Question
Using both the List, Stack, and Queue from the given below, write the main in Java: //Input: ( ( 1 + 2 ) - (
Using both the List, Stack, and Queue from the given below, write the main in Java:
//Input:
( ( 1 + 2 ) - ( ( 3 - 4 ) + ( 7 - 2 ) ) ) ( ( 1 + 2 ) - ( 3 - 4 ) ) ( ( ( 1 + 2 ) - 3 ) - 4 ) ( ( 1 + ( 2 - 3 ) - 4 ) ) ( 1 + ( 2 - ( 3 - 4 ) ) )
//Output:
Expression is: ( ( 1 + 2 ) - ( ( 3 - 4 ) + ( 7 - 2 ) ) ) Postfix is 1 2 + 3 4 - 7 2 - + - Value is -1.0 Expression is: ( ( 1 + 2 ) - ( 3 - 4 ) ) Postfix is 1 2 + 3 4 - - Value is 4.0 Expression is: ( ( ( 1 + 2 ) - 3 ) - 4 ) Postfix is 1 2 + 3 - 4 - Value is -4.0 Expression is: ( ( 1 + ( 2 - 3 ) - 4 ) ) Postfix is 1 2 3 - 4 - + Value is -4.0 Expression is: ( 1 + ( 2 - ( 3 - 4 ) ) ) Postfix is 1 2 3 4 - - + Value is 4.0
The requirements are as follows:
The input will be in a text file whose name is given by arg[0] of main().
It will contain a fully parenthesized infix expression containing only: "(", ")", "+", "-" and integers.
Must use arg[0] as the name of the input file
Use these class to write the main.
// List.java
import java.util.ArrayList; import java.util.Collections;
public class List { /** * Create an empty List */ public List() { mFront = new Node(Node.DUMMY); mRear = new Node(Node.DUMMY); mFront.mNext = mRear; mRear.mPrev = mFront; } /** * Create a new List that is a copy of the list input * @param list the List to copy */ public List(List list) { this(); for(Node current = list.mFront.mNext; current != list.mRear; current = current.mNext) { addToRear(current.mData); } } /** * Item becomes the new front element * @param item the item to add */ public void addToFront(String item) { Node newNode = new Node(item); newNode.mPrev = mFront; newNode.mNext = mFront.mNext; newNode.mPrev.mNext = newNode; newNode.mNext.mPrev = newNode; } /** * Item becomes the new rear element * @param item the item to add */ public void addToRear(String item) { Node newNode = new Node(item); newNode.mNext = mRear; newNode.mPrev = mRear.mPrev; newNode.mPrev.mNext = newNode; newNode.mNext.mPrev = newNode; } /** * If beforeItem is not in List, prints "Item Not Found" else add item in List before beforeItem * @param beforeItem the item in the list to add item before * @param item the item to add */ public void addBeforeItem(String beforeItem, String item) { Node beforeNode = find(beforeItem); if(beforeNode == null) { sop("Item Not Found"); return; } Node newNode = new Node(item); newNode.mPrev = beforeNode.mPrev; newNode.mNext = beforeNode; newNode.mPrev.mNext = newNode; newNode.mNext.mPrev = newNode; } /** * Item becomes the element after afterItem * @param afterItem the item in the list to add item before * @param item the item to add */ public void addAfterItem(String afterItem, String item) { Node afterNode = find(afterItem); if(afterNode == null) { sop("Item Not Found"); return; } Node newNode = new Node(item); newNode.mPrev = afterNode; newNode.mNext = afterNode.mNext; newNode.mNext.mPrev = newNode; newNode.mPrev.mNext = newNode; } /** * Returns the item at the front of the List (List is not altered) * @return the item at the front of the List */ public String getFront() { if(askCount() == 0) { sop("List Empty"); return ""; } return(mFront.mNext.mData); } /** * Returns the item at the rear of the List (List is not altered) * @return the item at the rear of the List */ public String getRear() { if(askCount() == 0) { sop("List Empty"); return ""; } return(mRear.mPrev.mData); } /** * Return true if item is in List, false otherwise * @param item to check presence in List * @return true if item is in List, false otherwise */ public boolean isPresent(String item) { if(find(item) != null) return true; else return