Question
Use the template files provided in zyBooks 12.4 (and at the end of this file) to complete the following exercises. Each step of the instructions
Use the template files provided in zyBooks 12.4 (and at the end of this file) to complete the following exercises.
Each step of the instructions is associated with specific tests, so you can test your program as you go along.
Program Requirements
A party planner needs to do calculations for an event, based on the number of guests and the size of tables. Class files are PartyPlanner.java and Party.java
Documentation: Only the top of file descriptions an author tags are required for this program.
Test 1 (5 pts):
Within the PartyPlanner class:
Write the code to read the number of guests and table capacity (i.e. number of people that each table will hold) from the user as integers.
After reading the first input, display a "Guest input valid integer" message ? After reading the second input, display a "Table input valid integer" message
Example:
Enter number of guests:
10
Guest input valid integer Enter capacity of one table:
5
Table input valid integer
Tests 2 through 4 (10 pts, 10 pts, 5 pts):
Within the PartyPlanner class:
Add an exception handler that includes:A try block around the code that reads the user inputs.
If no exception is thrown after reading the first input, display the same "Guest input valid integer" message as before
If no exception is thrown after reading the second input, display the same "Table input valid integer" message as before
A catch block to catch the InputMismatchException thrown if the user does not enter an integer for one of the inputs.
The catch block should display "Error - did not enter an integer value"
Test 5 (10 pts):
Within the Party class:
Add a constructor that has parameters for both data fields. ? Add getters for both data fields.
Test 6 (5 pts):
Within the Party class:
Add a calcFullTables() method that will divide numGuests by tableCapacity to determine and return an integer containing how many full tables would be needed for the event (NOTE: use integer division to ignore any remainder).
Test 7 (10 pts):
Within the PartyPlanner class:
Add code to the try block in the main method to:
Create an object with the user entered values as arguments to the constructor o Use the object created to call the calcFullTables() method.
Display the results, using the getter to access numGuests, in the format:
3 full table(s) needed for party of 30 guests
Test 8 (10 pts):
Within the PartyPlanner class:
Add a second catch block that will catch the ArithmeticException that may be thrown when the calcFullTables() method is called and the table capacity is 0 during the division.
The catch block should display "Error - cannot determine number of tables when table capacity is 0"
Test 9 through 12 (25 pts total):
Within the Party class:
Within the calcFullTables() method, before doing any calculations, add code to throw an IllegalArgumentException when either data field value is negative. o The throw should create a new IllegalArgumentException with the message "Error - cannot compute tables using negative value(s)"
Within the PartyPlanner class:
Add a third catch block that will catch the IllegalArgumentException that may be thrown when the calcFullTables() method is called.
The catch block should display the message from the IllegalArgumentException object
Warning: Do NOT display a quoted String here
display the message from the IllegalArgumentException object
Party.java template
public class Party {
private int numGuests;
private int tableCapacity;
// Insert your code here
}
PartyPlanner.java template
import java.util.InputMismatchException;
import java.util.Scanner;
public class PartyPlanner {
public static void main(String[] args) {
// Insert your code here
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
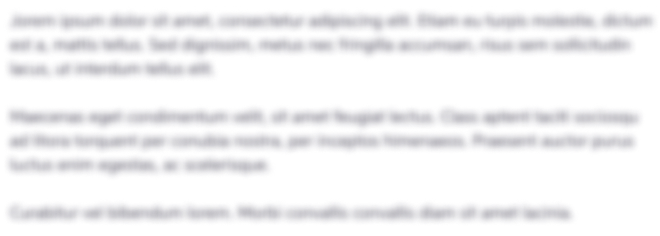
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started