Use the following specifications listed to get the output found at the bottom of the post
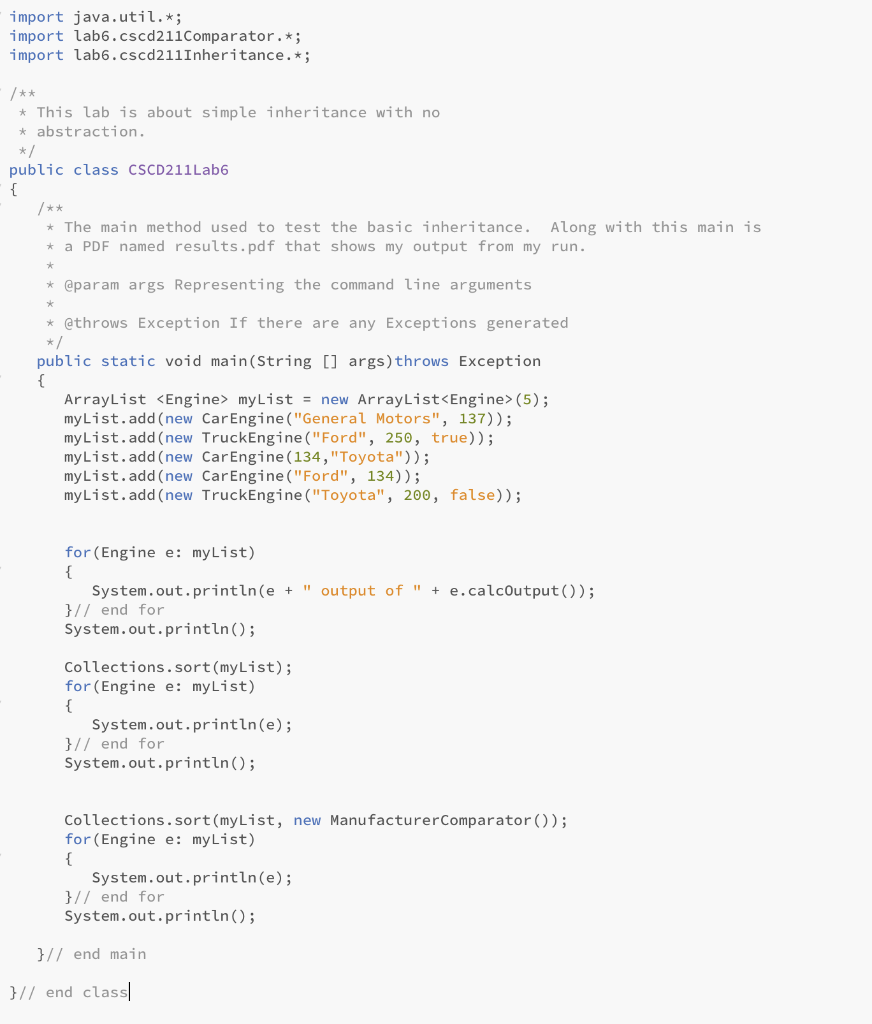
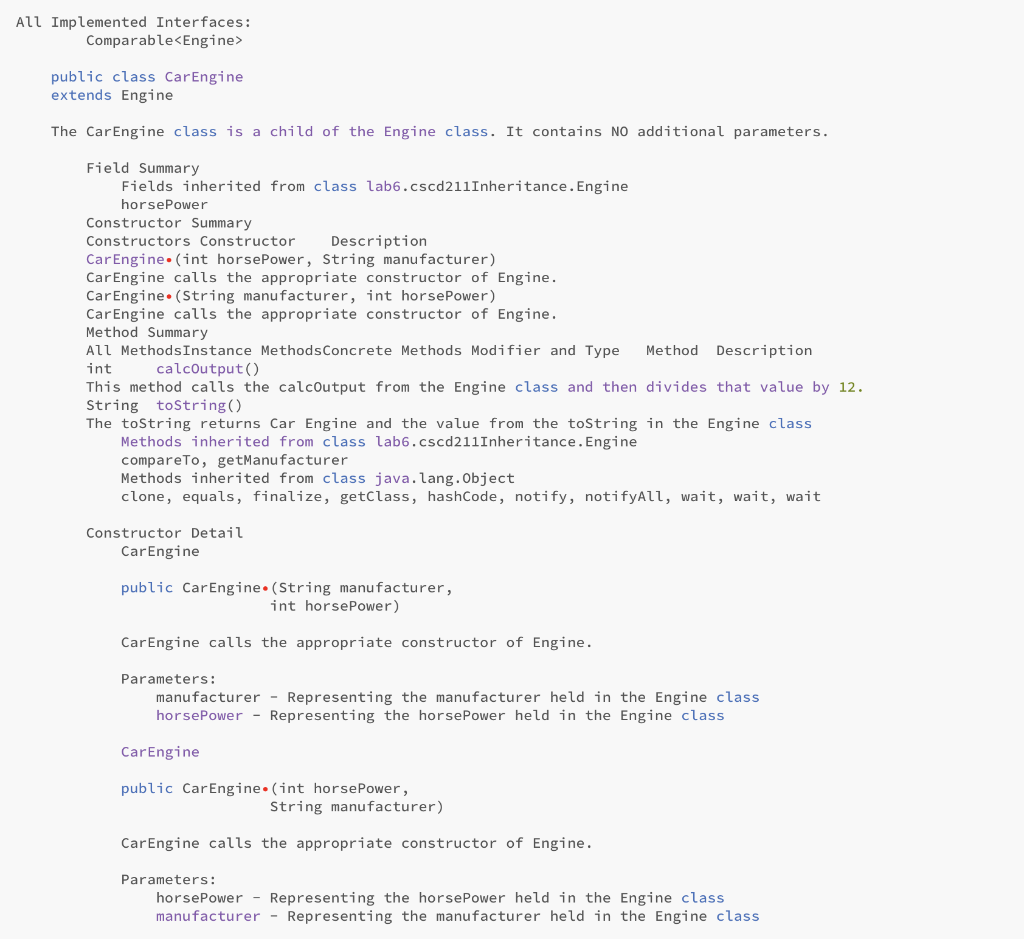
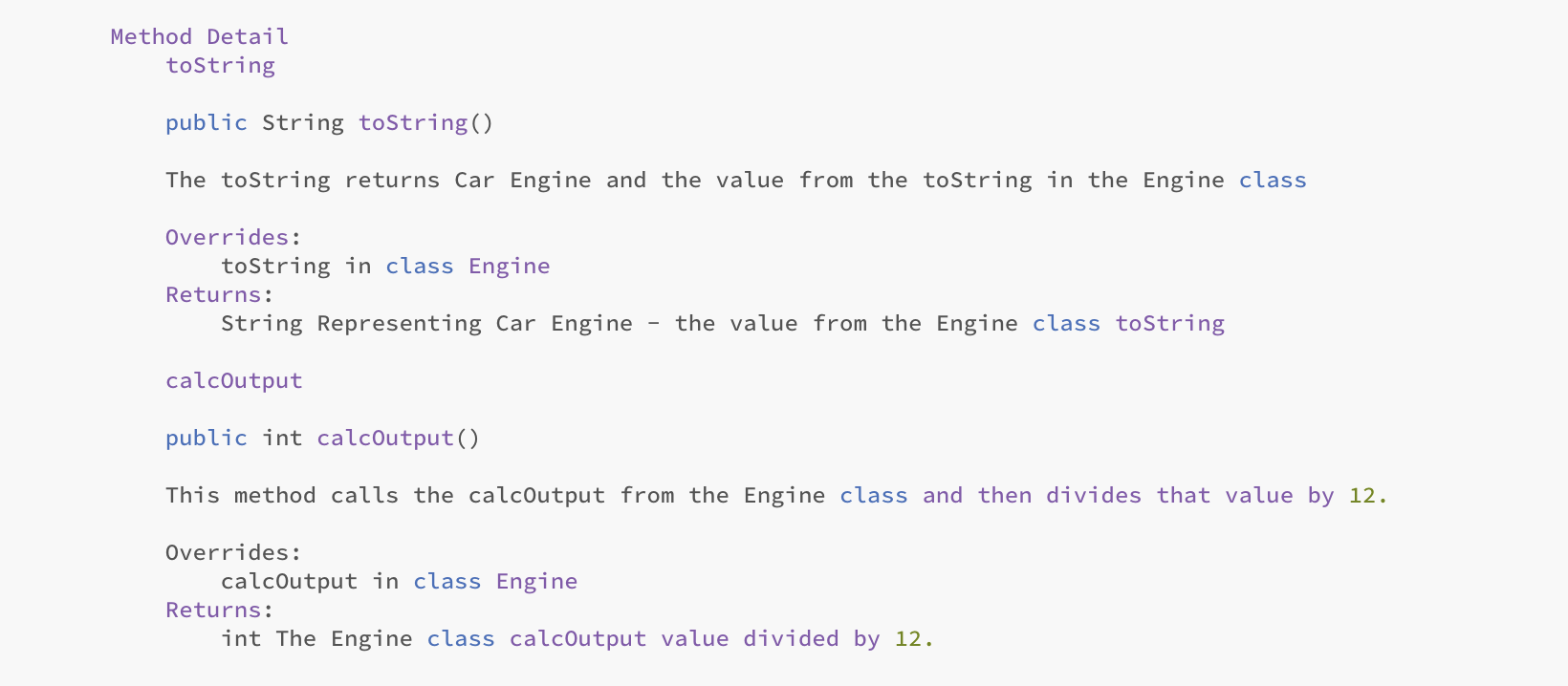
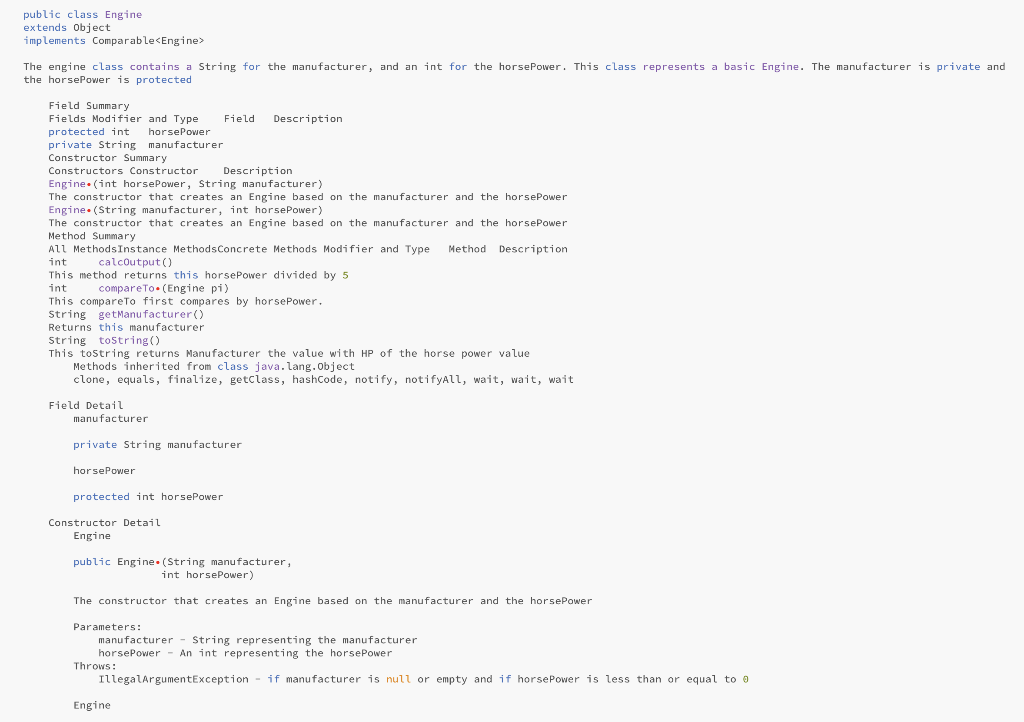

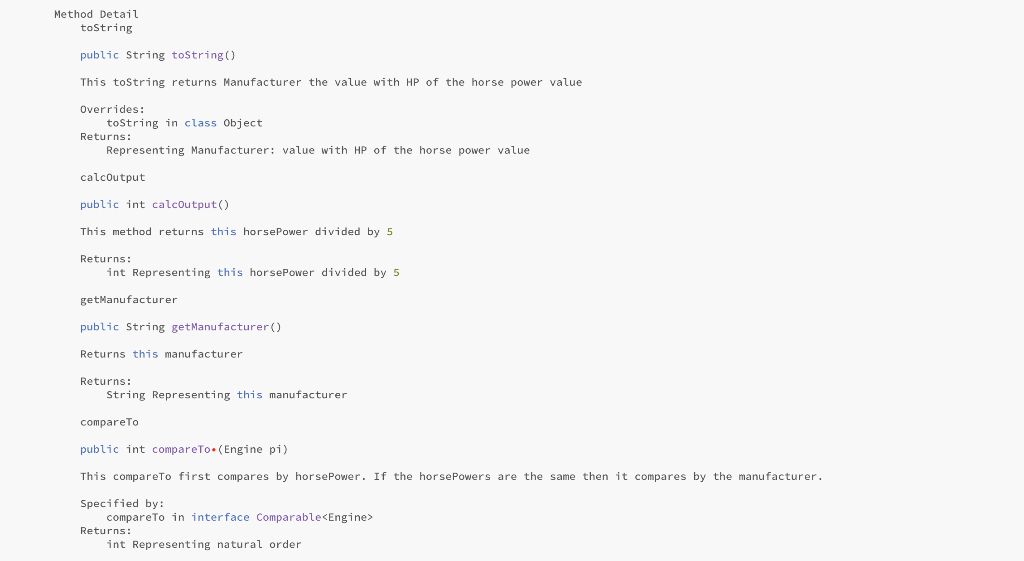
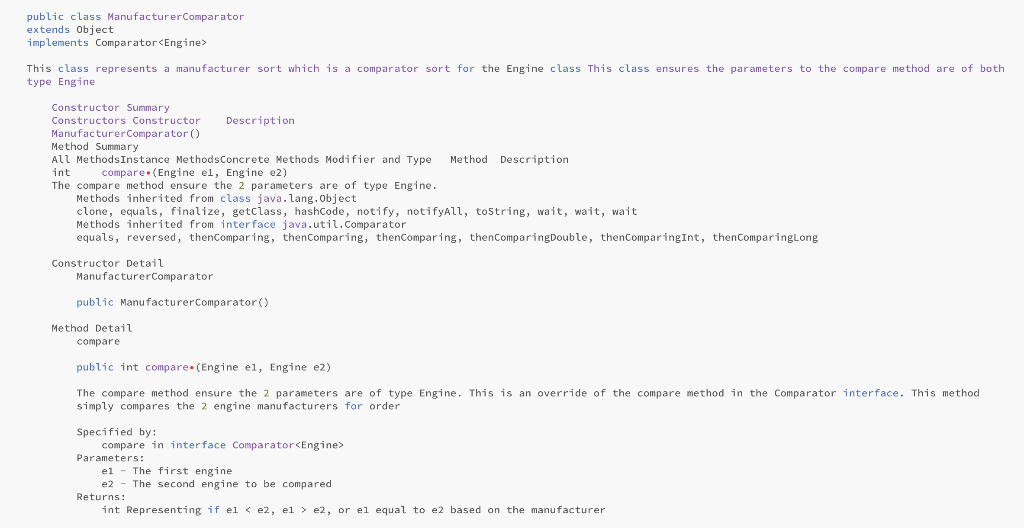
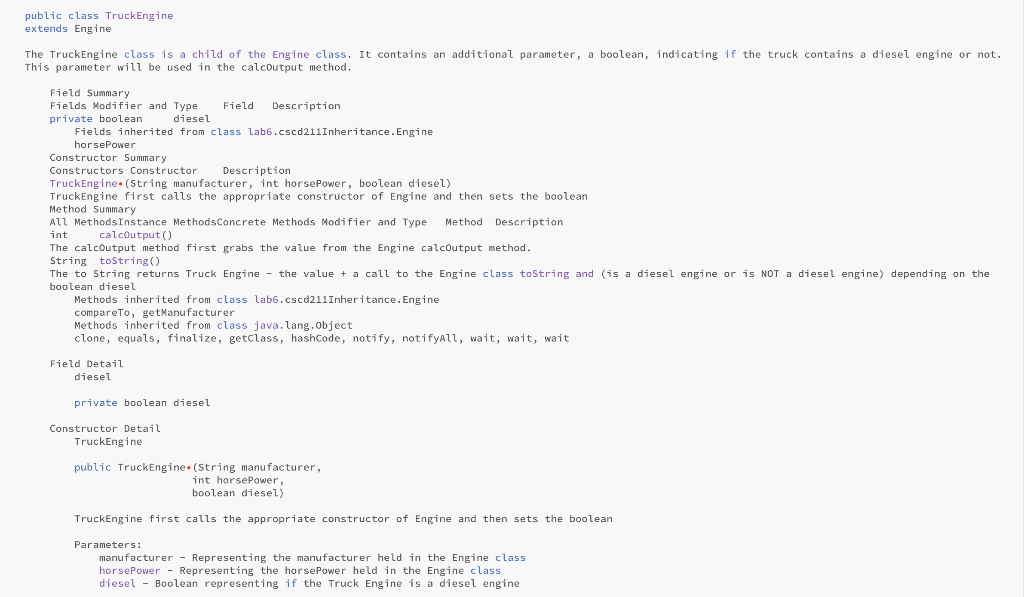
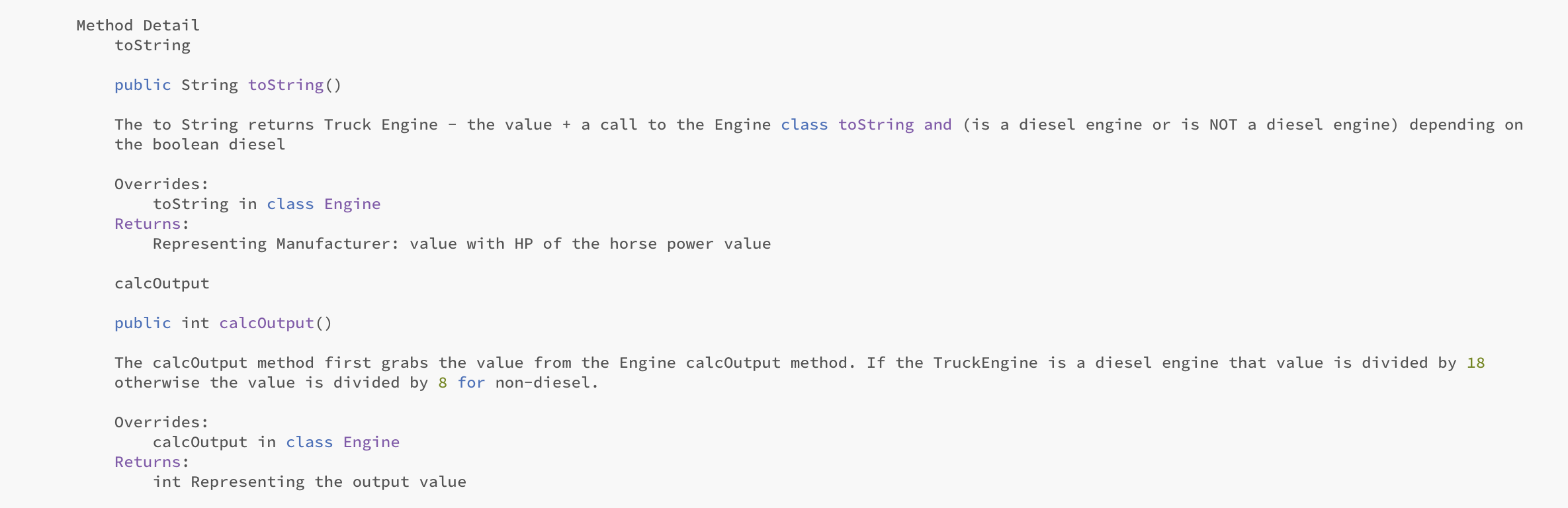
The Output
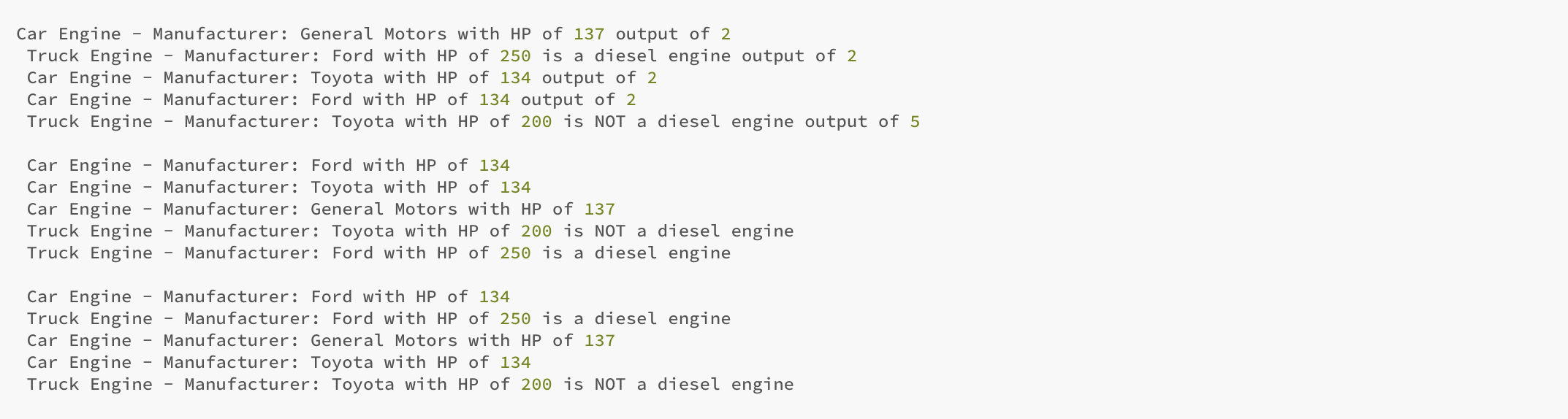
import java.util.*; import lab6.cscd211 Comparator.*; import lab6.cscd211Inheritance. *; /** * This lab is about simple inheritance with no * abstraction. */ public class CSCD211 Lab6 { /** * The main method used to test the basic inheritance. Along with this main is * a PDF named results.pdf that shows my output from my run. * * @param args Representing the command line arguments * * @throws Exception If there are any Exceptions generated */ public static void main(String [] args) throws Exception { ArrayList
myList = new ArrayList(5); myList.add(new Car Engine("General Motors", 137)); myList.add(new TruckEngine ("Ford", 250, true)); myList.add(new Car Engine (134, "Toyota")); myList.add(new CarEngine ("Ford", 134)); myList.add(new TruckEngine("Toyota", 200, false)); for (Engine e: myList) { System.out.println(e + " output of " + e.calcOutput(); } // end for System.out.println(); Collections.sort(myList); for (Engine e: myList) { System.out.println(e); } // end for System.out.println(); Collections.sort(myList, new Manufacturer Comparator()); for (Engine e: myList) { System.out.println(e); } // end for System.out.println(); }// end main }// end class All Implemented Interfaces: Comparable public class CarEngine extends Engine The CarEngine class is a child of the Engine class. It contains NO additional parameters. Field Summary Fields inherited from class lab6.cscd211Inheritance. Engine horsePower Constructor Summary Constructors Constructor Description CarEngine. (int horsePower, String manufacturer) CarEngine calls the appropriate constructor of Engine. CarEngine. (String manufacturer, int horsePower) CarEngine calls the appropriate constructor of Engine. Method Summary All Methods Instance MethodsConcrete Methods Modifier and Type Method Description int calcoutput) This method calls the calcOutput from the Engine class and then divides that value by 12. String toString() The toString returns Car Engine and the value from the toString in the Engine class Methods inherited from class labb.cscd211Inheritance. Engine compare To, getManufacturer Methods inherited from class java.lang. Object clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Constructor Detail CarEngine public Car Engine(String manufacturer, int horsePower) CarEngine calls the appropriate constructor of Engine. Parameters: manufacturer - Representing the manufacturer held in the Engine class horsePower - Representing the horsepower held in the Engine class CarEngine public CarEngine. (int horsepower, String manufacturer) CarEngine calls the appropriate constructor of Engine. Parameters: horsepower - Representing the horsepower held in the Engine class manufacturer - Representing the manufacturer held in the Engine class Method Detail toString public String toString() The toString returns Car Engine and the value from the toString in the Engine class Overrides: toString in class Engine Returns: String Representing Car Engine the value from the Engine class toString calcOutput public int calcoutput() This method calls the calcOutput from the Engine class and then divides that value by 12. Overrides: calcOutput in class Engine Returns: int The Engine class calcoutput value divided by 12. public class Engine extends Object implements Comparable The engine class contains a String for the manufacturer, and an int for the horsepower. This class represents a basic Engine. The manufacturer is private and the horsepower is protected Field Summary Fields Modifier and Type Field Description protected int horsePower private String manufacturer Constructor Summary Constructors Constructor Description Engine. (int horsePower, String manufacturer) The constructor that creates an Engine based on the manufacturer and the horsePower Engine.