Question
Use the scoreData header and implementation files included below the instructions. Use C++ for the program Step 1: Create a copy constructor for the scoreData
Use the scoreData header and implementation files included below the instructions.
Use C++ for the program
Step 1: Create a copy constructor for the scoreData class. The copy constructor should
- Copy the value of the data members name and size from the parameter object
- Dynamically create an array using the pointer data member scores
- Copy all the elements from the array in the parameter object to the newly created dynamic array
Step 2: Create a destructor for the scoreData class
Step 3: Write a program that includes the following
- Create a scoreData object
- Have the user enter test scores into the scoreData object
- Declare a second scoreData object and initialize the second object to the first scoreData object using the copy constructor
- Update the first score in the second scoreData object
- Output the test scores and average for both scoreData objects
***** scoreData.h file *****
#include #include using namespace std;
class scoreData{
private: string name; // Student name int size; // int *scores; // Pointer pointing to int public: scoreData(); // Default Constructure scoreData (int size); // Constructor that will accept an argument for each of the size data member void addValue(int subscript, int testscore); // Function to be used to store a single test score value to an element in the scores array double calcAvg(); // Calculate the average of the test scores stored in the scores data member array void print(); // Output the test scores in the scores data member array string getName(); // Get Name Accessor function void setName(string Name); // Set Names Mutator function }; #pragma once
***** scoreData.cpp Implementation file *****
#include #include #include #include "scoreData.h" using namespace std;
scoreData::scoreData() { size = 0; }
scoreData::scoreData(int sizeArray) { size = sizeArray; // Set the size data member scores = new int[size]; for (int count = 0; count < size; count++) { scores[count] = 0; } }
void scoreData::addValue(int subscript, int testscore) { scores[subscript] = testscore; }
double scoreData::calcAvg() { int average = 0; // int iterations = 0; int total_scores = 0;
// iterations = sizeof scores;
for (int count = 0; count < size; count++) { total_scores += scores[count]; }
average = (total_scores / size); cout << "The average score for " << name << " is " << average << endl; return average; }
void scoreData::print() { // int iterations = 0; // iterations = sizeof scores; cout << "The scores for " << name << endl; for (int count = 0; count < size; count++) { cout << "Score " << (count + 1) << " is " << scores[count] << endl; } }
string scoreData::getName() { return name; }
void scoreData::setName(string Name) { if (Name != "") name = Name; // else leaves it set to its previous value }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
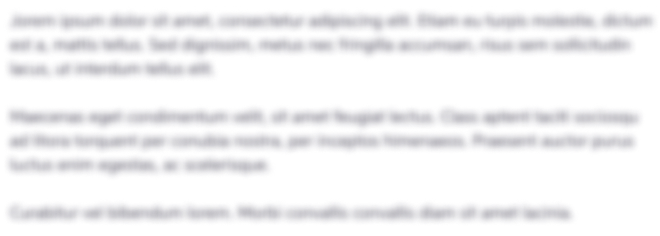
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started