Question
use the txt file in this java code. Booking.java: package booking; import java.util.*; import java.io.FileWriter; public class Booking { public static void main(String[] args) {
use the txt file in this java code.
Booking.java:
package booking;
import java.util.*;
import java.io.FileWriter;
public class Booking {
public static void main(String[] args) {
Scanner obj=new Scanner(System.in);
System.out.println("enter the name of the hotel ");
String hotelname=obj.nextLine();
Random random=new Random();
int countofrooms=random.nextInt(100);
while(countofrooms<=10)
{
countofrooms=random.nextInt(100);
}
//random type of room
int index=0;
//declaring a rooms array
Room rooms[]=new Room[countofrooms];
while(index
{
int type=random.nextInt(4);
while(type==0)
type=random.nextInt(4);
Room room=null;
switch(type)
{
case 1:room=new Room("king");
break;
case 2:room=new Room("queen");
break;
case 3:room=new Room("double");
break;
case 4: room = new Room("Cabanna");
break;
case 5: room = new Room(" ajoining");
}
rooms[index]=room;
index++;
}
//creating a hotel object
Hotel hotel=new Hotel(hotelname,rooms);
int input=-1;
do{
String rname,rtype;
switch(input)
{
case 1: System.out.println("Enter the name for a reservation.");
rname=obj.nextLine();//to bypass the consumption of new line
rname=obj.nextLine();
System.out.println("Enter the type of room to be reserved.");
rtype=obj.nextLine();
hotel.createReservation(rname, rtype);
break;
case 2:System.out.println("Enter the name by which the reservation is made.");
rname=obj.nextLine();//to bypass the consumption of new line
rname=obj.nextLine();
System.out.println("Enter the type of room reserved.");
rtype=obj.nextLine();
hotel.cancelReservation(rname, rtype);
break;
case 3:System.out.println("Enter a name for the invoice.");
rname=obj.nextLine();//to bypass
rname=obj.nextLine();
hotel.printInvoice(rname);
break;
case 4:System.out.println(hotel.toString());
break;
}
System.out.println("1.Make a reservation");
System.out.println("2.Cancel a reservation");
System.out.println("3.See an invoice");
System.out.println("4.See the hotel info");
System.out.println("5.Exit the booking system");
}while((input=obj.nextInt())!=5);
}
}
Hotel.java:
package booking; import java.util.NoSuchElementException;
class Hotel{
private String name;
private Room rooms[];
private Reservation reservation[];
private int index=-1;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Room[] getRooms() {
return rooms;
}
public void setRooms(Room[] rooms) {
this.rooms = rooms;
}
public Reservation[] getReservation() {
return reservation;
}
public void setReservation(Reservation[] reservation) {
this.reservation = reservation;
}
public Hotel(String name,Room rooms[])
{
this.rooms=rooms;
this.name=name;
reservation=new Reservation[rooms.length];
}
public void addReservation(Reservation reservation)
{
//add into the reservation array
this.reservation[++index]=reservation;
}
public void createReservation(String name,String type)
{
Room test=new Room();
Room room=test.findAvailableRoom(rooms, type);
if(room==null)
{
//print an error message
System.out.println("No such type of rooms are available at the moment.");
}
else{
room.setAvailability(false);
Reservation reserve=new Reservation(room,name);
addReservation(reserve);
}
}
public boolean removeReservation(String name,String type)
{
boolean flag=false;
for(int i=0;i<=index;i++)
{
if(reservation[i].getName().equals(name)&&reservation[i].getRoom().getType().equals(type))
{
flag=true;//found a reservation
reservation[i].getRoom().setAvailability(true);
//remove this reservation
int p=i;
for(int j=i+1;j
{
reservation[p]=reservation[j];
p++;
}
reservation[reservation.length-1]=null;
index--;
break;
}
}
try{
if(!flag)
throw new NoSuchElementException();
}
catch(Exception e)
{
System.out.println("No such reservation found !");
}
return flag;
}
public void cancelReservation(String name,String type)
{
boolean flag=removeReservation(name,type);
if(flag)
System.out.println("cancelled the reservation successfully.");
}
public void printInvoice(String name)
{
double bill=0;
for(int i=0;i<=index;i++)
{
if(reservation[i].getName().equals(name))
{
bill+=reservation[i].getRoom().getPrice();
}
}
if(bill==0)
System.out.println("No payable amount due.");
else
System.out.println("The user "+name+" has a payable amount of $"+bill+" ");
}
public String toString()
{
int queen=0;
int king=0;
int double_=0; int cabanna =0; int ajoining=0;
for(int i=0;i
{
if(rooms[i].getType().equals("king"))
king++;
else if(rooms[i].getType().equals("queen"))
queen++;
else
double_++;
}
return ("Hotel : "+name+" "+"Rooms: King:"+king+" queen:"+queen+" double:"+double_+" cabanna:"+cabanna+" ajoining: "+ajoining+" ");
}
}
reservation.java:
package booking;
class Reservation{
private String name;
private Room roomReserved;
public Reservation(Room room,String name)
{
this.name=name;
roomReserved=room;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Room getRoom() {
return roomReserved;
}
public void setRoom(Room roomReserved) {
this.roomReserved = roomReserved;
}
}
room.java:
package booking;
import java.util.NoSuchElementException;
import java.util.Random;
import java.util.Scanner;
class Room{
private String type;
private double price;
private boolean availability;
public Room()
{
//default constructor
}
public Room(String type)
{
this.type=type;
try{
if(type.equals("cabanna"))
{
price = 200;
}
if (type.equals("ajoining"))
{
price = 95;
}
if(type.equals("king"))
{
price=160;
}
else if(type.equals("queen"))
{
price=130;
}
else if(type.equals("double"))
{
price=97;
}
else
throw new IllegalArgumentException();
}
catch(Exception e)
{
System.out.println("The type of room specified is INVALID.");
}
availability=true;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public boolean getAvailability() {
return availability;
}
public void setAvailability(boolean availability) {
this.availability = availability;
}
//additional methods
public void changeAvailability()
{
this.availability=!availability;
}
public Room findAvailableRoom(Room rooms[],String type)
{
for(int i=0;i
{
if(rooms[i].getAvailability()&&rooms[i].getType().equals(type))
return rooms[i];
}
return null;
}
}
booking.txt:
1 Bob Mike king 160 1 Matt Benjamin King 160 2 Keller Helen Ajoining 95 3 Tim Mary Cabanna 200 4 James Alan Double 3 William Robin Cabbana 200 4 Jacob Tom double 97 2 Nathan Norman ajoining 95 5 martha helen queen 130
Step by Step Solution
There are 3 Steps involved in it
Step: 1
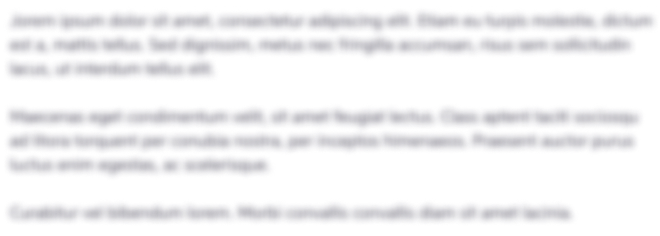
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started