Answered step by step
Verified Expert Solution
Question
1 Approved Answer
use the constructors as in the diagram. and send elevatorperson and elevator files only. & use your own code. thanks In this quiz, you will
use the constructors as in the diagram. and send elevatorperson and elevator files only. & use your own code. thanks
In this quiz, you will write 2 classes ElevatorPerson and Elevator. I provide additional classes you will use which are Mystack (from the lecture), Person, Student and Professor (from quiz 3). They are not fully shown in the class diagram but you can check the source files or the previous quiz explanations. Here is the class diagram. Important: Class, and public method names must be exactly the same with the class diagram. Otherwise your code will not run. Important: Write your name and id to the top of your source files as comments. Write meaningful comments to your code where necessary. Be sure to have correct indentation. Do not write a package name. Explanations: - Do not change the provided classes. Use them as they are - ElevatorPerson: This is to store a person along with extra information such as initial and target floors. - ElevatorPerson(person, ip, t): This is the only constructor and it takes three parameters - Person p: (can be a Student or a Professor as well - int ip: initialPosition from where the person enters the elevator. - int t : target floor where the person wants to go - In the constructor you need to initialize enterTime with the value of the static variable travelMeter of the Elevator as follows: enterTime = Elevator .getTravelMeter(); - You need to write getter methods for person and target as shown in the class diagram. - tostring() method will be overridden as follows. I am Fatih. I traveled 4 floors. I am happy. I am Ahmet. I traveled 12 floors. I am unhappy. That means Fatih traveled 4 floors e.g., (from 2 to 6 ) or (from 7 to 3 ) etc. This is the difference of elevator person's enterTime and elevator's current travelMeter. Therefore, he is happy because he did not waste his time when going from his initialposition to his target yet. Ahmet is not happy because he did not go to his target directly and wasted some time (Probably waited for Fatih's travel first.). In general, if the travel time is less than or equals to |target-initialPosition | the person is happy, otherwise unhappy. Elevator is a class simulating an elevator designed as a stack (last in first out). It keeps people in a stack so the person who entered the last needs to leave the first. - It has 6 private variables - minfloor and maxFloor indicate the minimum and maximum floors that the elevator can go. Their default values are 0 and 10 . - capacity: how many people the elevator can accommodate at the same time. - currentFloor: current position of the elevator. - people: this is an instance of MyStack to keep Person's. - travelMeter: to keep track of the total travel done with the elevator. This is a static variable. Whenever the elevator moves from floor a to b it should be incremented by ba. - There are two constructors, Elevator(name) and Elevator(size, minFloor, maxFloor). They set currentFloor to 0 and initiates people as an empty Mystack. No-arg constructor sets default values for size to 4 , minFloor to 0 , and maxFloor to 10 . - enter(Person p, int target): generates an ElevatorPerson and adds it to the people stack if there is available space. - If there is available space in the elevator, this method should print a string like "Ali is in." with the person's name and return true. It should return false otherwise. - Important: throw an IllegalArgumentException if the provided target floor does not exist, i.e., it is less than minfloor or larger than maxFloor. add this for instance: throw new IllegalArgumentException("target " + target + " out of bounds"); goToFloor(int floor): - If the specified floor does not exist, throw an IllegalArgumentException as in enter(..) method. - Elevator goes to the specified floor, i.e., change currentFloor. - Increment travelMeter by the travel amount | newFloor-previousFloor| - If the people at the top of the stack reached their target, they leave. - There might be one or more ElevatorPerson to reach their targets. - Print "Ali is out." - Print the ElevatorPerson who just left. - At the end of the method print the elevator (System.out.printin(this)). releaseEveryone(): - calls goToFloor(floor) method with the desired targets of the people until the elevator is empty. - isFull() and isEmpty() methods return a boolean value according to elevator's stack status. - toString() - returns a string as follows. Elevator is on floor: 5 , Number of people: 1 Sample run: -- The code -- //try Elevatorperson ElevatorPerson ep = new ElevatorPerson (new Person ("Ebru"), 2, 5); System.out.println(ep); /ot traveled yet so she is happy. 1/try Elevator Elevator e = new Elevator(); Person p1 = new Student ("A11", 1); Person p2 = new Student ("zeynep", 2); Person p3 = new Professor("Fatih", 1); e.enter (p1,8); e.goToFloor (1); e.enter (p2,5); e.goToFloor (5); //p2 leaves e.goToFloor (3); e.enter (p3,9); e.releaseEveryone (); - - corresponding output - I am Ebru. I traveled 0 floors. I am happy. Ali is in. Elevator is on floor: 1, Number of people: 1 Zeynep is in. Zeynep is out. I am Zeynep. I traveled 4 floors. I am happy. Elevator is on floor: 5, Number of people: 1 Elevator is on floor: 3, Number of people: 1 Fatih is in. Eatih is out. I am Fatih. I traveled 6 floors. I am happy. Elevator is on floor: 9, Number of people: 1 Ali is out. I am Ali. I traveled 14 floors. I am unhappy. Elevator is on floor: 8 , Number of people: 0 Submission: Send ElevatorPerson.java and Elevator.java to aybuzem as separate files. 