(String manufacturer, int horsePower) The constructor that creates an Engine based on the manufacturer and the horsePower Method Summary All Methods Instance MethodsConcrete Methods Modifier and Type Method Description int calcOutput() This method returns this horsepower divided by 5 int compareto. (Engine pi) This compare to first compares by horsepower. String getManufacturer() Returns this manufacturer String toString() This toString returns Manufacturer the value with HP of the horse power value Methods inherited from class java.lang. Object clone, equals, finalize, getClass, hashcode, notify, notifyAll, wait, wait, wait Field Detail manufacturer private String manufacturer horsePower protected int horsePower Constructor Detail Engine public Engine(String manufacturer, int horsePower) The constructor that creates an Engine based on the manufacturer and the horsePower Parameters: manufacturer - String representing the manufacturer horsePower - An int representing the horsepower Throws: IllegalArgumentException - if manufacturer is null or empty and if horsepower is less than or equal to o Engine public Engine: (int horsepower, String manufacturer) The constructor that creates an Engine based on the manufacturer and the horsePower Parameters: manufacturer - String representing the manufacturer horsePower - An int representing the horsePower Throws: IllegalArgumentException if manufacturer is null or empty and if horsepower is less than or equal to o Method Detail toString public String toString() This toString returns Manufacturer the value with HP of the horse power value Overrides: toString in class Object Returns: Representing Manufacturer: value with HP of the horse power value calcOutput public int calcoutput) This method returns this horsepower divided by 5 Returns: int Representing this horsepower divided by 5 getManufacturer public String getManufacturer Returns this manufacturer Returns: String Representing this manufacturer compareTo public int compareTo (Engine pi) This compareto first compares by horsepower. If the horsepowers are the same then it compares by the manufacturer. Specified by: compareto in interface comparable Returns: int Representing natural order public class Manufacturer Comparator extends Object implements Comparator This class represents a manufacturer sort which is a comparator sort for the Engine class This class ensures the parameters to the compare method are of both type Engine Constructor Summary Constructors Constructor Description Manufacturer Comparator) Method Summary All Methods Instance Methods Concrete Methods Modifier and Type Method Description int compare. (Engine el, Engine