Question
Use the personType class files and employeeType class files included below the following instructions. Use C++ for the program. Step1: Create a class Intern that
Use the personType class files and employeeType class files included below the following instructions.
Use C++ for the program.
Step1: Create a class Intern that is a derived class from the employeeType class
- Create a header file for the Intern class that contains the declarations for the data members and methods
- Create an implementation file for the Intern class that contains the implementation of the class methods
- Include the following data members in the Intern class
- year - store the intern's academic standing of Freshman, Sophomore, Junior, Senior or Graduate
- hours - hours worked in the week
- stipend - a base amount the intern receives each week
- Include the following methods in the Intern class
- set() - Function that will take 6 parameters to set the first name, last name, id, year, hours and stipend according to the parameter list
- print() - Function to output the Id, first name, last name, and pay
- calculatePay() - Function to calculate and return the pay for the intern. The pay will be calculated by taking the stipend amount and adding the hours worked by the standard pay rate for the academic year of the intern as follows
- Freshman - $10/hour
- Sophomore - $11/hour
- Junior - $14/hour
- Senior - $16/hour
- Graduate - $20/hour
- A constructor that will accept 6 parameters to initialize each data member of the Intern class
- The appropriate get and set methods for the year, hours and stipend data members
Step 2: Write a main program to do the following:
- Dynamically create an array of Intern objects. Create the size of the array from user input
- Accept user input for each of the intern objects in the array.
- Accept data for the name, ID, year, hours and stipend
- Do not allow the user to input data for Intern objects when the size of the array has been reached
- Allow the user to choose to stop entering data for the Intern objects before the number of objects in the array is reached
- Output the ID, Name, and pay for each Intern
- Make sure the code only outputs objects for which data has been entered. If the user chose to stop entering data before the total number of objects in the array was reached, do not output data for any objects that user data was not input
***** employeeType.h file *****
#ifndef H_employeeType #define H_employeeType #include "personType.h" class employeeType: public personType { public: virtual void print() const = 0; //Function to output employee's data.
virtual double calculatePay() const = 0; //Function to calculate and return the wages. //Postcondition: Pay is calculated and returned
void setId(long id); //Function to set the salary. //Postcondition: personId = id
long getId() const; //Function to retrieve the id. //Postcondition: returns personId
employeeType(string first = "", string last = "", long id = 0); //Constructor with parameters //Sets the first name, last name, payRate, and //hoursWorked according to the parameters. If //no value is specified, the default values are //assumed. //Postcondition: firstName = first; // lastName = last; personId = id
private: long personId; //stores the id };
#endif
***** employeeTypeImp.cpp file *****
#include
using namespace std; void employeeType::setId(long id) { personId = id; } long employeeType::getId() const { return personId; }
employeeType::employeeType(string first, string last, long id) : personType(first, last) { personId = id; }
***** personType.h file *****
.h//personType.h #ifndef H_personType #define H_personType #include
class personType { public: void print() const; //Function to output the first name and last name //in the form firstName lastName. void setName(string first, string last); //Function to set firstName and lastName according //to the parameters. //Postcondition: firstName = first; lastName = last
string getFirstName() const; //Function to return the first name. //Postcondition: The value of the firstName is returned.
string getLastName() const; //Function to return the last name. //Postcondition: The value of the lastName is returned.
personType(string first = "", string last = ""); //constructor //Sets firstName and lastName according to the parameters. //The default values of the parameters are empty strings. //Postcondition: firstName = first; lastName = last
private: string firstName; //variable to store the first name string lastName; //variable to store the last name };
#endif
***** personTypeImp.cpp file *****
//personTypeImp.cpp
#include
void personType::print() const { cout << firstName << " " << lastName; }
void personType::setName(string first, string last) { firstName = first; lastName = last; }
string personType::getFirstName() const { return firstName; }
string personType::getLastName() const { return lastName; }
//constructor personType::personType(string first, string last) { firstName = first; lastName = last; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
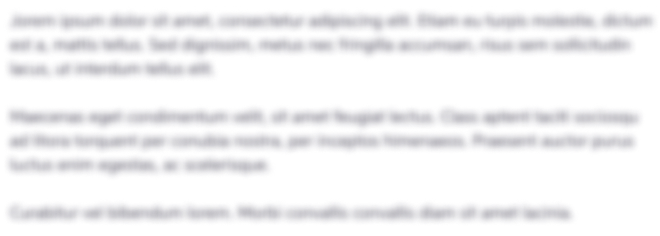
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started