3 In this quiz, you will write 2 classes ElevatorPerson and Elevator. I provide additional classes you will use which are Mystack (from the lecture), Person, Student and Professor (from quiz 3). They are not fully shown in the class diagram but you can check the source files or the previous quiz explanations. Here is the class diagram. Important: Class, and public method names must be exactly the same with the class diagram. Otherwise your code will not run. Important: Write your name and id to the top of your source files as comments. Write meaningful comments to your code where necessary. Be sure to have correct indentation. Do not write a package name. Explanations: - Do not change the provided classes. Use them as they are - ElevatorPerson: This is to store a person along with extra information such as initial and target floors. - ElevatorPerson(person, ip, t): This is the only constructor and it takes three parameters - Person p: (can be a Student or a Professor as well - int ip: initialPosition from where the person enters the elevator. - int t : target floor where the person wants to go - In the constructor you need to initialize enterTime with the value of the static variable travelMeter of the Elevator as follows: enterTime = Elevator .getTravelMeter(); - You need to write getter methods for person and target as shown in the class diagram. - tostring() method will be overridden as follows. I am Fatih. I traveled 4 floors. I am happy. I am Ahmet. I traveled 12 floors. I am unhappy. That means Fatih traveled 4 floors e.g., (from 2 to 6 ) or (from 7 to 3 ) etc. This is the difference of elevator person's enterTime and elevator's current travelMeter. Therefore, he is happy because he did not waste his time when going from his initialposition to his target yet. Ahmet is not happy because he did not go to his target directly and wasted some time (Probably waited for Fatih's travel first.). In general, if the travel time is less than or equals to |target-initialPosition | the person is happy, otherwise unhappy. Elevator is a class simulating an elevator designed as a stack (last in first out). It keeps people in a stack so the person who entered the last needs to leave the first. - It has 6 private variables - minfloor and maxFloor indicate the minimum and maximum floors that the elevator can go. Their default values are 0 and 10 . - capacity: how many people the elevator can accommodate at the same time. - currentFloor: current position of the elevator. - people: this is an instance of MyStack to keep Person's. - travelMeter: to keep track of the total travel done with the elevator. This is a static variable. Whenever the elevator moves from floor a to b it should be incremented by ba. - There are two constructors, Elevator(name) and Elevator(size, minFloor, maxFloor). They set currentFloor to 0 and initiates people as an empty Mystack. No-arg constructor sets default values for size to 4 , minFloor to 0 , and maxFloor to 10 . - enter(Person p, int target): generates an ElevatorPerson and adds it to the people stack if there is available space. - If there is available space in the elevator, this method should print a string like "Ali is in." with the person's name and return true. It should return false otherwise. - Important: throw an IllegalArgumentException if the provided target floor does not exist, i.e., it is less than minfloor or larger than maxFloor. add this for instance: throw new IllegalArgumentException("target " + target + " out of bounds"); goToFloor(int floor): - If the specified floor does not exist, throw an IllegalArgumentException as in enter(..) method. - Elevator goes to the specified floor, i.e., change currentFloor. - Increment travelMeter by the travel amount | newFloor-previousFloor| - If the people at the top of the stack reached their target, they leave. - There might be one or more ElevatorPerson to reach their targets. - Print "Ali is out." - Print the ElevatorPerson who just left. - At the end of the method print the elevator (System.out.printin(this)). releaseEveryone(): - calls goToFloor(floor) method with the desired targets of the people until the elevator is empty. - isFull() and isEmpty() methods return a boolean value according to elevator's stack status. - toString() - returns a string as follows. Elevator is on floor: 5 , Number of people: 1 Sample run: -- The code -- //try Elevatorperson ElevatorPerson ep = new ElevatorPerson (new Person ("Ebru"), 2, 5); System.out.println(ep); /ot traveled yet so she is happy. 1/try Elevator Elevator e = new Elevator(); Person p1 = new Student ("A11", 1); Person p2 = new Student ("zeynep", 2); Person p3 = new Professor("Fatih", 1); e.enter (p1,8); e.goToFloor (1); e.enter (p2,5); e.goToFloor (5); //p2 leaves e.goToFloor (3); e.enter (p3,9); e.releaseEveryone (); - - corresponding output - I am Ebru. I traveled 0 floors. I am happy. Ali is in. Elevator is on floor: 1, Number of people: 1 Zeynep is in. Zeynep is out. I am Zeynep. I traveled 4 floors. I am happy. Elevator is on floor: 5, Number of people: 1 Elevator is on floor: 3, Number of people: 1 Fatih is in. Eatih is out. I am Fatih. I traveled 6 floors. I am happy. Elevator is on floor: 9, Number of people: 1 Ali is out. I am Ali. I traveled 14 floors. I am unhappy. Elevator is on floor: 8 , Number of people: 0 Submission: Send ElevatorPerson.java and Elevator.java to aybuzem as separate files. 3Step by Step Solution
There are 3 Steps involved in it
Step: 1
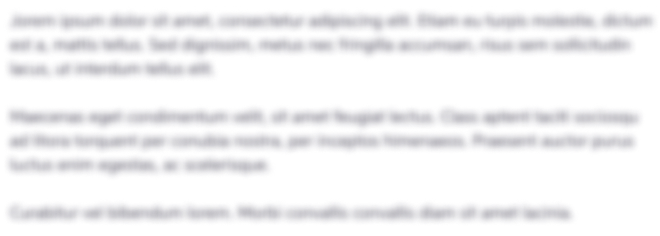
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started