e2) The compare method ensure the 2 parameters are of type Engine. Methods inherited from class java.lang. Object clone, equals, finalize, getClass, hashCode, notify, notifyAll, tostring, wait, wait, wait Methods inherited from interface java.util.comparator equals, reversed, thenComparing, thenComparing, thenComparing, thenComparingDouble, thenComparingInt, thenComparingLong Constructor Detail Manufacturer Comparator public Manufacturer Comparator() Method Detail compare public int compare.(Engine el, Engine e2) The compare method ensure the 2 parameters are of type Engine. This is an override of the compare method in the Comparator interface. This method simply compares the 2 engine manufacturers for order Specified by: compare in interface Comparator Parameters: el - The first engine e2 The second engine to be compared Returns: int Representing if el e2, el > e2, or el equal to e2 based on the manufacturer public class TruckEngine extends Engine The TruckEngine class is a child of the Engine class. It contains an additional parameter, a boolean, indicating if the truck contains a diesel engine or not. This parameter will be used in the calcOutput method. Field Summary Fields Modifier and Type Field Description private boolean diesel Fields inherited from class labb.cscd211Inheritance. Engine horsePower Constructor Summary Constructors Constructor Description TruckEngine(String manufacturer, int horsepower, boolean diesel) TruckEngine first calls the appropriate constructor of Engine and then sets the boolean Method Summary All Methods Instance MethodsConcrete Methods Modifier and Type Method Description int calcOutput The calcOutput method first grabs the value from the Engine calcOutput method. String toString() The to String returns Truck Engine - the value + a call to the Engine class toString and (is a diesel engine or is NOT a diesel engine) depending on the boolean diesel Methods inherited from class labs.cscd211Inheritance. Engine compareTo, getManufacturer Methods inherited from class java.lang. Object clone, equals, finalize, getClass, hashcode, notify, notifyAll, wait, wait, wait Field Detail diesel private boolean diesel Constructor Detail TruckEngine public TruckEngine.(String manufacturer, int horsePower, boolean diesel) TruckEngine first calls the appropriate constructor of Engine and then sets the boolean Parameters: manufacturer - Representing the manufacturer held in the Engine class horsepower - Representing the horsepower held in the Engine class diesel - Boolean representing if the Truck Engine is a diesel engine Method Detail toString public String toString() The to String returns Truck Engine - the value + a call to the Engine class toString and (is a diesel engine or is NOT a diesel