Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using a dynamic array, implement a polynomial class with polynomial addition, subtraction, and multiplication. The partial solutions (Polynomial.h and Polynomial_partial.cpp) are posted on Canvas in
Using a dynamic array, implement a polynomial class with polynomial addition, subtraction, and multiplication. The partial solutions (Polynomial.h and Polynomial_partial.cpp) are posted on Canvas in Files->Lab 9 files. Please extend the partial solution to complete the following operations: ?polynomial + polynomial ?constant + polynomial ?polynomial + constant ?polynomial polynomial ?constant polynomial ?polynomial constant ?polynomial * polynomial ?constant * polynomial ?polynomial * constant Supply functions to assign and extract coefficients, indexed by the exponents (degrees). Supply a function to evaluate the polynomial given a value of type double. f = 2*x^3 + 5*x^2 - 3*x g = -10*x^2 + 7 f + g = 2x^3 - 5x^2 - 3x + 7 f - g = 2x^3 +15x^2 - 3x - 7 f * g = 2x^3 5x^2 -3x -10x^2 7 ------------------------------- 14x^3 35x^2 -21x -20x^5 -50x^4 30x^3 ----------------------------------------------------------- -20x^5 -50x^4 44x^3 35x^2 -21x Polynomial-partial.cpp code: #include #include #include \"polynomial.h\" using namespace std; Polynomial::Polynomial() { degree = 10; coeff = new double[degree+1]; for (int i = 0; i { coeff[i] = 0; } } Polynomial::Polynomial(int degr) { degree = degr; coeff = new double[degree+1]; for (int i = 0; i { coeff[i] = 0; } } Polynomial::Polynomial(const Polynomial &poly) { degree = poly.get_degree(); coeff = new double[degree+1]; for (int i = 0; i { coeff[i] = poly.get_coeff(i); } } Polynomial::Polynomial(double cf[], int deg) { degree = deg; coeff = new double[degree+1]; for (int i = 0; i { coeff[i] = cf[i]; } } Polynomial::~Polynomial() { delete [] coeff; }int Polynomial::get_degree() const { return degree; } double Polynomial::get_coeff(int deg) const { if (degree { return 0; // The input degree is larger than the polynomial degree } return coeff[deg]; } void Polynomial::set_coeff(int degr, double val) { if (degree { cout return; } coeff[degr] = val; } // Evaluate the polynomial double Polynomial::evaluate(double val) { ... } // Assignment operator void Polynomial::operator =(const Polynomial &poly) { if (this == &poly) { // Copy to itself. Nothing to be done. return; } ... } // Overloaded operator + Polynomial operator+(const Polynomial &pola, const Polynomial &polb) { ... } // Overloaded ostream& operator { ... } Polynomial.h code: #ifndef POLYNOMIAL_H_ #define POLYNOMIAL_H_ #include using namespace std; // Class to implement polynomial operations class Polynomial { public: Polynomial(); Polynomial(int degr); // Initialize the object with degree of degr Polynomial(const Polynomial &poly); // Copy constructor Polynomial(double cf[], int degr); // Parameterized constructor. The input is an array of double values and // the degree. The array of double should have already been populated with // the coefficients of the polynomial. Polynomial(double ct); // A constructor to automatically convert a constant to a polynomial ~Polynomial(); int get_degree() const; // Return the private member degree. double get_coeff(int index) const; // Return the coefficient of a index. void set_coeff(int index, double val); // Mutator to alter a coefficient void operator = (const Polynomial &poly); // Assignment operator friend Polynomial operator+(const Polynomial &polya, const Polynomial &polyb); friend Polynomial operator-(const Polynomial &polya, const Polynomial &polyb); friend Polynomial operator*(const Polynomial &polya, const Polynomial &polyb); friend ostream& operator double evaluate(double val); // Evaluate the polynomial private: double *coeff; int degree; }; #endif /* POLYNOMIAL_H_ */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
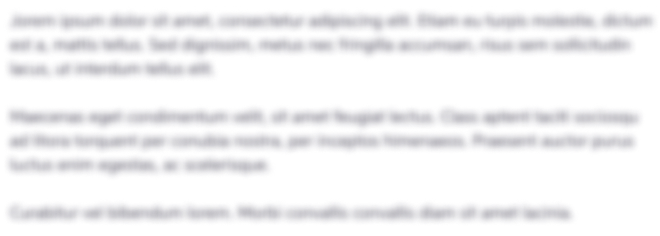
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started