false; } /** * Returns the number of items in the List * @return the number of items in the List */ public int askCount() { int count = 0; Node current = mFront.mNext; while(current != mRear) { current = current.mNext; ++count; } return count; } /** * If the List is empty, prints "List Empty" * If the List is not empty, removes the item at the front of the List */ public void removeFront() { if(askCount() == 0) { sop("List Empty"); return; } removeItem(mFront.mNext.mData); } /** * If the List is empty, prints "List Empty" * If the List is not empty, removes the item at the rear of the List */ public void removeRear() { if(askCount() == 0) { sop("List Empty"); return; } removeItem(mRear.mPrev.mData); } /** * If the List is empty, prints "List Empty" * If item is not present in List, prints "Item not found" * Otherwise, item is removed from the List * @param item the item to remove */ public void removeItem(String item) { if(askCount() == 0) { sop("List Empty"); return; } Node removeNode = find(item); if(removeNode == null) { sop("Item not found"); return; } removeNode.mPrev.mNext = removeNode.mNext; removeNode.mNext.mPrev = removeNode.mPrev; } /** * Print title on a line by itself * Prints the List from front to rear with 1 space between each item * @param title the description of the List */ public void print(String title) { System.out.println(" " + title); for(Node current = mFront.mNext; current != mRear; current = current.mNext) { System.out.print(current.mData + " "); } System.out.println(""); } /** * Print title on a line by itself * Prints the Sorted List with 1 space between each item * Does not alter the List * @param title the description of the List */ public void printSorted(String title) { ArrayList tempList = new ArrayList(); for(Node current = mFront.mNext; current != mRear; current = current.mNext) { tempList.add(current.mData); } Collections.sort(tempList); System.out.println(" " + title); for(String s : tempList) { System.out.print(s + " "); } System.out.println(""); } private class Node { public Node(String data) { mData = data; mPrev = null; mNext = null; } private static final String DUMMY= "dummy"; private String mData; private Node mPrev; private Node mNext; } private Node find(String item) { for(Node current = mFront.mNext; current != mRear; current = current.mNext) { if(current.mData.equals(item)) return current; } return null; } private static void sop(String s) { System.out.println(s); } private Node mFront; private Node mRear; }
//Stack.java
public class Stack { private List mStack; /** * Create an empty Stack * @param myList Create an empty Stack */ public Stack() { mStack = new List(); } /** Make item the Top of the Stack * * @param item Make item the Top of the Stack */ public void push(String item) { mStack.addToRear(item); } /** * Remove the Top of the Stack * @param i */ public void pop() { mStack.removeRear(); } /** * * @return the Top of the Stack do not remove it */ public String getTop() { return mStack.getRear(); } /** * * @return true if Stack is empty, false otherwise */ public boolean isEmpty() { return mStack.askCount() == 0; } }
//Queue.java
public class Queue { private List mQueue; public Queue() { mQueue= new List(); } public void add(String item) { mQueue.addToRear(item); } /** * Get the first item in the list and removes it * @return the front of the Queue */ public String remove() { String item = mQueue.getFront(); mQueue.removeFront(); return item;
} public boolean isEmpty() { return mQueue.askCount() == 0; } public void dump(String title) { mQueue.print(title); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
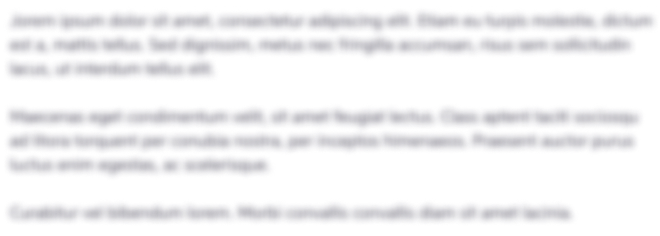
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started