Question
***USING C CODING LANGUAGE AND BUILDING OFF FOLLOWING SOURCE CODE: #include #include #include #include struct _data { // Define the structure (nothing is global here).
***USING C CODING LANGUAGE AND BUILDING OFF FOLLOWING SOURCE CODE:
#include
#include
#include
#include
struct _data { // Define the structure (nothing is global here).
char *name;
long number;
};
/*****************************************************/
/* See how big the file is. */
/*****************************************************/
int SCAN(FILE *(*stream)) { // We want to pass stream by reference (a pointer to a pointer).
int size = 0;
size_t chrCount;
char *text;
// Open the file and make sure it was opened.
if ((*stream = fopen("./hw5.data", "r")) == NULL) {
printf("ERROR - Could not open hw5.data file. ");
exit(0);
}
// Use getline to read in a full line of data/text.
// This way, we don't need to guess how big each name is.
while (1) {
text = NULL; // MUST be set to NULL!!!!
getline(&text, &chrCount, *stream); // getline expects 3 pointer arguments.
free(text);
if (feof(*stream)) break;
size++;
}
return size;
}
/*****************************************************/
/* Load the file. */
/*****************************************************/
struct _data *LOAD(FILE *stream, int size) { // Here we can just pass stream by value (we're not changing it).
int i;
size_t chrCount;
char *text, *phone, *name;
struct _data *BlackBox;
// Allocate memory for array of struct.
if ((BlackBox = (struct _data*)calloc(size, sizeof(struct _data))) == NULL) {
printf("ERROR - Could not allocate memory. ");
exit(0);
}
// Rewind the file.
rewind(stream);
// Use getline to read in ALL of the data/text.
for (i = 0; i < size; i++) {
text = NULL; // MUST be set to NULL!!!!
getline(&text, &chrCount, stream);
name = strtok(text, " "); // strtok extracts all characters to " ".
phone = strtok(NULL, " "); // strtok extracts all characters to " ".
// Allocate memory for name part of struct.
if ((BlackBox[i].name = (char*)calloc(strlen(name)+1, sizeof(char))) == NULL) {
printf("ERROR - Could not allocate memory. ");
exit(0);
}
strcpy(BlackBox[i].name, name); // copy name into struct.
BlackBox[i].number = atol(phone); // we want number as an long integer.
free(text);
}
fclose(stream);
return BlackBox; // Return pointer to dynamic array.
}
/*****************************************************/
/* Search for name. */
/*****************************************************/
void SEARCH(struct _data *BlackBox, char *name, int size) {
int i;
int found = 0;
printf("******************************************* ");
for (i = 0; i < size; i++) {
if (!strcmp(name, BlackBox[i].name)) {
printf("The name was found at the %d entry. ", i);
found = 1;
break;
}
}
if (found == 0) {
printf("The name was NOT found. ");
}
printf("******************************************* ");
}
/*****************************************************/
/* Free memory. */
/*****************************************************/
void FREE(struct _data *BlackBox, int size) {
int i;
for (i = 0; i < size; i++) {
free(BlackBox[i].name);
}
free(BlackBox);
BlackBox = NULL;
}
/*****************************************************/
/* Main */
/*****************************************************/
int main(int argv, char **argc) {
int size;
FILE *stream;
struct _data *BlackBox;
if (argv == 1) {
printf("******************************************* ");
printf("* You must include a name to search for. * ");
printf("******************************************* ");
}
if (argv == 2) {
size = SCAN(&stream);
BlackBox = LOAD(stream, size);
SEARCH(BlackBox, argc[1], size);
FREE(BlackBox, size);
}
return 0;
}
***
The program will require the following structure:
struct _data {
char *first, *middle, *last
long number;
};
The program will require command line arguments:
int main(int argv, char **argc) {
Where argv is the number of arguments and argc is an array
holding the arguments (each is a string). Your program must catch
any case where no command line arguement was provided and print
a warning message (see below).
You MUST include/use the following functions, defined as follows:
int SCAN(FILE *(*stream)) - this function will open the file/stream
and return an integer indicating how many lines there are. Note that
I need to pass stream, which is a pointer, by reference. So I am
passing this as a pointer to a pointer.
struct _data *LOAD(FILE *stream, int size) - this function will
rewind file, create the dynamic array (of size), and read in the
data, populating the _data struct dynamic array. Note that stream
is passed by value this time. The function then returns the populated
array of struct.
void SEARCH(struct _data *BlackBox, char *name, int size) - this function
will get the dynamic array of struct passed to it, the name we are looking
for, and the size of the array. This function will then search the dynamic
array for the name. See below for examples.
void FREE(struct _data *BlackBox, int size) - this function will free up
all of the dynamic memory we allocated. Take note of the number of times
malloc/calloc were called, as you need to free that same number.
Finally, the data file will be called hw6.data and will be formated as:
ron jonny johnson 7774013
jon ronny johnsen 7774014
tom jones smith 7774015
won ton lee 7774016
You code MUST be able to handle cases where the names are VERY long.
HINTS:
------
Functions that will make things much easier:
getline()
feof()
strtok()
atoi()
SAMPLE RUNS:
------------
Case 1 - No command line argument provided.
[ron@chef junk]$ ./CS230-5
*******************************************
* You must include a name to search for. *
*******************************************
Case 2 - Provided name is NOT in the list.
[ron@chef junk]$ ./CS230-5 joe
*******************************************
The name was NOT found.
*******************************************
Case 3 - Search by FIRST name (name is in the list).
[ron@chef junk]$ ./CS230-5 tom
*******************************************
The name was found at the 2 entry.
*******************************************
Case 4 - Search by FIRST and LAST name (name is in the list, note the "_"
wildcard).
[ron@chef junk]$ ./CS230-5 tom _ smith
*******************************************
The name was found at the 2 entry.
*******************************************
Case 4 - Search by FIRST, MIDDLE, and LAST name (name is in the list).
[ron@chef junk]$ ./CS230-5 tom jones smith
*******************************************
The name was found at the 2 entry.
*******************************************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
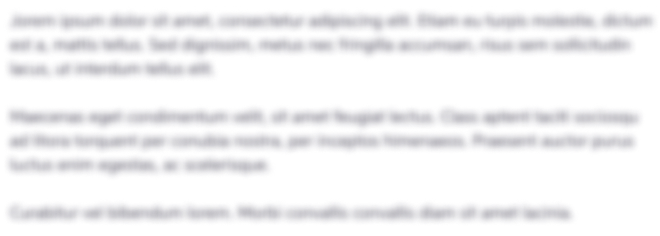
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started