Question
Use the provided main function file myword_main.c to test your programs as the following. Public test gcc mystring.c myword.c myword_main.c -o q2 q2 word processing:done
Use the provided main function file myword_main.c to test your programs as the following.
Public test
gcc mystring.c myword.c myword_main.c -o q2 q2 word processing:done line count:2 word count:10 distinct word count:6 this:2 is:2 the:2 first:1 test:2 second:1 saving result to file:done
Use mystring.h and mystring.c of Q1. Download and save myword_main.c to the C:/cp264/a3 directory.
Create myword.h and then develop myword.c programs.
myword.h
#ifndef MYWORD_H #define MYWORD_H #define MAX_WORD 30 #define MAX_LINE_LEN 1000 #define MAX_WORDS 1000 typedef struct word { char word[MAX_WORD]; int frequency; } WORD; typedef struct words { WORD word_array[MAX_WORDS]; int line_count; int word_count; int distinct_keyword_count; } WORDINFO; int process_word(char *filename, WORDINFO *wip); int save_to_file(char *filename, WORDINFO *wip); #endif
myword.c
#include#include #include "mystring.h" #include "myword.h" int process_word(char *infilename, WORDINFO *wip) { const char delimiters[] = " .,;:!()&?- \t \"\'"; // your implementation } int save_to_file(char *outfilename, WORDINFO *wip) { // your implementation }
use make to build
For Q2, you can try to use C build tool make to compile, run the program. Download Q2 makefile a save as file name: makefile to c:/cp264/a3 folder. Open makefile in text editor, it has the following contents.
q2.exe : q2.o mystring.o myword.o gcc q2.o mystring.o myword.o -o q2.exe q2.o : myword_main.c gcc -c myword_main.c -o q2.o myword.o : myword.h myword.c gcc -c myword.c -o myword.o mystring.o : mystring.h mystring.c gcc -c mystring.c -o mystring.o run: q2.exe q2.exe clean : rm q2.o mystring.o myword.o q2.exe
Then open cmd console, cd to c:/cp264/a3 folder. Type command: make to build q2.exe, run by typing: make run, and clean by typing: make clean
myword_main.c
#include
#include
#include "mystring.h"
#include "myword.h"
void display_word_summary(WORDINFO *wip) {
printf("%s:%d ", "line count", wip->line_count);
printf("%s:%d ", "word count", wip->word_count);
printf("%s:%d ", "distinct word count", wip->distinct_word_count);
int i;
for (i = 0; i distinct_word_count; i++) {
printf("%s:%d ", wip->word_array[i].word, wip->word_array[i].frequency);
}
}
int main(int argc, char *args[]) {
char infilename[40] = "textdata.txt"; //default input file name
char outfilename[40] = "result.txt"; //default output file name
if (argc > 1) {
if (argc >= 2) strcpy(infilename, args[1]);
if (argc >= 3) strcpy(outfilename, args[2]);
}
WORDINFO wordinfo = {0};
process_word(infilename, &wordinfo);
printf("word processing:done ");
display_word_summary(&wordinfo);
save_to_file(outfilename, &wordinfo);
printf("saving result to file:done ");
return 0;
}
Develop a C program which reads and processes an input text file like this textdata.txt. It retrieves the following information. I number of lines i number of words I number of distinct words words and their frequencies The program outputs the retrieved information to a file like this result.txt. Specifically, write C programs myword.h containing the following structure definitions and function headers, and myword.c containing the implementations of the functions. It is required to use your trim() and lower_case() functions from Q1, and it is allowed to use other string functions such as strcat() and strstr() from string library string.h 1. structure definitions: typedef struct word { char word[ 30 ]; int frequency; } WORD; typedef struct words { WORD word_array(1000); int line_count; int word_count; int distinct_word_count; } WORDINFO; 2. int process_word (char *filename, WORDINFO *wordinfo) opens and reads text file of name passed by *filename line by line. For each line, it gets each word, check if it is already in array wordinfo->word_array, if yes, increases its frequency by 1, otherwise inserts it to the end of the word_array and set its frequency 1, and update word_count and distinct_word_count. 3. int save_to_file(char *filename, WORDINFO *wordinfo) saves the data of WORDINFO words to file of name passed by filename in specified format
Step by Step Solution
There are 3 Steps involved in it
Step: 1
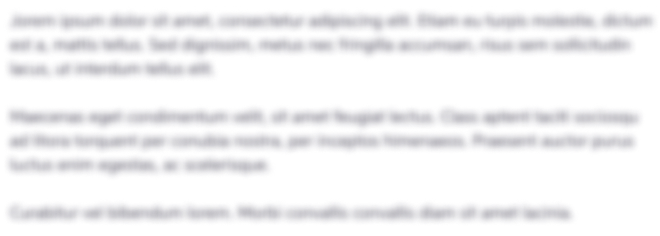
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started