmployeeSalary(); } cout << " The total salary of all the departments employees is: $"< break; } case 8: cout<<" Exiting: "; { char exitChoice; cout<<"ALERT. Data has not been saved and will be lost."; cout<<' '; cout<<" Do you wish to continue (Y/N)? "; cin>>exitChoice; if(exitChoice=='Y' || exitChoice=='y') { cout<<"Thank You. Good Bye"; cout<<' '<<' '; exit(0); break; } else if(exitChoice=='N' || exitChoice=='n' ) {} } default : cout<<" Select Option: "<<' '; break; } }while(choice!=9); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
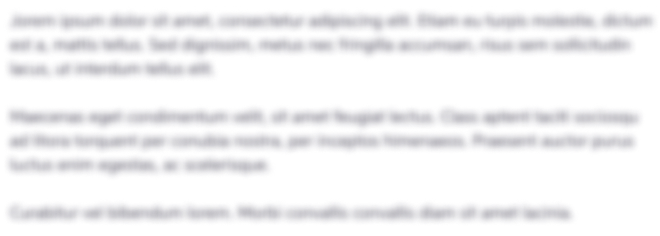
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started