engine) depending on the boolean diesel Overrides: toString in class Engine Returns: Representing Manufacturer: value with HP of the horse power value calcOutput public int calcOutput() The calcoutput method first grabs the value from the Engine calcoutput method. If the TruckEngine is a diesel engine that value is divided by 18 otherwise the value is divided by 8 for non-diesel. Overrides: calcoutput in class Engine Returns: int Representing the output value Car Engine - Manufacturer: General Motors with HP of 137 output of 2 Truck Engine - Manufacturer: Ford with HP of 250 is a diesel engine output of 2 Car Engine - Manufacturer: Toyota with HP of 134 output of 2 Car Engine Manufacturer: Ford with HP of 134 output of 2 Truck Engine Manufacturer: Toyota with HP of 200 is NOT a diesel engine output of 5 Car Engine Manufacturer: Ford with HP of 134 Car Engine Manufacturer: Toyota with HP of 134 Car Engine Manufacturer: General Motors with HP of 137 Truck Engine Manufacturer: Toyota with HP of 200 is NOT a diesel engine Truck Engine - Manufacturer: Ford with HP of 250 is a diesel engine Car Engine Manufacturer: Ford with HP of 134 Truck Engine Manufacturer: Ford with HP of 250 is a diesel engine Car Engine - Manufacturer: General Motors with HP of 137 Car Engine Manufacturer: Toyota with HP of 134 Truck Engine Manufacturer: Toyota with HP of 200 is NOT a diesel engine