Question
Use the variables that are given Assumptions 1. For now, the CokeMachine only dispenses Coke and no other types of items. 2. Payments are entered
Use the variables that are given
Assumptions
1. For now, the CokeMachine only dispenses Coke and no other types of items. 2. Payments are entered in pennies - $0.50 is entered as 50, $1.00 is entered as 100. 3. Any displays of change will be in dollars and cents. 4. Any payment entered cannot be used as part of the dispensed change. For example, if the user enter 1234 ($12.54) for payment, then the machine will only dispense a Coke if is has enough change for 1204. 5. When restocking the machine, the entire restock request has to fit. If the entire quantity does not fit, then the restock request is rejected. UML Use the following UML to create the outline of your CokeMachine class. Please note that exact names (spellings and case) should be used.
Create a menu in your .cpp file to use your Coke Machine. 0. Walk away 1. Buy a Coke 2. Restock Machine 3. Add change 4. Display Machine Info When you instantiate your CokeMachine object, your constructor will assign Your machine's name The price of a single Coke The amount of change in the machine The number of items (inventory level) in the machine The constructor I used (that you will see in the example output) is CokeMachine MyCokeMachine{"CSE 1325 Coke Machine", 50, 500, 100}; The max capacities of the machine (inventory and change) are set in the initalizers of the data members. These values are given in the UML. Any printing to the screen should take place in the .cpp file. Your CokeMachine class should not have any cins or couts in it. The UML is set up such that all functions return the information needed to print messages to the screen. Additional Information about the using of action in buyACoke() buyACoke() returns true/false to indicate whether or not the function worked. buyACoke() also sets action to different values depending on what happened while executing the code in buyACoke(). For example, if a Coke costs $0.50 and a payment of 30 cents is entered, then buyACoke() will fail but your .cpp program needs to know why so it can print a message to inform the user what went wrong your .cpp program needs to know what action to take. buyACoke() would assign a specific value to action that would relay back to your .cpp program what happened so that it can print a message about it. For example, buyACoke() if payment is insufficient, then action would be set to 3 (for example) .cpp program get action back from buyACoke() and print a message about insufficient funds because action is 3 You will need to define multiple values for action to mean the various actions that your .cpp program needs to take based on what happened when buyACoke() was called. I used an enumerated type so that I could use words instead of numbers to make my code easier to read. enum ACTION {OK, NOINVENTORY, NOCHANGE, INSUFFICIENTFUNDS, EXACTCHANGE};
The code needs to follow the rubric below.
UML-1 | Variable names and function names from CokeMachine UML match variables and functions in CokeMachine.h |
---|---|
UML-2 | Private data members from the UML are in the private section of CokeMachine.h. |
UML-3 | Public member functions from the UML are in the public section of CokeMachine.h |
UML - 4 | Member function parameters match UML in both name and type and member function return values must match in type. |
UML-5 | Initializations from UML are also in CokeMachine.h |
Constructor-1 | CokeMachine.h contains a constructor as defined by the UML. |
Constructor-2 | The constructor call in Code2.cpp sets The machine's name The price of a single Coke The amount of change in the machine The number of items (inventory level) in the machine |
Code2.cpp-1 | Displays the correct menu and uses a while loop to run different menu options. |
Code2.cpp-2 | "Buy a Coke" menu option asks for payment. Payment is entered in pennies. Member function buyACoke() is called with payment. |
Code2.cpp-3 | If buyACoke() returns TRUE, then use the value of action to either print "Here's your Coke and your change of $x.xx" or print "Thank you for the exact change. Here's your Coke". buyACoke() returns the values for action and change. Change should be a string of $x.xx. |
Code2.cpp-4 | If buyACoke() returns FALSE, then, based on the value of action, print a message about either insufficient funds or unable to give change or out of Coke or, if action is not recognized, print a message that the Coke machine is out of order. |
Code2-cpp-5 | "Restock Machine" menu option asks how much inventory to add to the machine. Member function incrementInventory() is called. If TRUE is returned, then print a message stating machine has been restocked. If FALSE is returned, then print a message about exceeding machine's capacity. In either case, call member function getInventoryLevel() to print the current inventory level. |
Code2.cpp-6 | "Add change" menu option asks how much change to add to the machine. Member function incrementChangeLevel() is called. If TRUE is returned, then print a message stating the change has been updated. If FALSE is returned, then print a message about exceeding machine's capacity. In either case, call member function getChangeLevel() to print the current change level. |
Code2.cpp-7 | "Display Machine Info" menu option calls member functions getInventoryLevel(), getMaxInventoryCapacity(), getChangeLevel(), getMaxChangeCapacity() and getCokePrice() and displays information to screen. |
Code2.cpp-8 | Menu has an option to exit the menu gracefully. Code2.cpp only displays the menu, accepts input, call the appropriate menu functions and print messages based on values returned by the member functions. |
Code2.cpp-9 | Code2.cpp should only display the menu, take user input, call member functions and display output based on information returned by member functions. |
CokeMachine.h-1 | No couts or cins in CokeMachine.h |
CokeMachine.h-2 | Member function incrementChangeLevel() verifies that taking a payment will not exceed the change max capacity. |
CokeMachine.h-3 | Member function incrementInventory() verifies that restocking request will not exceed the machine's inventory max capacity. |
CokeMachine.h-4 | Member function displayMoney() properly converts pennies to dollars and cents into a string. displayMoney() is only called by member functions and is NEVER called from Code2.cpp. All member functions that return change as a string must call displayMoney() to convert int change to string change. string containing dollars and cents should also contain the $ and decimal point so that money is displayed at $x.xx. |
makefile | makefile matches the template with only the 1st line being modified for student name and id. |
Functionality-1 | If buying a Coke is successful, then it should decrement inventory by 1 and add the value of CokePrice to change level |
Functionality-2 | If adding change is successful, then the change level should increase by the amount of change added |
Functionality-3 | If adding inventory is successful, then the inventory level should increase by the amount of inventory added. |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
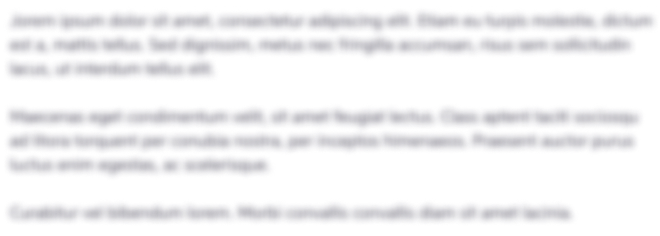
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started